Exploring Angular Modules: Organizing Your Application
Angular is a powerful framework for building dynamic and robust web applications. As projects grow in complexity, organizing the code becomes vital to maintainability and scalability. Angular modules offer a solution to tackle this challenge by providing a way to divide and conquer the application into smaller, manageable pieces. In this blog post, we’ll delve into the world of Angular modules and explore how they can help you organize your application efficiently.
Table of Contents
1. Understanding Angular Modules
Angular modules are the building blocks of an Angular application. They group related components, services, directives, and other pieces of code together to form cohesive units. A module encapsulates the functionality of a specific feature or section of your application, making it easier to understand, maintain, and reuse code.
2. Creating an Angular Module
To create a new module in an Angular application, you can use the Angular CLI or manually create the necessary files. Let’s start by using the Angular CLI to generate a new module called “ProductModule.”
bash ng generate module product
This command will generate a new folder named “product” under the “app” directory, containing the necessary files for the module.
3. Module Structure
An Angular module consists of four key parts:
- Imports: Here, you can include other modules that your module depends on. These imported modules expose their functionality to the current module, allowing you to use components, services, and directives from those modules within your own.
- Exports: This section determines what elements of the module are accessible to other modules that import it. By exporting specific components, services, or directives, you make them available for use in other parts of your application.
- Declarations: Here, you list the components, directives, and pipes that belong to the module. These elements are available for use within the module itself.
- Providers: This section is where you specify the services that the module provides. Services are singletons in Angular and are shared across the entire application.
4. Feature Modules vs. Shared Modules
Angular offers two main types of modules: feature modules and shared modules.
- Feature Modules: These modules are used to organize the application into distinct features. Each feature module typically represents a specific section of the application, such as a user profile, shopping cart, or dashboard. Feature modules help keep the codebase clean, making it easier to manage and scale.
- Shared Modules: Shared modules, as the name suggests, contain elements that are shared across multiple parts of the application. For example, if you have several modules that require the same custom directives or pipes, you can create a shared module to encapsulate and export those elements. This prevents code duplication and ensures consistent behavior throughout the application.
5. Lazy Loading
Lazy loading is a technique used to optimize the initial loading time of your application by loading modules on-demand rather than all at once. This is particularly useful for large applications where loading all modules upfront could lead to slow loading times.
To implement lazy loading, you need to set up your route configurations appropriately. When a user navigates to a route associated with a lazily loaded module, Angular loads the module on-demand. This way, the initial bundle size is reduced, and the application loads faster.
6. SharedModule for Reusable Elements
As mentioned earlier, SharedModule is a great way to encapsulate and share components, directives, and pipes that are used across multiple modules. However, there’s a caveat to be aware of: SharedModule should not provide services. If a service is provided in SharedModule, it will create a new instance of the service for each module that imports SharedModule, which may lead to unexpected behavior.
To overcome this issue, consider creating a separate CoreModule to provide application-wide services. The CoreModule should be imported once in the AppModule, ensuring that all services are singletons across the entire application.
7. Using NgModule Decorator
The @NgModule decorator is essential when defining an Angular module. It tells Angular how to compile and run module code. Let’s take a closer look at some common options provided by the @NgModule decorator:
- imports: An array of other modules that this module depends on.
- exports: An array of components, directives, or pipes that are made available to other modules.
- declarations: An array of components, directives, or pipes that belong to this module.
- providers: An array of services that the module provides.
- bootstrap: Specifies the component that should be bootstrapped when the module is loaded (used in AppModule only).
8. Best Practices for Organizing Modules
To ensure a well-organized Angular application, here are some best practices for working with modules:
- Feature-based organization: Organize your modules based on features to maintain a clear separation of concerns. This approach will make your codebase easier to navigate and understand.
- Lazy loading for performance: Use lazy loading for feature modules that are not immediately required when the application loads. This way, you can significantly improve the initial loading time of your application.
- SharedModule for reusable elements: Leverage SharedModule to encapsulate and share components, directives, and pipes that are used across multiple modules. Avoid providing services in SharedModule to prevent service duplication.
- CoreModule for application-wide services: Create a CoreModule to provide application-wide services and import it once in the AppModule to ensure singleton instances.
- Avoid circular dependencies: Be mindful of module dependencies to avoid circular dependencies, which can lead to runtime errors.
- Keep modules lean and focused: Aim for lean modules with a clear focus on their responsibilities. Avoid adding unrelated elements to a module to keep the codebase organized and maintainable.
Conclusion:
Angular modules play a critical role in organizing and structuring your application. They provide a way to encapsulate functionality, promote reusability, and improve maintainability and scalability. By adhering to best practices and understanding how to use feature modules, shared modules, and lazy loading, you can create a well-organized Angular application that is easy to navigate, develop, and maintain. Embrace the power of Angular modules, and take your web development projects to new heights!
Table of Contents
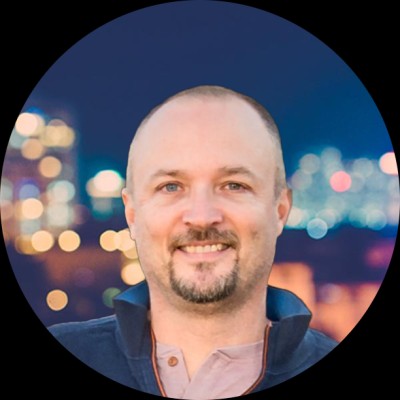
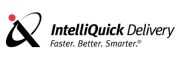