Exploring Angular Interceptors: Centralized HTTP Request Handling
In the world of web development, efficiency and security are paramount. Handling HTTP requests in a streamlined and secure manner is essential for building robust web applications. Angular, one of the most popular frontend frameworks, provides a powerful tool called interceptors that can help you achieve just that.
Table of Contents
In this comprehensive guide, we will delve into Angular interceptors, exploring what they are, how they work, and why they are a vital part of modern web development. We will also provide practical examples and use cases to demonstrate how you can harness the power of interceptors to centralize HTTP request handling in your Angular applications.
1. What Are Angular Interceptors?
Angular interceptors are a mechanism for intercepting HTTP requests and responses globally within an Angular application. They allow you to add middleware logic that can modify requests or responses before they are sent to or received from the server. This centralized approach to request handling provides several benefits:
1.1. Authentication and Authorization
Interceptors can be used to add authentication tokens or headers to every outgoing request. This ensures that only authenticated users can access protected resources on the server. Here’s a simple example of an interceptor that adds an authorization token to outgoing requests:
typescript import { Injectable } from '@angular/core'; import { HttpInterceptor, HttpEvent, HttpHandler, HttpRequest, } from '@angular/common/http'; import { Observable } from 'rxjs'; @Injectable() export class AuthInterceptor implements HttpInterceptor { intercept( req: HttpRequest<any>, next: HttpHandler ): Observable<HttpEvent<any>> { // Add authorization token to the request header const authToken = 'your-auth-token'; const authReq = req.clone({ setHeaders: { Authorization: `Bearer ${authToken}`, }, }); return next.handle(authReq); } }
1.2. Logging and Error Handling
You can use interceptors to log requests and responses, making it easier to debug issues in your application. Additionally, they allow you to handle errors globally, providing a consistent way to manage errors across your application.
typescript import { Injectable } from '@angular/core'; import { HttpInterceptor, HttpEvent, HttpHandler, HttpRequest, HttpResponse, HttpErrorResponse, } from '@angular/common/http'; import { Observable } from 'rxjs'; import { tap } from 'rxjs/operators'; @Injectable() export class LoggingInterceptor implements HttpInterceptor { intercept( req: HttpRequest<any>, next: HttpHandler ): Observable<HttpEvent<any>> { return next.handle(req).pipe( tap( (event) => { if (event instanceof HttpResponse) { // Log successful response console.log('Response:', event); } }, (error) => { if (error instanceof HttpErrorResponse) { // Log error response console.error('Error:', error); } } ) ); } }
1.3. Caching and Transformation
Interceptors can cache responses or transform data before it reaches your components. For example, you can cache frequently requested data to reduce server load or transform the response structure to match your application’s needs.
typescript import { Injectable } from '@angular/core'; import { HttpInterceptor, HttpEvent, HttpHandler, HttpRequest, HttpResponse, } from '@angular/common/http'; import { Observable, of } from 'rxjs'; @Injectable() export class CacheInterceptor implements HttpInterceptor { private cache = new Map<string, HttpResponse<any>>(); intercept( req: HttpRequest<any>, next: HttpHandler ): Observable<HttpEvent<any>> { if (req.method !== 'GET') { return next.handle(req); } // Check if the response is cached const cachedResponse = this.cache.get(req.url); if (cachedResponse) { return of(cachedResponse); } // If not cached, make the request and cache the response return next.handle(req).pipe( tap((event) => { if (event instanceof HttpResponse) { this.cache.set(req.url, event); } }) ); } }
Now that we understand the benefits of interceptors, let’s explore how to use them in an Angular application.
2. How to Use Angular Interceptors
To use interceptors in an Angular application, you need to follow these steps:
2.1. Create an Interceptor
Interceptors are TypeScript classes that implement the HttpInterceptor interface. They typically have an intercept method, which is called for every HTTP request. You can create multiple interceptors to handle different aspects of request processing.
typescript import { Injectable } from '@angular/core'; import { HttpInterceptor, HttpEvent, HttpHandler, HttpRequest, } from '@angular/common/http'; import { Observable } from 'rxjs'; @Injectable() export class MyInterceptor implements HttpInterceptor { intercept( req: HttpRequest<any>, next: HttpHandler ): Observable<HttpEvent<any>> { // Add your logic here return next.handle(req); } }
2.2. Register Interceptors
To make interceptors work, you need to register them with Angular’s Dependency Injection system. You can do this by providing them in the HTTP_INTERCEPTORS multi-provider token. This token allows you to add multiple interceptors in a specific order of execution.
typescript import { NgModule } from '@angular/core'; import { HTTP_INTERCEPTORS } from '@angular/common/http'; @NgModule({ providers: [ { provide: HTTP_INTERCEPTORS, useClass: MyInterceptor, multi: true, }, // Add more interceptors here if needed ], }) export class MyModule {}
2.3. Configure Interceptors
Interceptors can be configured to execute conditionally, depending on the request or response. You can add conditions within the intercept method to determine when the interceptor should apply its logic.
typescript import { Injectable } from '@angular/core'; import { HttpInterceptor, HttpEvent, HttpHandler, HttpRequest, } from '@angular/common/http'; import { Observable } from 'rxjs'; @Injectable() export class ConditionalInterceptor implements HttpInterceptor { intercept( req: HttpRequest<any>, next: HttpHandler ): Observable<HttpEvent<any>> { if (req.url.includes('special-api')) { // Apply interceptor logic only for requests to 'special-api' // Add your logic here } return next.handle(req); } }
2.4. Order of Execution
Interceptors are executed in the order in which they are provided. Angular processes each interceptor’s intercept method sequentially, with the response of one interceptor being passed as input to the next.
