Using Angular with Redux Toolkit: State Management Simplified
State management is a critical aspect of building modern web applications, and when it comes to developing robust and maintainable Angular applications, having a well-structured state management solution is essential. Redux Toolkit is a powerful library that simplifies state management in Angular, making it easier to handle application state and reducing boilerplate code. In this blog post, we’ll explore how to use Angular with Redux Toolkit to streamline your state management process.
Table of Contents
1. Why Use Redux Toolkit with Angular?
Before we dive into the nitty-gritty details, let’s understand why Redux Toolkit is a valuable addition to your Angular project.
1.1. Centralized State Management
In Angular, components often need to share data and state. Managing this shared state can quickly become complex and error-prone. Redux Toolkit provides a centralized store where all your application state is stored, making it accessible to any component without the need for complex data passing between components.
1.2. Predictable State Changes
Redux Toolkit enforces a strict unidirectional data flow, which ensures that state changes are predictable and can be traced easily. This predictability is crucial for debugging and maintaining your Angular application.
1.3. Simplified Development Workflow
With Redux Toolkit, you can write cleaner and more maintainable code. It reduces the boilerplate code typically associated with state management, allowing you to focus on building features rather than managing state.
2. Setting Up Redux Toolkit in Angular
Let’s walk through the steps to set up Redux Toolkit in an Angular application.
2.1. Install Dependencies
Start by installing the required packages using npm or yarn:
bash npm install @ngrx/store @ngrx/effects @ngrx/entity @ngrx/store-devtools
2.2. Create Actions, Reducers, and Effects
In Redux Toolkit, you define actions to describe state changes, reducers to handle those actions, and effects for handling side effects. Here’s an example of how to create these:
Actions
typescript // counter.actions.ts import { createAction, props } from '@ngrx/store'; export const increment = createAction('[Counter] Increment'); export const decrement = createAction('[Counter] Decrement'); export const reset = createAction('[Counter] Reset');
Reducers
typescript // counter.reducer.ts import { createReducer, on } from '@ngrx/store'; import { increment, decrement, reset } from './counter.actions'; export const initialState = 0; export const counterReducer = createReducer( initialState, on(increment, (state) => state + 1), on(decrement, (state) => state - 1), on(reset, () => initialState) );
Effects
typescript // counter.effects.ts import { Injectable } from '@angular/core'; import { Actions, createEffect, ofType } from '@ngrx/effects'; import { of } from 'rxjs'; import { catchError, map, mergeMap } from 'rxjs/operators'; @Injectable() export class CounterEffects { constructor( private actions$: Actions, // Add your services here ) {} // Define your effects here }
2.3. Configure the Store
To configure the store, you’ll need to modify your app module:
typescript // app.module.ts import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { StoreModule } from '@ngrx/store'; import { EffectsModule } from '@ngrx/effects'; import { counterReducer } from './counter.reducer'; @NgModule({ declarations: [AppComponent], imports: [ BrowserModule, StoreModule.forRoot({ count: counterReducer }), EffectsModule.forRoot([]), ], bootstrap: [AppComponent], }) export class AppModule {}
2.4. Dispatch Actions in Components
Now that you have set up Redux Toolkit in your Angular application, you can start dispatching actions in your components to modify the state. Here’s an example of how to do this:
typescript // counter.component.ts import { Component } from '@angular/core'; import { Store } from '@ngrx/store'; import { increment, decrement, reset } from './counter.actions'; @Component({ selector: 'app-counter', template: ` <div> <p>Count: {{ count$ | async }}</p> <button (click)="increment()">Increment</button> <button (click)="decrement()">Decrement</button> <button (click)="reset()">Reset</button> </div> `, }) export class CounterComponent { count$ = this.store.select('count'); constructor(private store: Store) {} increment() { this.store.dispatch(increment()); } decrement() { this.store.dispatch(decrement()); } reset() { this.store.dispatch(reset()); } }
3. Simplifying State Management with Redux Toolkit Features
Redux Toolkit offers several features that simplify state management further. Let’s explore some of them:
3.1. createSlice for Reducers
createSlice is a handy function that generates actions and reducers based on a predefined slice of your state. This simplifies the process of creating reducers and actions. Here’s how you can use it:
typescript // counter.slice.ts import { createSlice } from '@reduxjs/toolkit'; const counterSlice = createSlice({ name: 'counter', initialState: 0, reducers: { increment: (state) => state + 1, decrement: (state) => state - 1, reset: () => 0, }, }); export const { increment, decrement, reset } = counterSlice.actions; export default counterSlice.reducer;
3.2. Immutability Made Easy
Redux Toolkit uses the Immer library under the hood, which allows you to write code that appears to modify state directly while maintaining immutability. This simplifies complex state updates:
typescript // Using Immer with Redux Toolkit on(increment, (state) => { state.count += 1; // Directly modify state })
3.3. DevTools Integration
Redux DevTools extension is an invaluable tool for debugging your application’s state changes. Redux Toolkit seamlessly integrates with DevTools, providing a powerful debugging experience out of the box.
