Angular Material Design: Creating Beautiful User Interfaces
In today’s digital age, user experience plays a pivotal role in the success of any web application. The appearance and usability of an application are critical factors that significantly impact user engagement and retention. Angular Material Design offers a solution to this challenge, enabling developers to create visually appealing and user-friendly interfaces effortlessly. In this blog post, we will delve into the world of Angular Material Design, exploring its key features, components, and how to use it to craft beautiful user interfaces. So, let’s dive in!
Table of Contents
1. What is Angular Material Design?
Angular Material Design is a UI component library for Angular applications, built on top of Google’s Material Design principles. Material Design is a design language developed by Google that emphasizes a clean, consistent, and modern look across different platforms and devices. Angular Material Design adopts these principles and provides a rich set of UI components, styles, and animations that enable developers to create visually stunning and consistent user interfaces.
2. Setting Up Angular Material Design
To get started with Angular Material Design, you need an Angular project up and running. If you haven’t set up an Angular project yet, you can do so using the Angular CLI:
bash ng new my-angular-app cd my-angular-app
Once your project is set up, you can install Angular Material and its dependencies using npm:
bash npm install @angular/material @angular/cdk @angular/animations
Next, you’ll need to import the necessary Angular Material modules into your application’s main module:
typescript // src/app/app.module.ts import { NgModule } from '@angular/core'; import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; import { MatButtonModule } from '@angular/material/button'; import { MatInputModule } from '@angular/material/input'; import { MatIconModule } from '@angular/material/icon'; // Import other required modules @NgModule({ imports: [ BrowserAnimationsModule, MatButtonModule, MatInputModule, MatIconModule, // Other modules go here ], // Other declarations, providers, etc. }) export class AppModule { }
With the setup complete, you are now ready to leverage the power of Angular Material Design in your application.
3. Key Features of Angular Material Design
Angular Material Design comes with a wide array of features that simplify UI development and provide a consistent user experience. Let’s explore some of its key features:
3.1. Material Components
Angular Material Design offers a collection of ready-to-use UI components, such as buttons, cards, dialogs, menus, and more. These components are designed following the Material Design guidelines, ensuring a visually pleasing appearance and seamless integration into your application.
3.2. Theming
Theming is a crucial aspect of Angular Material Design, allowing developers to customize the look and feel of their applications. By using predefined color palettes or creating custom themes, you can achieve a unique and cohesive design that aligns with your brand identity.
3.3. Accessibility
Angular Material Design places a strong emphasis on accessibility. The components are designed and developed with accessibility best practices in mind, making it easier for users with disabilities to interact with your application.
3.4. Animations
Smooth animations enhance the overall user experience, and Angular Material Design provides various built-in animations for components. These animations help create fluid transitions, adding a touch of elegance to your UI.
3.5. Responsive Design
With mobile devices becoming increasingly prevalent, responsive design is a must. Angular Material Design ensures that the components adapt gracefully to different screen sizes and orientations, guaranteeing a seamless user experience across all devices.
4. Exploring Key Components
Let’s explore some of the essential Angular Material Design components and how to use them in your application.
4.1. Buttons
Buttons are fundamental UI elements for triggering actions. Angular Material Design provides a range of button styles, including raised buttons, flat buttons, and icon buttons. To use buttons in your application, follow these steps:
html <!-- src/app/app.component.html --> <button mat-raised-button>Click Me!</button> <button mat-flat-button>Cancel</button> <button mat-icon-button><mat-icon>favorite</mat-icon></button>
In this example, we have used mat-raised-button, mat-flat-button, and mat-icon-button directives to create different types of buttons.
4.2. Cards
Cards are a versatile component used to present information in a structured manner. They are commonly used for displaying snippets of content, product cards, or user profiles. To use cards in your application, follow these steps:
html <!-- src/app/app.component.html --> <mat-card> <mat-card-header> <div mat-card-avatar></div> <mat-card-title>John Doe</mat-card-title> <mat-card-subtitle>Software Engineer</mat-card-subtitle> </mat-card-header> <mat-card-content> <p> Hello, I am John Doe, a software engineer passionate about crafting beautiful user interfaces with Angular Material Design. </p> </mat-card-content> </mat-card>
In this example, we’ve used the mat-card directive to create a card with a header and content sections.
4.3. Icons
Icons play a crucial role in modern UI design. Angular Material Design makes it easy to include Material icons in your application. To use icons, follow these steps:
html <!-- src/app/app.component.html --> <mat-icon>favorite</mat-icon>
Here, we’ve used the mat-icon directive to display the “favorite” icon. You can replace “favorite” with the name of any Material icon to use it.
4.4. Inputs
Input fields are used to gather user data, such as text, numbers, or dates. Angular Material Design provides stylized and interactive input fields. To use inputs, follow these steps:
html <!-- src/app/app.component.html --> <mat-form-field> <mat-label>Enter your name</mat-label> <input matInput placeholder="Name"> </mat-form-field>
In this example, we’ve used the mat-form-field and matInput directives to create a styled input field with a floating label.
Conclusion
Angular Material Design empowers developers to build beautiful and intuitive user interfaces with ease. Its vast collection of components, theming capabilities, animations, and accessibility features make it a valuable tool for crafting visually appealing and user-friendly applications. By following the steps outlined in this blog post, you can get started with Angular Material Design and take your UI development to new heights. Embrace the power of Material Design principles, and delight your users with stunning interfaces that leave a lasting impression. Happy coding!
Remember, this is just the tip of the iceberg when it comes to Angular Material Design. Continue exploring the official documentation and experimenting with various components to unlock the full potential of this remarkable library.
Table of Contents
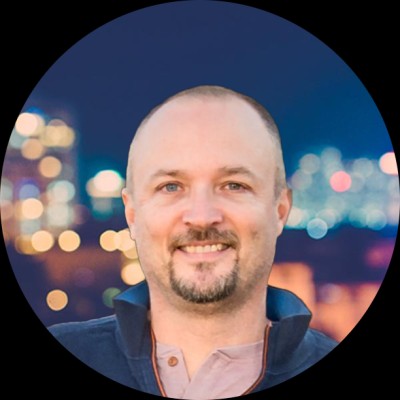
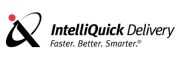