Angular Localization: Creating Multi-Language Applications
In today’s globalized world, it is crucial for web developers to cater to users from different linguistic backgrounds. Creating a multi-language application not only expands your potential user base but also enhances the user experience for non-native English speakers. Angular, as a popular front-end framework, offers robust localization support to build applications that are accessible and engaging to users from diverse regions.
Table of Contents
In this blog, we will explore the concept of localization, delve into Angular’s built-in internationalization (i18n) features, and learn how to create multi-language applications efficiently. By the end of this tutorial, you’ll have a clear understanding of how to implement localization in your Angular projects, empowering you to reach a wider audience.
1. Understanding Localization
1.1. What is Localization?
Localization is the process of adapting a web application to a specific language, region, or culture, ensuring that users from different locales can interact with the application effortlessly. It involves translating text, date formats, numbers, currencies, and other elements, thereby creating a seamless experience for users regardless of their native language.
1.2. The Need for Localization in Angular Applications
Angular’s wide adoption across the globe necessitates providing localized versions of applications. Without localization, non-English-speaking users may find it challenging to navigate an application, leading to decreased user satisfaction and engagement. To address this, Angular offers robust i18n support, enabling developers to create applications that dynamically adapt to various languages.
2. Getting Started with Angular Internationalization (i18n)
2.1. Setting Up a New Angular Project
To begin, make sure you have Node.js and Angular CLI installed on your system. If not, you can install them by following the instructions on the official Node.js and Angular websites.
Now, let’s create a new Angular project using Angular CLI:
bash ng new angular-localization-app cd angular-localization-app
2.2. Enabling i18n in the Angular Project
Angular’s i18n features depend on the use of internationalized HTML templates and message files. To enable i18n in your Angular project, use the following command:
bash ng add @angular/localize
This command installs the necessary packages and configures your project to support localization.
2.3. Creating a Language Translation Template
Once i18n is enabled, you can extract translatable messages from your project’s templates and components into a translation template file. To generate the translation template, run the following command:
bash ng xi18n
This will create an messages.xlf file in the src directory of your project. The file contains all the translatable messages extracted from your project.
2.4. Understanding the Translation File (messages.xlf)
The messages.xlf file follows the XML Localization Interchange File Format (XLIFF). It contains source messages, which are the original translatable strings, and target messages, which are the translated versions of those strings.
Here’s an example of how a message looks in the messages.xlf file:
xml <trans-unit id="greeting" datatype="html"> <source>Hello, world!</source> <target>¡Hola, mundo!</target> </trans-unit>
The <source> tag contains the original message in the default language (usually English), while the <target> tag holds the translated message in the target language.
2.5. Creating Translations for Different Languages
To create translations for different languages, you need to manually translate the messages in the messages.xlf file. Here’s an example of how the file would look after translating the messages into Spanish:
xml <trans-unit id="greeting" datatype="html"> <source>Hello, world!</source> <target>¡Hola, mundo!</target> </trans-unit> <trans-unit id="farewell" datatype="html"> <source>Goodbye!</source> <target>¡Adiós!</target> </trans-unit>
Repeat this process for all the languages you want to support in your application.
2.6. Configuring Angular to Use a Specific Language
To configure Angular to use a specific language, you need to tell the application which translation file to load. This can be achieved by updating the angular.json file:
json { ... "projects": { "angular-localization-app": { ... "architect": { "build": { ... "options": { "localize": ["en", "es"] } } } } } }
In this example, we have specified “localize”: [“en”, “es”], indicating that the application should load English (en) as the default language and Spanish (es) as an additional supported language.
3. Using Localization in Angular Components
With i18n set up and translations available, let’s learn how to use localization in Angular components.
3.1. Displaying Translated Text in Templates
To display translated text in templates, you can use the Angular i18n attribute with the i18n pipe. The i18n attribute marks the translatable text, while the i18n pipe triggers the translation based on the current locale.
Here’s an example of how to use localization in a component template:
html <h1 i18n="@@greeting">Hello, world!</h1> <p i18n="@@farewell">Goodbye!</p>
In this example, the i18n attribute’s value, such as @@greeting, is an identifier used to map the template text to its corresponding translation in the messages.xlf file.
3.2. Language Switching
To allow users to switch between different languages, you can create a language switcher component or integrate language selection into your application’s settings.
In the language switcher component, you can use Angular’s LOCALE_ID token from @angular/core to set the locale based on the user’s language selection.
Here’s an example of how to implement a simple language switcher:
typescript import { Component, Inject } from '@angular/core'; import { LOCALE_ID } from '@angular/core'; @Component({ selector: 'app-language-switcher', template: ` <select (change)="changeLanguage($event.target.value)"> <option value="en">English</option> <option value="es">Español</option> </select> `, }) export class LanguageSwitcherComponent { constructor(@Inject(LOCALE_ID) private localeId: string) {} changeLanguage(language: string) { this.localeId = language; } }
In this example, we use Angular’s dependency injection to access the LOCALE_ID token, and the changeLanguage method allows the user to update the selected language dynamically.
