Exploring Angular Material Components: Building UIs Faster
In today’s fast-paced world of web development, building user interfaces that are not only visually appealing but also responsive and feature-rich is crucial. However, developing UI components from scratch can be time-consuming and can divert your focus from the core functionality of your web application. This is where Angular Material comes to the rescue. In this blog post, we will delve deep into Angular Material components, exploring how they can help you build UIs faster and more efficiently.
Table of Contents
1. What is Angular Material?
Angular Material is a UI component library for Angular applications. Developed and maintained by the Angular team at Google, it provides a set of high-quality, pre-designed UI components that can be easily integrated into your Angular applications. These components follow the Material Design guidelines, ensuring a consistent and aesthetically pleasing user experience.
2. Benefits of Using Angular Material Components
Before we dive into the practical aspects, let’s explore some of the key benefits of using Angular Material components in your web development projects:
2.1. Consistency
Angular Material components are designed following the Material Design principles. This means that your application will have a consistent and modern look and feel, improving the overall user experience.
2.2. Time Efficiency
One of the most significant advantages of Angular Material is that it saves you a substantial amount of development time. You don’t need to create UI components from scratch, allowing you to focus on the core functionality of your application.
2.3. Customization
While Angular Material components come with predefined styles, they are highly customizable. You can easily adapt their appearance to match your application’s branding and style guidelines.
2.4. Responsiveness
Angular Material components are built with responsiveness in mind. They adapt seamlessly to different screen sizes and devices, ensuring your application looks great on desktops, tablets, and smartphones.
2.5. Accessibility
Accessibility is a crucial aspect of web development. Angular Material components are designed with accessibility in mind, making it easier for you to create web applications that are usable by a wider audience, including people with disabilities.
Now that we’ve covered the advantages let’s dive into the practical side of using Angular Material components.
3. Getting Started with Angular Material
To start using Angular Material in your Angular project, you need to follow these steps:
3.1. Create an Angular Project
If you don’t have an Angular project already, you can create one using the Angular CLI (Command Line Interface). Open your terminal and run the following command:
bash ng new my-angular-app
3.2. Install Angular Material
Once your Angular project is set up, you can install Angular Material using npm (Node Package Manager). Navigate to your project directory and run:
bash ng add @angular/material
This command will install Angular Material and prompt you to choose a theme for your application.
3.3. Import Angular Material Modules
After installation, you need to import the Angular Material modules you want to use in your application. Open the app.module.ts file and add the following imports:
typescript import { MatButtonModule } from '@angular/material/button'; import { MatIconModule } from '@angular/material/icon'; // Import other modules as needed @NgModule({ declarations: [ // ... ], imports: [ // ... MatButtonModule, MatIconModule, // Add other modules here ], bootstrap: [AppComponent] }) export class AppModule { }
Make sure to import only the modules you plan to use to keep your application’s bundle size minimal.
4. Exploring Angular Material Components
Now that you have Angular Material set up in your project, let’s explore some of the commonly used components and see how they can save you time and effort.
4.1. Buttons
Buttons are a fundamental part of any web application’s UI. Angular Material provides a MatButton component that you can use to create various types of buttons with different styles.
html <!-- Example of a raised button --> <button mat-raised-button>Click me</button> <!-- Example of an icon button --> <button mat-icon-button> <mat-icon>favorite</mat-icon> </button>
Angular Material buttons come with built-in styling and ripple effects, saving you the trouble of writing CSS for basic button functionality.
4.2. Icons
Icons are essential for enhancing the visual appeal of your user interface. Angular Material makes it easy to incorporate icons into your application using the MatIcon component.
html <!-- Example of using a Material Design icon --> <mat-icon>home</mat-icon> <!-- Example of using a custom SVG icon --> <mat-icon svgIcon="my-custom-icon"></mat-icon>
You can use Material Design icons or even add custom SVG icons to suit your application’s requirements.
