Mastering Input Validation in Angular: A Comprehensive Guide to Template-Driven and Reactive Forms
Angular is an incredibly powerful framework that simplifies the development of dynamic web applications. A favorite among companies looking to hire Angular developers, one of its outstanding features is its robust form handling capabilities. Angular provides two distinct approaches to forms – Template-Driven and Reactive Forms – both of which offer dynamic capabilities for controlling and validating input.
In this blog post, we will explore the nuances of form handling in Angular, focusing on input validation using both approaches. With this knowledge, not only will you be equipped to harness the full power of Angular forms but you’ll also understand why many organizations choose to hire Angular developers to optimize their web application development.
Understanding Angular Forms
Before delving into validation, it’s important to understand Angular’s two approaches to handling forms.
- Template-Driven Forms: These are simple to use and suit scenarios with simple logic. They use a “directive” based approach where the logic is driven from the template side of the application.
- Reactive Forms: These are more robust, offering more flexibility and scalability – ideal for complex scenarios. They are driven by the model, and hence they’re in sync with the application’s state.
Input Validation in Template-Driven Forms
Input validation is critical to any form. It helps ensure that the user provides all required data in the correct format. Angular’s template-driven forms handle validation using directives, which can be easily added to the HTML elements in the form.
Let’s take an example:
```html <form #form="ngForm" (ngSubmit)="onSubmit(form)"> <div> <label for="name">Name:</label> <input id="name" name="name" [(ngModel)]="model.name" required /> <div *ngIf="name.invalid && (name.dirty || name.touched)" class="error"> Name is required. </div> </div> </form> ```
In the code above, the `required` directive adds the validation rule that makes the name field mandatory. The `*ngIf` statement displays an error message if the field is invalid and has been either touched or modified (`name.dirty || name.touched`).
Input Validation in Reactive Forms
Reactive forms, on the other hand, define form controls and validators in the component class. They provide a clear and definite structure, making it easier to manage complex validation rules.
Here’s an example of a simple reactive form with validation:
```typescript import { Component } from '@angular/core'; import { FormGroup, Validators, FormBuilder } from '@angular/forms'; @Component({ selector: 'app-root', templateUrl: './app.component.html' }) export class AppComponent { form: FormGroup; constructor(private fb: FormBuilder) { this.form = this.fb.group({ name: ['', Validators.required] }); } onSubmit() { if (this.form.valid) { // Handle data } } } ```
In the component’s HTML:
```html <form [formGroup]="form" (ngSubmit)="onSubmit()"> <div> <label for="name">Name:</label> <input id="name" formControlName="name" /> <div *ngIf="form.get('name').invalid && (form.get('name').dirty || form.get('name').touched)" class="error"> Name is required. </div> </div> <button [disabled]="!form.valid">Submit</button> </form> ```
In the code above, we’ve created a new form group with a single control called ‘name’, which is required (`Validators.required`). The `*ngIf` statement in the HTML checks for the same conditions as before, but this time, we’re working with a form control in the reactive form group.
Implementing Custom Validators
Sometimes, the built-in validators such as `required`, `minlength`, and `maxlength` are not enough. In such scenarios, Angular allows us to create custom validators.
Let’s create a custom validator to check if the entered name starts with a capital letter.
```typescript import { AbstractControl, ValidationErrors, ValidatorFn } from '@angular/forms'; export function startsWithCapital(): ValidatorFn { return (control: AbstractControl): ValidationErrors | null => { const value = control.value; if (value && value[0] !== value[0].toUpperCase()) { return { 'startsWithCapital': true }; } return null; }; } ```
Here, the `startsWithCapital()` function returns a validator function that checks if the value of the form control starts with a capital letter. This type of function creation is a clear example of the skills and capabilities Angular developers bring to the table. If the function determines that the value does not start with a capital letter, it returns an error object; otherwise, it returns null, indicating no error. It’s this sort of functionality that leads many companies to hire Angular developers for their front-end needs.
This custom validator can be added to our reactive form like so:
```typescript this.form = this.fb.group({ name: ['', [Validators.required, startsWithCapital()]] }); ```
Now, the name field is required and must start with a capital letter.
Handling Asynchronous Validation
Angular also provides the ability to handle asynchronous validation, which is handy when we need to validate data against a server, for instance, checking the availability of a username.
```typescript import { AbstractControl, ValidationErrors, AsyncValidatorFn } from '@angular/forms'; import { Observable } from 'rxjs'; export function uniqueNameValidator(): AsyncValidatorFn { return (control: AbstractControl): Observable<ValidationErrors | null> => { return uniqueNameService.isNameTaken(control.value).pipe( map(isTaken => (isTaken ? { uniqueName: true } : null)), catchError(() => of(null)) ); }; } ```
In the example above, `uniqueNameValidator()` is an asynchronous validator function that checks whether a given name is unique by calling a hypothetical service `uniqueNameService`.
Conclusion
Angular provides a robust suite of features for form handling and validation. It allows developers, including those you might hire as Angular developers, to easily ensure that input data is correct and formatted properly, making data handling a breeze.
By understanding and utilizing both template-driven and reactive forms, as well as built-in and custom validators, you can harness the full power of Angular forms. This makes your Angular applications more secure, reliable, and user-friendly, showing why hiring Angular developers can be a beneficial decision. Whether you’re working with simple or complex forms, Angular and its proficient developers have the tools to make input validation easy and efficient.
Table of Contents
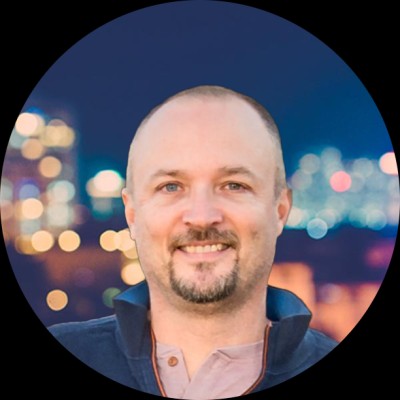
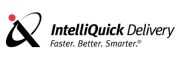