Unlocking the Power of Efficient Programming with an In-Depth Look at C#’s Data Types
Data types are a fundamental concept in programming, and a comprehensive understanding of them can provide a solid foundation for more advanced aspects of a programming language. This knowledge is especially crucial when looking to hire C# developers, as mastery of data types demonstrates their proficiency in the language. In this blog post, we’ll explore data types in C#, a widely used, general-purpose, and object-oriented programming language that skilled developers should have in their toolbox.
What is a Data Type?
In computer programming, a data type defines the kind of value a variable can hold. They specify the size and the type of data like integer, decimal, boolean, character, string, etc. A programmer must define the data type of a variable when declaring it, as this influences how the system will interpret the data.
Primitive Data Types in C#
C# offers a variety of primitive or built-in data types. These include:
- Integer types: These represent whole numbers, and they can be both positive and negative. They include byte, short, int, and long, each with varying storage capacities and value ranges. For example, an ‘int’ can store a 32-bit integer, while a ‘long’ can store a 64-bit integer.
- Floating point types: These represent real numbers (numbers with fractions). The two floating point types are ‘float’ and ‘double’. A ‘float’ occupies 32 bits of storage and a ‘double’ occupies 64 bits. The ‘double’ offers 15-16 digits of precision, while ‘float’ offers 6-7 digits.
- Decimal types: ‘decimal’ is a 128-bit data type. It’s mostly used for financial calculations where precision is paramount.
- Boolean types: ‘bool’ type represents two values, ‘true’ or ‘false’. They’re used for logical conditions.
- Character types: ‘char’ represents a single 16-bit Unicode character.
- String types: ‘string’ represents a sequence of characters. In C#, a string is an object of the String class.
Non-Primitive Data Types in C#
Non-primitive or reference data types in C# include:
- Class: This is a blueprint for creating objects. An object encapsulates data and methods that manipulate the data.
- Object: An ‘object’ is an instance of a class. It can store any kind of value.
- Array: This is a collection of elements of the same data type.
- Interface: An interface contains only the declaration of methods, properties, events, or indexers.
- Delegate: It’s a reference type variable that can hold a reference to the method.
- Dynamic: Dynamic types are resolved at runtime instead of compile-time.
Type Conversion in C#
In C#, you might sometimes need to convert one data type into another. C# supports two kinds of type conversions:
1. implicit Conversion: This conversion happens automatically when there is no risk of data loss. For example, converting an ‘int’ to a ‘long’ can be done implicitly.
```csharp int num = 100000; long bigNum = num;
2. Explicit Conversion: When there’s a risk of data loss, explicit conversion or typecasting is required. For example, converting a ‘long’ to an ‘int’ requires an explicit conversion.
```csharp long bigNum = 100000; int num = (int) bigNum;
The var Keyword
In addition to explicit data types, C# also includes a ‘var’ keyword that allows you to declare a variable without specifying an explicit data type in its declaration. The compiler then infers the type from the value on the right-hand side of the initialization statement.
```csharp var num = 5; // int var pi = 3.14; // double var message = "Hello, World!"; // string
Nullable Types
C# provides a special data type, nullable type, to hold both a defined value and an undefined value. A nullable type can represent the correct range of values for its underlying value type, plus an additional null value. Nullable types are declared by appending a “?” to the data type.
```csharp int? num = null; if(num.HasValue) { Console.WriteLine("num = " + num.Value); } else { Console.WriteLine("num = Null"); }
Conclusion
Understanding data types is crucial to effective programming in C#, a factor that’s significantly important when you are looking to hire C# developers. Mastery over data types not only allows developers to manage your data more efficiently, but it also gives a clearer understanding of how your program functions under the hood. Remember, choosing the right data type can optimize your code, reduce errors, and improve performance.
C# is a rich and versatile language, a key reason why many businesses opt to hire C# developers. It provides a variety of data types to accommodate any data needs. From primitives like integers and strings to complex types like classes and interfaces, C# offers a broad spectrum of options to help build powerful and efficient applications.
In programming, details matter, and understanding your data types is an important part of mastering the details. Happy coding!
Table of Contents
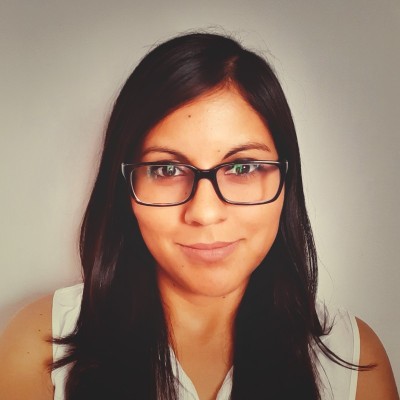
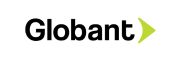