Mastering Angular Routing: Advanced Techniques and Patterns
Angular, one of the most popular front-end frameworks, provides a robust routing system out of the box. Routing is a fundamental part of building modern web applications, allowing users to navigate seamlessly between different views or pages while maintaining a single-page application (SPA) feel. While basic routing in Angular is relatively straightforward, mastering advanced techniques and patterns can significantly enhance your application’s performance, maintainability, and user experience.
Table of Contents
In this blog, we’ll explore advanced Angular routing techniques and patterns that will take your skills to the next level. Whether you’re a seasoned Angular developer looking to deepen your knowledge or a newcomer eager to understand routing intricacies, this guide has something for you.
1. Understanding the Basics of Angular Routing
1.1. Setting Up a Basic Routing Configuration
To get started with Angular routing, you need to set up a basic routing configuration in your application. This typically involves defining routes, specifying their corresponding components, and configuring a default route. Here’s an example:
typescript // app-routing.module.ts import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { HomeComponent } from './home.component'; import { AboutComponent } from './about.component'; const routes: Routes = [ { path: '', component: HomeComponent }, { path: 'about', component: AboutComponent }, // Add more routes here ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
1.2. Navigating Between Routes
Once you have your routes configured, you can use Angular’s routerLink directive to create navigation links in your templates. For example:
html <!-- app.component.html --> <a routerLink="/">Home</a> <a routerLink="/about">About</a>
1.3. Passing Data Between Routes
In many cases, you’ll need to pass data between routes. Angular provides various mechanisms for achieving this, such as route parameters and query parameters. Here’s an example of passing data using route parameters:
typescript // app-routing.module.ts const routes: Routes = [ { path: 'product/:id', component: ProductDetailComponent } // Add more routes here ];
And in your component:
typescript // product-detail.component.ts import { ActivatedRoute } from '@angular/router'; // ... constructor(private route: ActivatedRoute) {} ngOnInit() { this.route.params.subscribe(params => { const productId = +params['id']; // Fetch product details using the productId }); }
These are the basics of Angular routing, and they are essential for building any Angular application. However, as your application grows, you’ll encounter scenarios that demand more advanced routing techniques.
2. Routing Strategies for Optimal Performance
2.1. Hash vs. Path Location Strategies
Angular supports two location strategies for routing: hash-based and path-based. The default is the path-based strategy, which uses standard URLs like example.com/products. However, in some cases, you might want to use the hash-based strategy, which adds a hash fragment to the URL, like example.com/#/products. Hash-based routing can be advantageous when deploying your application to servers with limited configuration options.
You can configure the location strategy in your app’s routing module:
typescript // app-routing.module.ts import { NgModule } from '@angular/core'; import { RouterModule, Routes, LocationStrategy, HashLocationStrategy } from '@angular/router'; // Use HashLocationStrategy for hash-based routing @NgModule({ imports: [RouterModule.forRoot(routes, { useHash: true })], exports: [RouterModule], providers: [{ provide: LocationStrategy, useClass: HashLocationStrategy }] }) export class AppRoutingModule { }
2.2. Preloading Modules for Faster Load Times
In larger Angular applications, loading all modules upfront can lead to slower initial page load times. To mitigate this, you can implement module preloading. Angular provides a preloading strategy that allows you to load specific modules lazily, after the initial page load, reducing the initial bundle size.
typescript // app-routing.module.ts import { NgModule } from '@angular/core'; import { RouterModule, Routes, PreloadAllModules } from '@angular/router'; @NgModule({ imports: [RouterModule.forRoot(routes, { preloadingStrategy: PreloadAllModules })], exports: [RouterModule] }) export class AppRoutingModule { }
2.3. Using Route Resolvers for Data Fetching
Fetching data before activating a route is a common requirement in Angular applications. Route resolvers allow you to fetch data before a route is activated and the associated component is rendered. This ensures that the component has the necessary data when it initializes.