It’s important to consider the order of execution when registering interceptors. For example, if you have an interceptor that adds authentication headers, it should come before an interceptor that logs responses.
typescript @NgModule({ providers: [ { provide: HTTP_INTERCEPTORS, useClass: AuthInterceptor, multi: true, }, { provide: HTTP_INTERCEPTORS, useClass: LoggingInterceptor, multi: true, }, ], }) export class MyModule {}
3. Real-World Use Cases for Angular Interceptors
Now that you have a good understanding of what Angular interceptors are and how to use them, let’s explore some real-world use cases where they can greatly benefit your application.
3.1. Authentication and Token Refresh
Interceptors can handle token-based authentication seamlessly. When a user logs in or a token expires, you can use an interceptor to refresh the token and automatically attach it to subsequent requests. This ensures that users remain authenticated without manually updating tokens in each API call.
typescript @Injectable() export class AuthInterceptor implements HttpInterceptor { constructor(private authService: AuthService) {} intercept( req: HttpRequest<any>, next: HttpHandler ): Observable<HttpEvent<any>> { const authToken = this.authService.getToken(); if (authToken) { const authReq = req.clone({ setHeaders: { Authorization: `Bearer ${authToken}`, }, }); return next.handle(authReq); } else { return next.handle(req); } } }
3.2. Caching for Improved Performance
Caching responses using interceptors can significantly improve application performance. You can store frequently requested data in memory and serve it directly from the cache, reducing the load on the server and decreasing latency.
typescript @Injectable() export class CacheInterceptor implements HttpInterceptor { private cache = new Map<string, HttpResponse<any>>(); intercept( req: HttpRequest<any>, next: HttpHandler ): Observable<HttpEvent<any>> { if (req.method !== 'GET') { return next.handle(req); } const cachedResponse = this.cache.get(req.url); if (cachedResponse) { return of(cachedResponse); } return next.handle(req).pipe( tap((event) => { if (event instanceof HttpResponse) { this.cache.set(req.url, event); } }) ); } }
3.3. Error Handling and Logging
Interceptors can centralize error handling and logging, making it easier to manage errors throughout your application. You can log error responses, display user-friendly error messages, and even perform automatic retries for certain types of errors.
typescript @Injectable() export class ErrorHandlingInterceptor implements HttpInterceptor { constructor(private toastr: ToastrService) {} intercept( req: HttpRequest<any>, next: HttpHandler ): Observable<HttpEvent<any>> { return next.handle(req).pipe( catchError((error) => { if (error instanceof HttpErrorResponse) { // Log the error console.error('HTTP Error:', error); // Show a user-friendly error message this.toastr.error('An error occurred. Please try again later.'); // Optionally, perform automatic retries for certain errors if (error.status === 500) { return next.handle(req); } } return throwError(error); }) ); } }
3.4. Request and Response Transformation
Interceptors can transform request data before it is sent to the server and response data before it reaches your components. This is useful for adapting data formats to match your application’s requirements.
typescript @Injectable() export class DataTransformationInterceptor implements HttpInterceptor { intercept( req: HttpRequest<any>, next: HttpHandler ): Observable<HttpEvent<any>> { if (req.url.includes('/api/transform')) { const modifiedReq = req.clone({ body: this.transformRequestData(req.body), }); return next.handle(modifiedReq).pipe( map((event) => { if (event instanceof HttpResponse) { event = event.clone({ body: this.transformResponseData(event.body), }); } return event; }) ); } return next.handle(req); } private transformRequestData(data: any): any { // Transform request data here return data; } private transformResponseData(data: any): any { // Transform response data here return data; } }
Conclusion
Angular interceptors are a powerful tool for centralizing HTTP request handling in your web applications. They provide a flexible and modular way to enhance security, improve performance, and simplify error handling and logging. By using interceptors effectively, you can streamline the development process and ensure a more robust and efficient frontend for your users.
In this guide, we’ve covered the basics of Angular interceptors, how to create and register them, and real-world use cases where they can make a significant difference. As you continue to explore and use Angular interceptors, you’ll discover even more ways to optimize your web applications and provide a better user experience.
So, start integrating interceptors into your Angular projects today and harness the full potential of centralized HTTP request handling. Your users will thank you for the improved security and performance of your web applications.
Table of Contents
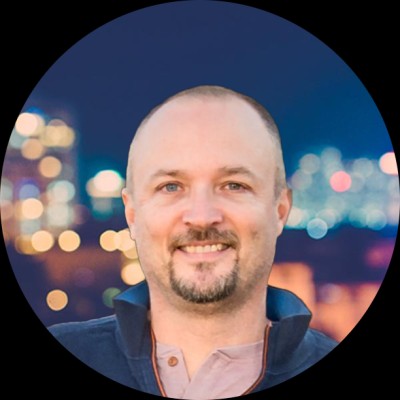
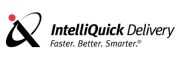