4. Advanced State Management with Redux Toolkit
Redux Toolkit can handle complex state management scenarios effortlessly. Let’s explore some advanced concepts:
4.1. Entity Slices
Entity slices are particularly useful when dealing with collections of data. You can use the createEntityAdapter function to simplify managing arrays of objects. For example, managing a list of todos becomes straightforward:
typescript // todo.slice.ts import { createEntityAdapter, createSlice } from '@reduxjs/toolkit'; const todosAdapter = createEntityAdapter<Todo>({ selectId: (todo) => todo.id, sortComparer: (a, b) => a.date.localeCompare(b.date), }); const todoSlice = createSlice({ name: 'todos', initialState: todosAdapter.getInitialState(), reducers: { addTodo: todosAdapter.addOne, updateTodo: todosAdapter.updateOne, removeTodo: todosAdapter.removeOne, }, }); export const { addTodo, updateTodo, removeTodo } = todoSlice.actions; export const { selectAll: selectAllTodos } = todosAdapter.getSelectors(); export default todoSlice.reducer;
4.2. Async Actions
Handling asynchronous actions like API requests is simplified using the createAsyncThunk function. Here’s an example of fetching data from an API and updating the state:
typescript // user.slice.ts import { createSlice, createAsyncThunk } from '@reduxjs/toolkit'; export const fetchUser = createAsyncThunk('user/fetchUser', async (userId) => { const response = await fetch(`/api/users/${userId}`); return response.json(); }); const userSlice = createSlice({ name: 'user', initialState: { data: null, loading: 'idle', error: null }, reducers: {}, extraReducers: (builder) => { builder .addCase(fetchUser.pending, (state) => { state.loading = 'pending'; }) .addCase(fetchUser.fulfilled, (state, action) => { state.loading = 'fulfilled'; state.data = action.payload; }) .addCase(fetchUser.rejected, (state, action) => { state.loading = 'rejected'; state.error = action.error.message; }); }, }); export default userSlice.reducer;
Conclusion
State management in Angular applications can be a complex task, but Redux Toolkit simplifies the process significantly. By centralizing state, enforcing predictability, and providing helpful features, Redux Toolkit streamlines the development workflow and makes your codebase more maintainable. Whether you’re building a small project or a large-scale application, consider using Redux Toolkit to simplify your state management and boost productivity in your Angular projects.
In this blog post, we’ve only scratched the surface of what Redux Toolkit can do. As you explore it further, you’ll discover even more ways to streamline your Angular application’s state management. So, go ahead and give Redux Toolkit a try in your next Angular project, and experience the benefits of simplified state management firsthand. Happy coding!
Table of Contents
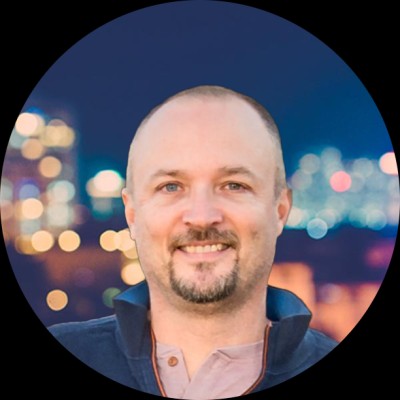
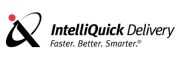