Here’s an example of how to implement a route resolver:
typescript // product-resolver.service.ts import { Injectable } from '@angular/core'; import { Resolve, ActivatedRouteSnapshot } from '@angular/router'; @Injectable({ providedIn: 'root' }) export class ProductResolver implements Resolve<any> { constructor(private productService: ProductService) {} resolve(route: ActivatedRouteSnapshot) { const productId = route.params['id']; return this.productService.getProduct(productId); } }
And then, in your route configuration:
typescript // app-routing.module.ts const routes: Routes = [ { path: 'product/:id', component: ProductDetailComponent, resolve: { product: ProductResolver } } // Add more routes here ];
With route resolvers, you can ensure that your components have the required data, improving user experience and application performance.
3. Lazy Loading Modules
3.1. What Is Lazy Loading?
Lazy loading is a technique that allows you to load Angular modules only when they are needed, rather than including them in the initial bundle. This can significantly reduce the initial page load time, as the browser doesn’t have to download and parse code for modules that the user might not immediately use.
3.2. Implementing Lazy Loading in Angular
Lazy loading in Angular is achieved by creating feature modules and configuring your routes to load them lazily. Here’s how you can set up lazy loading:
typescript // app-routing.module.ts const routes: Routes = [ { path: 'dashboard', loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule) }, { path: 'products', loadChildren: () => import('./products/products.module').then(m => m.ProductsModule) }, // Add more lazy-loaded routes here ];
In this example, the DashboardModule and ProductsModule will be loaded lazily when the user navigates to the respective routes.
3.3. Code Splitting for Smaller Bundles
Lazy loading goes hand in hand with code splitting, a technique that breaks your application code into smaller chunks (bundles) that are loaded on-demand. This ensures that users only download the code necessary for the features they use, resulting in faster load times and improved performance.
Angular CLI supports code splitting out of the box. When you build your application, it automatically generates separate bundles for lazy-loaded modules, making it easy to optimize your app’s performance.
By implementing lazy loading and code splitting, you can significantly enhance the loading speed of your Angular application, especially as it grows in complexity.
4. Route Guards: Protecting Your Routes
4.1. Introduction to Route Guards
Route guards are used to protect routes in your application, allowing you to control access based on certain conditions. Angular provides several types of route guards:
- CanActivate: Determines whether a route can be activated.
- CanActivateChild: Similar to CanActivate but for child routes.
- CanDeactivate: Determines whether a route can be deactivated.
- CanLoad: Determines whether a lazy-loaded module can be loaded.
4.2. Creating and Implementing Route Guards
To create a route guard, you need to implement one of the guard interfaces mentioned above and provide it as part of the route configuration. Here’s an example of how to create a simple AuthGuard:
typescript // auth.guard.ts import { Injectable } from '@angular/core'; import { CanActivate, ActivatedRouteSnapshot, RouterStateSnapshot, UrlTree, Router } from '@angular/router'; import { Observable } from 'rxjs'; import { AuthService } from './auth.service'; @Injectable({ providedIn: 'root' }) export class AuthGuard implements CanActivate { constructor(private authService: AuthService, private router: Router) {} canActivate( next: ActivatedRouteSnapshot, state: RouterStateSnapshot): Observable<boolean | UrlTree> | Promise<boolean | UrlTree> | boolean | UrlTree { if (this.authService.isAuthenticated()) { return true; } else { // Redirect to the login page if not authenticated return this.router.createUrlTree(['/login']); } } }
And then, in your route configuration:
typescript // app-routing.module.ts const routes: Routes = [ { path: 'admin', component: AdminComponent, canActivate: [AuthGuard] }, // Add more guarded routes here ];
In this example, the AuthGuard checks if the user is authenticated before allowing access to the ‘admin’ route.
