Unveiling the Core Principles of Object-Oriented Programming in C#
Programming paradigms shape the way we design, organize, and write code. Among the various programming paradigms, Object-Oriented Programming (OOP) has gained immense popularity for its clear modular structure that allows for complex applications to be built in a manageable way. This is particularly important when you’re looking to hire C# developers, as their grasp of these concepts will greatly influence the quality of the code they write.
Microsoft’s C# is a powerful language that embraces the OOP paradigm at its core, making it an excellent choice when hiring C# developers for your projects. A profound understanding of OOP in C# equips developers with the ability to write code that’s easier to read, test, and maintain.
In this blog post, we will explore the fundamentals of Object-Oriented Programming (OOP) in C#, providing you with the knowledge to effectively assess the skills of potential C# developers you aim to hire.
Understanding the Basics
At its core, Object-Oriented Programming is a method of programming that aims to simplify software development and maintenance by utilizing objects that represent real-world entities. This approach is particularly favored when you’re looking to hire C# developers, as it forms a crucial part of their skill set.
An object is an entity that encapsulates data and functions that manipulate the data. The ability to work with such objects effectively is a key competency to look for when you hire C# developers. This mastery of encapsulating data and functionality in a single object allows
developers to create code that is efficient, easy to debug, and maintainable, making it an essential consideration when hiring C# developers for your projects.
In OOP, C# allows you to define the blueprints for these objects via ‘Classes’. Classes are defined using the ‘class’ keyword and encapsulate data and methods that operate on the data.
Let’s consider a simple class definition in C#.
```csharp public class Dog { //Data members public string name; public string breed; //Member function public void Bark() { Console.WriteLine("Woof! Woof!"); } }
In this class, `name` and `breed` are data members, whereas `Bark()` is a member function. Objects can be created from this class, and each object will have its own copy of data members.
Pillars of Object-Oriented Programming
OOP in C# is underpinned by four main principles: encapsulation, inheritance, polymorphism, and abstraction. Let’s explore each one of them.
Encapsulation
Encapsulation is the mechanism of hiding data and methods within an object and making the data accessible only through the object’s methods. It enhances the security of the data. The `private` keyword is commonly used for encapsulation.
```csharp public class Dog { private string name; private string breed; public void SetName(string name) { this.name = name; } public string GetName() { return name; } }
In the example above, the `name` data member is private and can only be accessed and manipulated using the public `SetName` and `GetName` methods.
Inheritance
Inheritance allows us to define a class based on another class. This principle supports the concept of “reusability,” allowing existing classes to be reused. In C#, the `:` operator is used to inherit a class.
```csharp public class Animal { public string name; } public class Dog : Animal { public string breed; }
In the example above, the `Dog` class inherits the `Animal` class and hence has access to its public and protected members.
Polymorphism
Polymorphism allows us to use a child class object in a parent class reference with its own functionality. It provides flexibility while coding by allowing the same function to perform different tasks based on its inputs or class.
Let’s illustrate polymorphism with an example:
```csharp public class Animal { public virtual void Sound() { Console.WriteLine("The animal makes a sound"); } } public class Dog : Animal { public override void Sound() { Console.WriteLine("The dog barks"); } }
In this example, the `Dog` class overrides the `Sound` method of the `Animal` class. This allows us to make the `Dog` object perform its own `Sound` action, even when called with an `Animal` reference.
Abstraction
Abstraction refers to showing only necessary details and hiding the complexity from the user. It is accomplished in C# using abstract classes and interfaces.
```csharp public abstract class Animal { public abstract void Sound(); } public class Dog : Animal { public override void Sound() { Console.WriteLine("The dog barks"); } }
In the above example, `Animal` is an abstract class and `Sound()` is an abstract method. `Dog` class overrides the abstract `Sound` method.
More Key Concepts in C# OOP
Constructors
A constructor is a special method that is invoked when an object is created. It usually has the same name as the class and does not have a return type.
```csharp public class Dog { public string name; public string breed; //Constructor public Dog(string name, string breed) { this.name = name; this.breed = breed; } }
Properties
Properties are a way in C# to read, write, and manipulate the data of private fields. They provide a layer of security to the class’s data.
```csharp public class Dog { private string name; public string Name { get { return name; } set { name = value; } } }
Interfaces
Interfaces define a contract or behavior that classes or structs can implement. An interface can contain declarations of methods, properties, and events.
```csharp interface IAnimal { void Sound(); } public class Dog : IAnimal { public void Sound() { Console.WriteLine("The dog barks"); } }
Conclusion
The concepts we’ve discussed are fundamental to OOP in C#. Once mastered, they provide a strong foundation for tackling more complex coding challenges and building sophisticated software systems. This knowledge is invaluable when you’re looking to hire C# developers, as these principles greatly influence the effectiveness and quality of their work.
By adhering to the principles of encapsulation, inheritance, polymorphism, and abstraction, developers can create applications that are modular, easy to understand, and easy to maintain. Each object you create is like a tiny machine: it has a specific role, it knows how to interact with its environment, it can leverage its parent’s behaviors, and it can be abstracted away to create larger, more complex systems.
Understanding these principles is a mark of a competent C# developer. So, when you’re planning to hire C# developers, their grasp on these concepts will be a clear indication of their ability to deliver high-quality, efficient, and maintainable code.
Remember, the aim of OOP and C# is to improve the clarity, flexibility, and reliability of your software. Happy coding!
Table of Contents
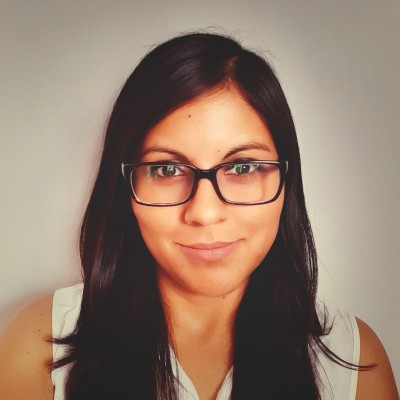
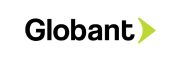