Building Offline-First Applications with Angular and Service Workers
In today’s digitally connected world, web applications are expected to work seamlessly, whether users have an internet connection or not. Users often encounter spotty connectivity, especially on mobile devices, and expect apps to continue functioning even in offline mode. This demand has given rise to the concept of “offline-first” web applications. In this blog post, we’ll explore how to build offline-first applications with Angular and Service Workers.
Table of Contents
1. What Are Offline-First Applications?
Offline-first applications are web applications designed to provide a consistent and responsive user experience, even when there is no internet connection or when connectivity is unreliable. These applications are not dependent on a continuous online connection and can continue to function offline, syncing data and updates with the server when a connection is available.
Building an offline-first application involves implementing various strategies and technologies to ensure that users can perform essential tasks, view cached content, and seamlessly interact with the app, all while offline. One of the key technologies that make this possible is Service Workers.
2. Understanding Service Workers
Service Workers are JavaScript scripts that run in the background of a web application, separate from the main thread. They act as intermediaries between the web application and the network, intercepting network requests and providing programmatic control over network responses.
Service Workers are an essential part of Progressive Web Apps (PWAs) and are used to cache assets and data, making it possible for web applications to work offline. They can intercept requests for assets like HTML, CSS, JavaScript, images, and API responses, allowing developers to serve cached content when the network is unavailable.
3. Why Use Service Workers with Angular?
Angular is a popular JavaScript framework for building dynamic web applications. When combined with Service Workers, Angular becomes a powerful tool for creating offline-first web applications. Here are some reasons why you should consider using Service Workers with Angular:
- Improved Performance: Service Workers can cache assets, reducing the need to download them on subsequent visits. This significantly improves page load times, especially on slower connections.
- Offline Access: Users can access your Angular application even when they are offline. This can be crucial for applications that require access to data in remote locations or for users with intermittent internet access.
- Reduced Data Usage: Cached assets reduce the amount of data transferred over the network, saving users’ data plans and ensuring a better experience on limited connections.
- Enhanced Reliability: By providing a consistent experience regardless of network status, you create a more reliable and user-friendly application.
Now that we understand the benefits of using Service Workers with Angular, let’s dive into the steps required to build an offline-first application.
4. Building an Offline-First Angular Application
To create an offline-first Angular application, you need to follow these steps:
4.1. Set Up an Angular Project
If you don’t already have an Angular project, you can create one using the Angular CLI (Command Line Interface). First, make sure you have the Angular CLI installed globally:
bash npm install -g @angular/cli
Once installed, you can create a new Angular project:
bash ng new offline-first-app
4.2. Create Service Worker Configuration
To use Service Workers in your Angular application, you need to set up a configuration file for the service worker. Angular provides built-in support for this. Run the following command to generate the configuration file:
bash ng add @angular/pwa
This command will set up the necessary configuration files and add the required dependencies to your project.
4.3. Define Offline Behavior
In your Angular application, you should define the behavior you want when the app is offline. For example, you can create a custom offline page or display cached content. You can do this by modifying the service worker’s ngsw-config.json file.
json { "index": "/index.html", "assetGroups": [ // ... ], "dataGroups": [ // ... ], "navigationUrls": [ // ... ], "offline": { "page": "/offline.html", "assets": [ // ... ] }, // ... }
In the above configuration, we specify that when the app is offline, it should serve the /offline.html page along with any cached assets.
4.4. Cache Assets
Service Workers allow you to cache assets and data so that they can be served even when the user is offline. You can define which assets to cache in the ngsw-config.json file or use Angular service workers’ built-in caching mechanisms.
typescript import { Component, OnInit } from '@angular/core'; import { SwUpdate } from '@angular/service-worker'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit { constructor(private swUpdate: SwUpdate) {} ngOnInit() { if (this.swUpdate.isEnabled) { this.swUpdate.available.subscribe(() => { if (confirm('A new version is available. Load it?')) { window.location.reload(); } }); } } }
In the above code snippet, we check for updates from the service worker and prompt the user to reload the page when a new version is available.
4.5. Handle Data Synchronization
For offline-first applications that require data synchronization, you’ll need to implement strategies for syncing data between the client and the server when connectivity is restored. This might involve queuing data requests and sending them when the network is available.
typescript import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; @Injectable({ providedIn: 'root' }) export class DataService { constructor(private http: HttpClient) {} getData(): Observable<any> { // Check if online, if not, fetch data from local storage if (!navigator.onLine) { const cachedData = localStorage.getItem('cachedData'); if (cachedData) { return new Observable((observer) => { observer.next(JSON.parse(cachedData)); observer.complete(); }); } } // If online, fetch data from the server return this.http.get('/api/data'); } }
In this example, the DataService checks if the client is online and, if not, retrieves cached data from local storage.
5. Testing Your Offline-First Angular App
Testing your offline-first Angular application is crucial to ensure it behaves as expected in various network conditions. Here are some testing scenarios to consider:
5.1. Online Testing
Test your application under normal online conditions to ensure that it functions correctly when there is a stable internet connection. Verify that data is fetched from the server and that updates are sent successfully.
5.2. Offline Testing
Simulate offline conditions by disconnecting your device from the internet or using browser developer tools to disable the network. Verify that your application still loads and displays cached content. Test whether user interactions, such as form submissions, are queued and sent when the device regains connectivity.
5.3. Service Worker Updates
Ensure that your service worker updates are working as expected. Deploy a new version of your application, and verify that users are prompted to reload the page when an update is available.
5.4. Data Synchronization
Test data synchronization by sending data from the client to the server while offline and ensuring it syncs when connectivity is restored. Check for edge cases, such as conflicts or data loss, and implement appropriate handling.
Conclusion
Building offline-first applications with Angular and Service Workers is essential for providing a robust and reliable user experience. By following the steps outlined in this blog post, you can create web applications that continue to function seamlessly, even when users are offline or have unreliable connectivity. Offline-first development is not only about improving user experience but also about making your application more resilient and accessible in a world where connectivity is not always guaranteed. Embrace the power of Service Workers and take your Angular applications to the next level by delivering a true offline-first experience.
Start building your offline-first Angular application today and ensure your users have a smooth experience, whether they are online or offline.
Table of Contents
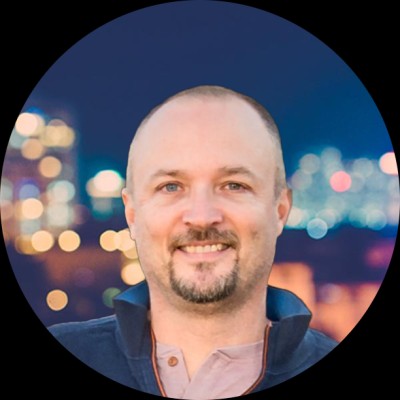
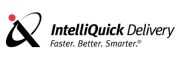