4.3. Combining Guards for Fine-Grained Access Control
You can combine multiple guards for fine-grained access control. For example, you might have a route that requires both authentication and specific role permissions. You can achieve this by creating and using multiple guards in your route configuration.
typescript // app-routing.module.ts const routes: Routes = [ { path: 'admin', component: AdminComponent, canActivate: [AuthGuard, RoleGuard], data: { roles: ['admin'] } }, // Add more routes with multiple guards here ];
In this example, both the AuthGuard and RoleGuard must return true for the user to access the ‘admin’ route.
5. Advanced Routing Patterns
5.1. Child Routes and Nested Navigation
Angular allows you to define child routes within a parent route. This enables nested navigation structures and can be useful for creating complex layouts or feature modules. Child routes are defined in the route configuration of the parent route.
typescript // app-routing.module.ts const routes: Routes = [ { path: 'dashboard', component: DashboardComponent, children: [ { path: 'overview', component: OverviewComponent }, { path: 'stats', component: StatsComponent }, // Add more child routes here ] }, // Add more routes with child routes here ];
In this example, the ‘dashboard’ route has child routes ‘overview’ and ‘stats,’ allowing for nested navigation.
5.2. Auxiliary Routes for Sidebar Layouts
Auxiliary routes are a powerful feature in Angular that allow you to display secondary content alongside the primary route. This is particularly useful for creating layouts with sidebars or pop-up dialogs that can be shown independently of the main content.
typescript // app-routing.module.ts const routes: Routes = [ { path: 'dashboard', component: DashboardComponent, outlet: 'sidebar' }, // Add more auxiliary routes here ];
In this example, the ‘dashboard’ route is an auxiliary route named ‘sidebar.’
5.3. Route Animations for a Polished User Experience
Adding animations to your routes can enhance the user experience and make your application feel more interactive. Angular’s built-in animation features allow you to animate route transitions seamlessly.
Here’s a simple example of how to create a fade-in animation for route transitions:
typescript // app.module.ts import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; @NgModule({ imports: [ // ... BrowserAnimationsModule ], // ... }) export class AppModule { } typescript // route-animations.ts import { transition, trigger, query, style, animate, group, } from '@angular/animations'; export const routeAnimations = trigger('routeAnimations', [ transition('* <=> *', [ query(':enter, :leave', [ style({ position: 'absolute', width: '100%', }), ]), group([ query(':enter', [ style({ opacity: 0 }), animate('0.3s ease-in-out', style({ opacity: 1 })), ]), query(':leave', [ animate('0.3s ease-in-out', style({ opacity: 0 })), ]), ]), ]), ]);
Then, apply the animation to your routes:
html <!-- app.component.html --> <router-outlet [@routeAnimations]="prepareRoute(outlet)"></router-outlet> typescript // app.component.ts @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'], animations: [routeAnimations] }) export class AppComponent { prepareRoute(outlet: RouterOutlet) { return outlet && outlet.activatedRouteData && outlet.activatedRouteData['animation']; } }
By adding animations to your routes, you can create a more engaging and visually appealing user experience.
6. Handling Route Transitions with RouteReuseStrategy
6.1. What Is Route Reuse Strategy?
Angular provides a mechanism called RouteReuseStrategy that allows you to control how Angular reuses or recreates components when navigating between routes. By default, Angular destroys and recreates components when you navigate away from a route. However, in some cases, you may want to retain the component’s state and avoid unnecessary recreation.