4.3. Navigation
Navigation is a critical aspect of user experience. Angular Material provides several navigation components like MatToolbar and MatSidenav that simplify the creation of navigation menus and toolbars.
html <!-- Example of a simple toolbar --> <mat-toolbar color="primary"> <span>My App</span> </mat-toolbar> <!-- Example of a side navigation menu --> <mat-sidenav-container> <mat-sidenav opened>Menu content goes here</mat-sidenav> <mat-sidenav-content>Main content goes here</mat-sidenav-content> </mat-sidenav-container>
These components come with built-in responsiveness, ensuring your navigation elements work seamlessly across different devices.
4.4. Forms
Forms are a crucial part of web applications for user input and data collection. Angular Material offers a set of form components that can significantly speed up form creation.
html <!-- Example of an input field --> <mat-form-field> <input matInput placeholder="Username"> </mat-form-field> <!-- Example of a datepicker --> <mat-form-field> <input matInput [matDatepicker]="picker" placeholder="Choose a date"> <mat-datepicker-toggle matSuffix [for]="picker"></mat-datepicker-toggle> <mat-datepicker #picker></mat-datepicker> </mat-form-field>
Angular Material provides form controls, input fields, datepickers, and other form-related components with built-in validation and error handling.
4.5. Dialogs
Dialogs are often used for displaying important information or collecting user input. Angular Material’s MatDialog component simplifies the process of creating dialogs.
typescript import { MatDialog } from '@angular/material/dialog'; import { MyDialogComponent } from './my-dialog.component'; // Open a dialog const dialogRef = this.dialog.open(MyDialogComponent); // Handle dialog result dialogRef.afterClosed().subscribe(result => { console.log(`Dialog result: ${result}`); });
With Angular Material, you can create custom dialogs and modals effortlessly.
5. Customizing Angular Material Components
While Angular Material components come with predefined styles, you can easily customize them to fit your application’s branding and design guidelines. Here are a few ways to do that:
5.1. Theming
Angular Material allows you to create custom themes to match your application’s visual identity. You can define your color palette, typography, and more using the mat-theme mixin in your application’s stylesheet.
scss @import '~@angular/material/theming'; // Define your custom theme $custom-theme: mat.define-dark-theme( ( color: ( primary: $custom-primary-color, accent: $custom-accent-color, warn: $custom-warn-color, ), typography: $custom-typography-config, ) ); // Apply the custom theme @include mat.all-component-themes($custom-theme);
5.2. Component Styling
You can override the default styles of Angular Material components by using CSS classes or component-specific styling.
css /* Example: Styling a button */ .custom-button { background-color: #007acc; color: #fff; } <!-- Apply the custom style to a button --> <button mat-raised-button class="custom-button">Custom Button</button>
5.3. Customizing Icons
To use custom SVG icons, you can define them in your application and use the MatIconRegistry and DomSanitizer to make them available to Angular Material.
typescript import { MatIconRegistry } from '@angular/material/icon'; import { DomSanitizer } from '@angular/platform-browser'; // Register custom SVG icons const svgIcon = 'my-custom-icon'; const svgURL = this.sanitizer.bypassSecurityTrustResourceUrl('path/to/your/custom-icon.svg'); this.matIconRegistry.addSvgIcon(svgIcon, svgURL);
Conclusion
Angular Material is a powerful tool for building user interfaces quickly and efficiently in your Angular applications. Its extensive collection of pre-designed components, along with customization options, makes it a go-to choice for developers looking to save time and maintain a consistent design in their web applications.
By leveraging Angular Material, you can focus more on implementing the core functionality of your application and less on the nitty-gritty details of UI development. So, the next time you start a new Angular project or want to revamp an existing one, consider Angular Material as your UI component library to supercharge your development process. With Angular Material, you’ll be able to create stunning user interfaces with ease, leaving a lasting impression on your users.
Table of Contents
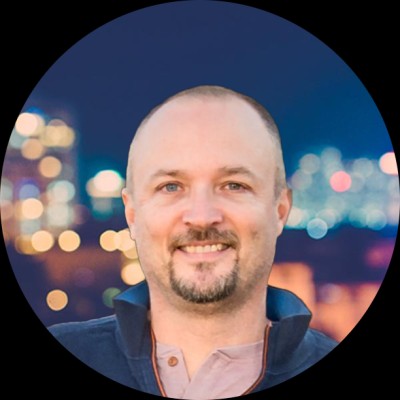
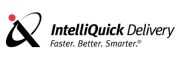