3.3. Pluralization and Date/Number Formatting
Localization is not just about translating text; it also involves handling plural forms and formatting dates and numbers based on the target language’s conventions. Angular’s ngPlural, DatePipe, and DecimalPipe help handle these scenarios efficiently.
Here’s an example of using ngPlural for pluralization:
html <div i18n> {totalItems, plural, =0 {No items} =1 {One item} other {# items} } </div>
In this example, we use the ngPlural directive to handle pluralization based on the value of totalItems. The other block will be used if totalItems is not equal to 0 or 1.
3.3.1. Date and Number Formatting
Angular provides the DatePipe and DecimalPipe to format dates and numbers, respectively, according to the user’s locale.
html <p> Today is {{ today | date }} </p> <p> Total Revenue: {{ revenue | number: '1.2-2' }} </p>
In this example, the DatePipe is used to format the today variable as a date string, while the DecimalPipe formats the revenue variable as a number with two decimal places.
3.3.2. Handling Right-to-Left (RTL) Languages
Some languages are written from right to left, such as Arabic and Hebrew. When translating your application for RTL languages, you must ensure that the layout and styles are appropriately mirrored.
Angular provides the dir attribute, which you can use to set the text direction based on the user’s language. For instance:
html <div [dir]="isRTL ? 'rtl' : 'ltr'"> <!-- Content goes here --> </div>
In this example, the isRTL variable determines whether the content should be displayed from right to left or left to right.
4. Localizing Services and Constants
So far, we have seen how to localize the text and format dates and numbers. However, you may also need to translate service messages and constants.
4.1. Localizing Service Messages
Suppose you have a service that displays success and error messages to users. To ensure these messages are displayed in the user’s preferred language, you can use Angular’s TranslateService. First, you need to import and set up the TranslateService in your application:
typescript import { Injectable } from '@angular/core'; import { TranslateService } from '@ngx-translate/core'; @Injectable({ providedIn: 'root', }) export class MyService { constructor(private translate: TranslateService) {} }
Next, you’ll need to provide translations for your service messages. To do this, you can create a JSON file for each supported language and load them using the TranslateService.
typescript // English translation (en.json) { "successMessage": "Action completed successfully!", "errorMessage": "An error occurred while performing the action.", ... } typescript Copy code // Spanish translation (es.json) { "successMessage": "¡Acción completada con éxito!", "errorMessage": "Se produjo un error al realizar la acción.", ... }
Then, in your MyService, you can use the TranslateService to fetch the appropriate message based on the user’s language:
typescript import { Injectable } from '@angular/core'; import { TranslateService } from '@ngx-translate/core'; @Injectable({ providedIn: 'root', }) export class MyService { constructor(private translate: TranslateService) {} showSuccessMessage() { const message = this.translate.instant('successMessage'); // Display the success message to the user } showErrorMessage() { const message = this.translate.instant('errorMessage'); // Display the error message to the user } }
4.2. Localizing Constants
If your application uses constants, such as configuration settings or labels, you can also localize them. Create a JSON file for each supported language and define the translations accordingly:
typescript // English translation (en.json) { "appTitle": "My Angular App", ... } typescript Copy code // Spanish translation (es.json) { "appTitle": "Mi Aplicación de Angular", ... }
Now, you can use the TranslateService in your component to fetch the localized constants:
typescript import { Component } from '@angular/core'; import { TranslateService } from '@ngx-translate/core'; @Component({ selector: 'app-root', template: ` <h1>{{ appTitle }}</h1> `, }) export class AppComponent { appTitle: string; constructor(private translate: TranslateService) { this.appTitle = this.translate.instant('appTitle'); } }
Conclusion
Congratulations! You have successfully learned how to create multi-language applications in Angular using localization. By leveraging Angular’s built-in i18n features, you can now translate text, format dates and numbers, handle pluralization, and provide localized services and constants.
Creating multi-language applications not only widens your user base but also demonstrates your commitment to inclusivity and user experience. By accommodating users from different linguistic backgrounds, you create a more accessible and user-friendly application, fostering engagement and satisfaction among your global audience.
Now it’s time to put your knowledge into practice and start building applications that resonate with users from around the world. Happy coding and best of luck with your multi-language Angular projects!
Table of Contents
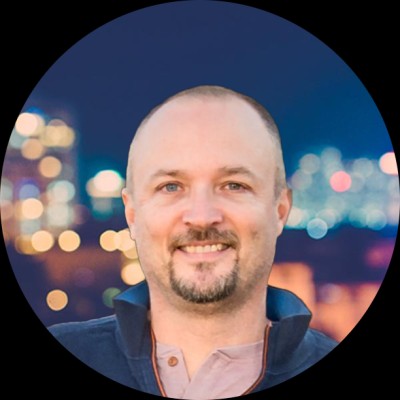
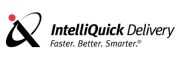