Exploring Angular Forms: Validation and Error Handling
Angular is a powerful front-end framework that simplifies the development of dynamic web applications. One of its essential features is its robust support for forms. In this blog post, we will dive deep into Angular forms, focusing on validation and error handling. Whether you are a seasoned Angular developer or just getting started, understanding how to work with forms effectively is crucial. By the end of this guide, you’ll have a solid grasp of Angular form validation and error handling techniques.
Table of Contents
1. Introduction to Angular Forms
1.1. Why Forms Matter in Web Applications
Forms are an integral part of most web applications. They enable users to input data, perform searches, submit feedback, and much more. Without forms, web applications would be limited in functionality. However, working with forms can be complex, especially when it comes to validation and error handling.
In a typical web application, you need to ensure that the data submitted via forms is valid and meets certain criteria. For example, you might want to make sure that an email address is in the correct format, a password meets security requirements, or a date of birth is within a specific range. This is where form validation comes into play.
1.2. Angular Forms: A Brief Overview
Angular provides two main approaches for working with forms: Template-Driven Forms and Reactive Forms. Let’s briefly explore both of them before diving into validation and error handling.
- Template-Driven Forms: This approach is template-centric. You create forms directly in your component’s HTML template. Template-Driven Forms are suitable for simple forms with minimal logic.
- Reactive Forms: Reactive Forms are code-centric. You define the form structure and validation rules in your component class. This approach is more flexible and suitable for complex forms.
In this blog post, we will explore both template-driven and reactive forms, along with their validation and error handling techniques.
2. Template-Driven Forms
2.1. Creating Template-Driven Forms
Template-Driven Forms are an excellent choice for simple forms where you want to quickly get up and running. Here’s a basic example of creating a template-driven form in Angular:
html <!-- app.component.html --> <form #myForm="ngForm" (ngSubmit)="onSubmit(myForm)"> <div class="form-group"> <label for="name">Name</label> <input type="text" id="name" name="name" ngModel required> </div> <div class="form-group"> <label for="email">Email</label> <input type="email" id="email" name="email" ngModel email required> </div> <button type="submit">Submit</button> </form>
In the code snippet above:
- We use the ngForm directive to create a form and bind it to the myForm template variable.
- Each input field is associated with a model using ngModel, which allows two-way data binding.
- We use the required and email validators to ensure the name and email fields are filled and contain valid email addresses.
2.2. Validating Template-Driven Forms
Validation in template-driven forms is primarily done using directives like required, minlength, maxlength, and more. These directives enforce specific validation rules on form controls.
For example, to ensure that a password field has a minimum length of 8 characters, you can use the minlength directive like this:
html <input type="password" id="password" name="password" ngModel minlength="8" required>
2.3. Error Handling in Template-Driven Forms
When a form control doesn’t meet its validation criteria, Angular adds CSS classes to the control, indicating its validity state. You can use these classes to style the control or display error messages.
Here’s an example of how to display error messages for the name field:
html <div class="form-group"> <label for="name">Name</label> <input type="text" id="name" name="name" ngModel required #name="ngModel"> <div *ngIf="name.invalid && (name.dirty || name.touched)" class="error-message"> <div *ngIf="name.errors.required">Name is required.</div> </div> </div>
In this code:
- We use the ngModel template reference variable #name to access the name control’s properties.
- We conditionally display an error message when the name control is invalid, dirty, or touched and has the required error.
Now that we’ve covered the basics of template-driven forms, let’s move on to reactive forms.
3. Reactive Forms
3.1. Building Reactive Forms
Reactive Forms provide more control and flexibility compared to template-driven forms. To create a reactive form, you need to define the form structure and validation rules in your component class.
Here’s an example of how to create a simple reactive form:
typescript // app.component.ts import { Component } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; @Component({ selector: 'app-root', templateUrl: './app.component.html', }) export class AppComponent { myForm: FormGroup; constructor(private formBuilder: FormBuilder) { this.myForm = this.formBuilder.group({ name: ['', [Validators.required]], email: ['', [Validators.required, Validators.email]], }); } onSubmit() { // Handle form submission } }
In this code:
- We import the necessary modules from @angular/forms.
- We use the FormBuilder to create a FormGroup called myForm.
- Each form control is defined with an initial value (empty string) and an array of validators.
3.2. Validating Reactive Forms
Validation in reactive forms is similar to template-driven forms but defined in the component class. Validators such as Validators.required and Validators.email are used to enforce validation rules.
To check the validity of a control in the component class, you can use the following code:
typescript const emailControl = this.myForm.get('email'); if (emailControl.hasError('required')) { // Handle the required error }
3.3. Error Handling in Reactive Forms
Error handling in reactive forms involves displaying error messages in the template based on the form control’s validity state.
Here’s an example of how to display error messages for the email field:
html <div class="form-group"> <label for="email">Email</label> <input type="email" id="email" formControlName="email"> <div *ngIf="myForm.get('email').invalid && myForm.get('email').touched" class="error-message"> <div *ngIf="myForm.get('email').hasError('required')">Email is required.</div> <div *ngIf="myForm.get('email').hasError('email')">Invalid email format.</div> </div> </div>
In this code:
- We use the formControlName directive to bind the email input to the email control.
- We conditionally display error messages based on the control’s validity state and specific errors.
4. Custom Validators
While Angular provides a set of built-in validators, you may encounter scenarios where you need to create custom validation rules for your forms. Angular allows you to create custom validators easily.
4.1. Creating Custom Validators
To create a custom validator, you need to define a function that returns a validator function or an object. The validator function should take a form control as an argument and return null if the control is valid or an error object if it’s not.
Here’s an example of a custom validator that checks if a password contains at least one uppercase letter:
typescript function uppercaseValidator(control: AbstractControl): ValidationErrors | null { const value: string = control.value || ''; if (/[A-Z]/.test(value)) { return null; // Valid } return { uppercase: true }; // Invalid }
4.2. Integrating Custom Validators into Forms
Once you’ve created a custom validator, you can use it in your form controls like any other validator. Here’s an example of how to use the uppercaseValidator in a reactive form:
typescript this.myForm = this.formBuilder.group({ password: ['', [Validators.required, uppercaseValidator]], });
In this code, we include uppercaseValidator in the array of validators for the password control.
Conclusion
In this blog post, we’ve explored Angular forms, with a focus on validation and error handling. We covered both template-driven and reactive forms, demonstrating how to create forms, validate user input, and handle errors effectively. Additionally, we discussed the creation and integration of custom validators to suit your specific form validation needs.
Mastering Angular forms is essential for building robust web applications that provide a seamless user experience. Whether you choose template-driven forms for simplicity or reactive forms for flexibility, you now have the knowledge and tools to implement form validation and error handling in your Angular projects effectively. Happy coding!
Table of Contents
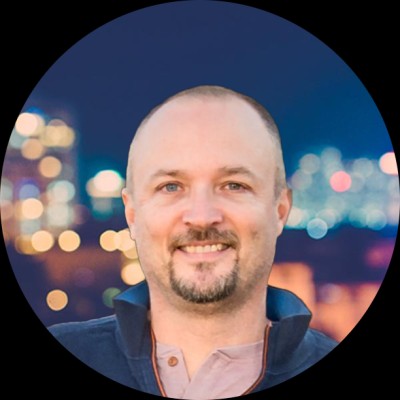
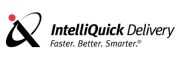