Angular and Redux: State Management in Web Applications
State management is a crucial aspect of web development, especially in complex applications. As the scale and complexity of web applications increase, handling the application’s state becomes more challenging. State management libraries and patterns have emerged to help developers address this problem effectively.
Table of Contents
In this blog, we will explore how Angular and Redux can work together to provide efficient state management in web applications. Angular is a popular and powerful frontend framework, while Redux is a predictable state container that can be used with any frontend library. Combining these two technologies can help us manage state in a scalable and maintainable way.
1. Understanding State Management
Before diving into the details of Angular and Redux integration, let’s first understand the concept of state management. In web applications, state refers to the current data and its structure at a particular point in time. The state can be anything from the user’s authentication status, UI preferences, or data fetched from the server.
Managing state effectively is crucial for several reasons:
- Predictability: With proper state management, the application’s behavior becomes predictable, making it easier to debug and maintain the codebase.
- Scalability: As the application grows, managing state becomes more complex. An organized state management system ensures scalability and maintainability.
- Performance: By managing state efficiently, we can avoid unnecessary re-renders and improve application performance.
- Testing: Well-structured state management facilitates testing and helps in writing robust test cases.
2. Introduction to Redux
Redux is a state management library inspired by Flux, but with a more functional approach. It provides a single source of truth for the application state and ensures that state modifications are predictable by following strict guidelines. Redux operates on a unidirectional data flow, which means data flows in a single direction within the application.
Key concepts in Redux:
- Store: The store is a single JavaScript object that holds the entire application state. It is immutable, and the only way to change the state is by dispatching actions.
- Actions: Actions are plain JavaScript objects that represent an event or an intention to change the state. They contain a type property that specifies the action’s type and additional data as needed.
- Reducers: Reducers are pure functions that take the current state and an action as arguments and return the new state based on the action’s type. Reducers should never mutate the original state but create a new state object.
- Dispatch: Dispatching an action is the only way to trigger a state change. When an action is dispatched, it flows through the reducers, and the new state is returned.
- Selectors: Selectors are functions used to extract specific pieces of state from the store. They help in keeping the component logic separate from the state structure.
3. Integrating Redux with Angular
Angular, being a powerful framework, provides various ways to handle state management. However, integrating Redux with Angular can be beneficial, especially in large-scale applications. Redux’s predictable state management can make debugging and testing easier in such scenarios.
To integrate Redux with Angular, follow these steps:
Step 1: Install Dependencies
First, we need to install the required packages. Install @ngrx/store, the official Redux library for Angular, and ngrx/effects if you plan to use side effects in your application.
bash npm install @ngrx/store @ngrx/effects
Step 2: Define the Application State
Before setting up the store, we need to define the application state. Identify the different pieces of state your application requires and create an interface representing the entire state.
typescript // app.state.ts interface AppState { user: User; products: Product[]; cart: CartItem[]; // Add more state properties as needed }
In this example, we have defined a state that includes user details, product information, and the items in the cart.
Step 3: Create Actions
Next, define actions that represent the various events that can modify the state. Create a separate file for actions and list all the actions your application needs.
typescript // app.actions.ts import { createAction, props } from '@ngrx/store'; import { User, Product, CartItem } from './app.state'; // Define actions using `createAction` function const login = createAction('[Auth] Login', props<{ user: User }>()); const logout = createAction('[Auth] Logout'); const loadProducts = createAction('[Products] Load', props<{ products: Product[] }>()); const addToCart = createAction('[Cart] Add', props<{ item: CartItem }>()); // Add more actions as needed
In this example, we have defined actions for user login, logout, loading products, and adding items to the cart.
Step 4: Create Reducers
Now, create reducers to handle each action and modify the state accordingly. Each reducer is responsible for updating a specific piece of the application state.
typescript // app.reducers.ts import { createReducer, on } from '@ngrx/store'; import { login, logout, loadProducts, addToCart } from './app.actions'; import { AppState } from './app.state'; // Define the initial state const initialState: AppState = { user: null, products: [], cart: [], }; // Create reducers using `createReducer` function export const appReducer = createReducer( initialState, on(login, (state, { user }) => ({ ...state, user })), on(logout, (state) => ({ ...state, user: null })), on(loadProducts, (state, { products }) => ({ ...state, products })), on(addToCart, (state, { item }) => ({ ...state, cart: [...state.cart, item] })) // Add more reducer functions as needed );
In this example, we have created reducers for handling login, logout, loading products, and adding items to the cart.
Step 5: Register the Reducer
To use the created reducer, we need to register it with the Angular application. In the root module, import the necessary modules and define the store.
typescript // app.module.ts import { NgModule } from '@angular/core'; import { StoreModule } from '@ngrx/store'; import { appReducer } from './app.reducers'; @NgModule({ imports: [ // Other imports... StoreModule.forRoot({ app: appReducer }), ], // Other declarations and providers... }) export class AppModule { }
In this example, we have registered the appReducer under the key app in the store.
Step 6: Dispatch Actions
Finally, we can now dispatch actions from our components or services to modify the state. To do this, inject the Store into the component or service where you want to trigger an action.
typescript // user.component.ts import { Component } from '@angular/core'; import { Store } from '@ngrx/store'; import { login } from './app.actions'; import { User } from './app.state'; @Component({ selector: 'app-user', template: ` <!-- User login form --> `, }) export class UserComponent { constructor(private store: Store) { } loginUser(user: User) { this.store.dispatch(login({ user })); } }
In this example, we have a UserComponent with a loginUser method that dispatches the login action with the user details.
4. Using Selectors
As the application grows, accessing specific pieces of state directly from the store can become tedious and error-prone. To avoid this, we can use selectors to extract specific parts of the state.
typescript // app.selectors.ts import { createSelector, createFeatureSelector } from '@ngrx/store'; import { AppState } from './app.state'; // Create a feature selector to select the entire state const selectAppState = createFeatureSelector<AppState>('app'); // Create selectors to access specific pieces of state export const selectUser = createSelector( selectAppState, (state) => state.user ); export const selectProducts = createSelector( selectAppState, (state) => state.products ); export const selectCartItems = createSelector( selectAppState, (state) => state.cart );
In this example, we have created selectors to access the user, products, and cart items from the state.
Conclusion
In conclusion, Angular and Redux can be a powerful combination for state management in web applications. Angular provides a robust framework for building complex user interfaces, while Redux ensures a predictable state management pattern. By following the steps outlined in this blog, you can seamlessly integrate Redux into your Angular application and handle state management efficiently.
Remember that state management is a crucial aspect of web development, and choosing the right approach can greatly impact the scalability and maintainability of your application. By adopting Redux and Angular, you can build web applications that are easy to maintain, test, and scale as your project grows.
So, start integrating Redux into your Angular project and unleash the true potential of state management in web applications! Happy coding!
Table of Contents
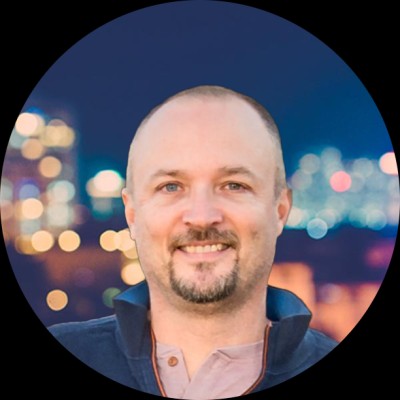
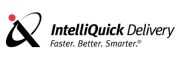