Exploring Angular Internationalization: Building Global Apps
In our increasingly interconnected world, building applications that cater to a global audience is not just a choice but a necessity. Angular, one of the most popular JavaScript frameworks, provides robust internationalization (i18n) capabilities to help developers create applications that can be easily adapted for users around the world. In this comprehensive guide, we will delve into the world of Angular internationalization, covering everything from localization to translation and best practices for building truly global apps.
Table of Contents
1. Introduction to Angular Internationalization (i18n)
Before we dive into the intricacies of Angular internationalization, let’s establish a basic understanding of what i18n is and why it’s crucial for global application development.
1.1. What is Internationalization (i18n)?
Internationalization, often abbreviated as i18n (due to the 18 letters between ‘i’ and ‘n’ in the word), is the process of designing and developing software in a way that allows it to be easily adapted to different languages, regions, and cultures. It involves separating the application’s user interface from its content to facilitate translation and localization.
1.2. Why is i18n Important?
Building applications that are not limited by language or location is essential for reaching a wider audience. By incorporating i18n into your Angular project, you ensure that your app can be seamlessly translated into various languages and tailored to meet the needs of users from diverse backgrounds.
2. Setting Up Your Angular Project for i18n
To start building a globally-ready Angular app, you’ll need to set up your project to support internationalization. Here’s a step-by-step guide to getting started.
2.1. Creating a New Angular Project
If you don’t have an Angular project already, you can create one using the Angular CLI (Command Line Interface). Open your terminal and run the following command:
bash ng new my-global-app
This command will generate a new Angular project named “my-global-app.”
2.2. Adding Angular’s i18n Support
Angular offers built-in i18n support, making it relatively straightforward to internationalize your app. To enable i18n support, navigate to your project’s root folder and run the following command:
bash ng add @angular/localize
This command will add the necessary dependencies and configuration for i18n to your project.
3. Localization in Angular
Localization is the process of adapting your application to a specific locale, which includes formatting dates, numbers, and currencies according to the conventions of that locale. Angular makes localization easier through its built-in tools.
3.1. Understanding Locale and Formats
In Angular, a “locale” represents a specific geographical or cultural region. Each locale has its own set of formatting rules for various data types, such as numbers and dates. Angular’s internationalization tools allow you to work with these locales seamlessly.
3.2. Configuring the Default Locale
By default, Angular uses the locale of the user’s browser. However, you can configure a specific default locale for your application. This is useful when you want to ensure a consistent experience for all users, regardless of their browser settings.
To set the default locale, open your angular.json file and locate the “i18n” section. Add your desired default locale like this:
json "i18n": { "sourceLocale": "en-US", "locales": { "fr-FR": "src/locale/messages.fr.xlf" } }
In this example, we’ve set the default locale to “en-US.”
3.3. Handling Pluralization
Pluralization is a crucial aspect of localization, as different languages have varying rules for plural forms. Angular provides a powerful tool called the ngPlural directive to handle pluralization gracefully. Here’s a code sample demonstrating its usage:
html <p [ngPlural]="itemCount"> <ng-container *ngPluralCase="'=0'">No items</ng-container> <ng-container *ngPluralCase="'=1'">One item</ng-container> <ng-container *ngPluralCase="'other'">{{ itemCount }} items</ng-container> </p>
In this example, the ngPlural directive takes the itemCount variable as input and displays different messages based on its value.
4. Translating Your Angular App
Once your application is properly localized, the next step is to translate its content into multiple languages. Angular offers a straightforward way to achieve this with its translation tools.
4.1. Extracting Messages for Translation
Before you can translate your app, you need to extract all the translatable messages from your code. Angular provides a CLI command for this purpose:
bash ng extract-i18n
This command generates a message file (messages.xlf) containing all the translatable text in your project. You can then send this file to translators or translation services to get it translated into different languages.
4.2. Creating Translation Files
Angular uses XLIFF (XML Localization Interchange File Format) files for storing translations. To create translation files for a specific language, you can use the ng xi18n command followed by the –output-path flag to specify the directory where the translation files should be generated:
bash ng xi18n --output-path=src/locale --locale=fr
This command creates a translation file for the French language in the specified src/locale directory.
4.3. Using the Translate Pipe
Once you have your translation files ready, you can use the translate pipe to display translated content in your templates. Here’s an example of how to use it:
html <p>{{ 'Hello, World!' | translate }}</p>
In this example, the translate pipe will look up the translation for the string “Hello, World!” in the current locale and display the appropriate translation.
5. Best Practices for Angular Internationalization
While Angular’s i18n tools make it relatively easy to internationalize your app, there are some best practices you should follow to ensure a smooth and efficient localization process.
5.1. Organizing Your Translation Files
As your application grows, you may end up with a significant number of translation files. To keep your project organized, consider creating subdirectories within your src/locale folder to group translation files by language or region. For example:
bash src/locale/ en/ messages.en.xlf fr/ messages.fr.xlf es/ messages.es.xlf
This structure makes it easier to manage and maintain your translation files.
5.2. Testing and Maintaining Translations
Regularly test your translations to ensure they display correctly in your application. Automated testing tools can help identify issues with missing or incorrect translations. Additionally, consider using continuous integration (CI) pipelines to automate the translation process and catch errors early.
5.3. Handling Dynamic Content
Some content in your application may be dynamic, such as user-generated text or data fetched from external sources. To ensure these dynamic elements are also translated, implement a strategy for handling dynamic content. This may involve using placeholders in your translation strings and replacing them with dynamic data at runtime.
Conclusion
Building global applications that cater to users from diverse linguistic and cultural backgrounds is no longer a luxury but a necessity. Angular’s internationalization (i18n) capabilities empower developers to create applications that can easily adapt to different languages and regions.
In this guide, we explored the fundamentals of Angular internationalization, from setting up your project for i18n to localizing, translating, and implementing best practices. With this knowledge in hand, you’re well-equipped to embark on your journey of building truly global Angular applications. By embracing i18n, you can expand your app’s reach and provide an inclusive experience for users worldwide. Happy coding!
Table of Contents
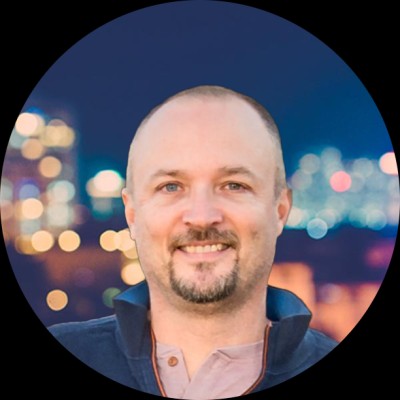
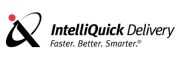