6.2. Customizing Route Reuse Behavior
To implement a custom RouteReuseStrategy, you need to create a class that implements the RouteReuseStrategy interface and provide it to the Angular application. Here’s an example of a simple CustomReuseStrategy:
typescript // custom-reuse-strategy.ts import { RouteReuseStrategy, ActivatedRouteSnapshot, DetachedRouteHandle } from '@angular/router'; export class CustomReuseStrategy implements RouteReuseStrategy { private handlers: { [key: string]: DetachedRouteHandle } = {}; shouldDetach(route: ActivatedRouteSnapshot): boolean { return !!route.data.reuse; } store(route: ActivatedRouteSnapshot, handle: DetachedRouteHandle | null): void { if (handle && route.data.reuse) { this.handlers[route.routeConfig?.path || ''] = handle; } } shouldAttach(route: ActivatedRouteSnapshot): boolean { return !!route.data.reuse && !!this.handlers[route.routeConfig?.path || '']; } retrieve(route: ActivatedRouteSnapshot): DetachedRouteHandle | null { if (!route.data.reuse) return null; return this.handlers[route.routeConfig?.path || '']; } shouldReuseRoute(future: ActivatedRouteSnapshot, curr: ActivatedRouteSnapshot): boolean { return future.routeConfig === curr.routeConfig; } }
Then, provide this custom strategy in your app module:
typescript // app.module.ts import { RouteReuseStrategy } from '@angular/router'; import { CustomReuseStrategy } from './custom-reuse-strategy'; @NgModule({ // ... providers: [ // ... { provide: RouteReuseStrategy, useClass: CustomReuseStrategy }, ], // ... }) export class AppModule { }
By customizing the route reuse strategy, you can optimize your application’s performance by retaining component state when navigating between routes, where appropriate.
7. Debugging and Troubleshooting Angular Routing
7.1. Common Routing Pitfalls and How to Avoid Them
While working with Angular routing, you may encounter common pitfalls that can lead to issues such as incorrect route navigation or unexpected behavior. Some of the common issues include:
- Incorrect Route Configuration: Double-check your route configurations to ensure they match the component and path you intend to use.
- Missing <router-outlet>: Make sure you have a <router-outlet> element in your template where the routed components should be rendered.
- Route Order: Routes are evaluated in the order they are defined. Ensure that more specific routes come before more generic ones to prevent unexpected route matches.
- Route Guards: Ensure your route guards return the correct values (e.g., true for access granted and false or a URL tree for access denied).
- Route Parameters: Handle route parameters correctly, and make sure they are present when needed.
- Route Data: Make use of route data to store additional information about routes, such as animation configurations or custom data needed by guards and resolvers.
7.2. Using Angular DevTools for Route Inspection
Angular DevTools is a browser extension that provides advanced debugging capabilities for Angular applications. It includes a dedicated routing tab that allows you to inspect the current route configuration, route parameters, and router state. Installing and using Angular DevTools can significantly simplify debugging routing-related issues.
7.3. Debugging Route Configuration
If you encounter issues with route configuration, you can use the Angular CLI’s ng command-line tool to generate a routing report that displays the current route configuration:
bash ng run <your-app>:route-recognizer
This report provides a visual representation of your application’s routes, making it easier to spot configuration errors.
Conclusion
Mastering advanced Angular routing techniques and patterns is essential for building robust and efficient single-page applications. In this comprehensive guide, we’ve covered a wide range of topics, from the basics of setting up routes and passing data to advanced techniques like lazy loading, route guards, and route reuse strategies.
By applying these advanced techniques and patterns, you can create Angular applications that are not only performant but also provide a seamless and polished user experience. Routing is a critical part of any web application, and with the knowledge gained from this guide, you’ll be well-equipped to tackle complex routing scenarios and build feature-rich Angular applications.
Table of Contents
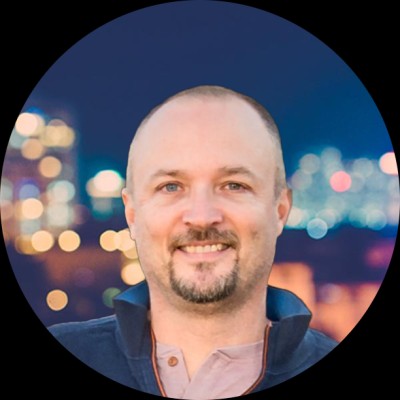
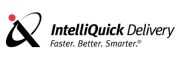