Express and Authentication: Implementing User Management and Access Control
In today’s digital landscape, security and user privacy are paramount concerns for web application developers. One of the key aspects of ensuring a secure environment is implementing user authentication and access control within your Express.js application. In this blog post, we will delve into the world of user management and access control, exploring how to integrate these essential features into your Express.js projects. We’ll cover everything from user registration and login to role-based authorization, equipping you with the tools to create a robust and secure application.
1. Introduction to User Authentication and Access Control
User authentication is the process of verifying the identity of a user, typically through a username and password, before granting access to a web application. Access control, on the other hand, involves determining what actions a user is allowed to perform within the application based on their role and permissions.
By implementing user authentication and access control, you can safeguard sensitive data, prevent unauthorized access, and tailor the user experience based on their roles. In this blog post, we will use the Express.js framework to build a sample application that demonstrates these concepts.
2. Setting Up an Express.js Application
To get started, make sure you have Node.js and npm (Node Package Manager) installed on your machine. Create a new directory for your project and initialize it with the following commands:
bash mkdir express-authentication-demo cd express-authentication-demo npm init -y
Next, install the necessary packages:
bash npm install express mongoose bcrypt jsonwebtoken express-session
Here, we’re using the Express.js framework for building our application, Mongoose for interacting with MongoDB, bcrypt for hashing passwords, jsonwebtoken for handling authentication tokens, and express-session for managing user sessions.
3. User Registration: Creating New Accounts
3.1 Creating the Registration Form
The first step in user management is allowing users to create new accounts. This involves designing a registration form where users can input their desired username, email, and password. Additionally, consider including validation checks to ensure strong passwords and unique usernames.
html <!-- registration.html --> <!DOCTYPE html> <html> <head> <title>User Registration</title> </head> <body> <h1>Register a New Account</h1> <form action="/register" method="POST"> <label for="username">Username:</label> <input type="text" id="username" name="username" required><br> <label for="email">Email:</label> <input type="email" id="email" name="email" required><br> <label for="password">Password:</label> <input type="password" id="password" name="password" required><br> <button type="submit">Register</button> </form> </body> </html>
In this form, we’re collecting the user’s username, email, and password. Remember to set up the Express route to serve this registration page and handle form submissions.
3.2 Handling Registration Requests
Create an Express route to handle the registration process. This route will receive the user’s registration data, validate it, hash the password, and store the user in the database.
javascript const express = require('express'); const bcrypt = require('bcrypt'); const User = require('./models/user'); const app = express(); app.use(express.urlencoded({ extended: true })); app.get('/register', (req, res) => { res.sendFile(__dirname + '/registration.html'); }); app.post('/register', async (req, res) => { const { username, email, password } = req.body; // Check if username is already taken const existingUser = await User.findOne({ username }); if (existingUser) { return res.status(400).send('Username already exists'); } // Hash the password const hashedPassword = await bcrypt.hash(password, 10); // Create a new user const newUser = new User({ username, email, password: hashedPassword }); await newUser.save(); res.send('Registration successful'); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this code snippet, we’re using the bcrypt library to securely hash the password before storing it in the database. This way, even if the database is compromised, the actual passwords remain confidential.
4. User Login: Granting Access to Registered Users
4.1 Building the Login Interface
Once users have registered, they should be able to log in to the application. Create a login form that accepts their credentials and submits them to the server for authentication.
html <!-- login.html --> <!DOCTYPE html> <html> <head> <title>User Login</title> </head> <body> <h1>Login to Your Account</h1> <form action="/login" method="POST"> <label for="username">Username:</label> <input type="text" id="username" name="username" required><br> <label for="password">Password:</label> <input type="password" id="password" name="password" required><br> <button type="submit">Login</button> </form> </body> </html>
As before, set up the Express routes to serve the login page and handle login requests.
5. Authenticating User Credentials
In the login route, you’ll need to authenticate the user’s credentials. Compare the entered password with the hashed password stored in the database using bcrypt’s compare function.
javascript app.get('/login', (req, res) => { res.sendFile(__dirname + '/login.html'); }); app.post('/login', async (req, res) => { const { username, password } = req.body; const user = await User.findOne({ username }); if (!user) { return res.status(401).send('Invalid username or password'); } const isPasswordValid = await bcrypt.compare(password, user.password); if (!isPasswordValid) { return res.status(401).send('Invalid username or password'); } // Create and send an authentication token const token = createAuthToken(user); res.send({ token }); });
6. Implementing Sessions and Tokens
In the above code, after successfully authenticating the user’s credentials, we generate an authentication token using jsonwebtoken. This token can be sent to the client and included in subsequent requests to authenticate the user without needing to send the username and password with each request.
javascript const jwt = require('jsonwebtoken'); const secretKey = 'your-secret-key'; function createAuthToken(user) { const payload = { userId: user._id }; return jwt.sign(payload, secretKey, { expiresIn: '1h' }); }
The token’s payload typically includes the user’s unique identifier (such as their user ID) and an expiration time. The secret key is used to sign the token and verify its authenticity.
7. Role-Based Access Control: Managing User Privileges
7.1 Defining User Roles and Permissions
In many applications, users have different roles (e.g., regular user, admin) that determine their level of access and the actions they can perform. Define roles and associated permissions in your application.
javascript const UserRoles = { USER: 'user', ADMIN: 'admin' }; const Permissions = { READ_POST: 'read_post', WRITE_POST: 'write_post' }; // User schema const userSchema = new mongoose.Schema({ username: String, email: String, password: String, role: { type: String, enum: Object.values(UserRoles), default: UserRoles.USER } }); const User = mongoose.model('User', userSchema);
In this example, we’ve defined two user roles (USER and ADMIN) and two permissions (READ_POST and WRITE_POST).
7.2 Middleware for Authorization
To enforce role-based access control, create a middleware that checks whether a user has the required role to access a certain route.
javascript function requireRole(role) { return (req, res, next) => { if (req.user && req.user.role === role) { next(); } else { res.status(403).send('Access denied'); } }; }
7.3 Protecting Routes Based on Roles
Now you can use the requireRole middleware to protect specific routes based on user roles.
javascript app.get('/admin/dashboard', requireRole(UserRoles.ADMIN), (req, res) => { // Only admins can access this route res.send('Admin dashboard'); });
This ensures that only users with the ADMIN role can access the admin dashboard route.
8. Enhancing Security with Additional Measures
8.1 Implementing Two-Factor Authentication
Two-factor authentication (2FA) adds an extra layer of security by requiring users to provide a second authentication factor, such as a code sent to their mobile device, in addition to their password.
javascript const speakeasy = require('speakeasy'); // Generate a secret for each user const userSecret = speakeasy.generateSecret(); // Store the user's secret securely (e.g., in the database) // Generate a one-time verification code const verificationCode = speakeasy.totp({ secret: userSecret.base32, encoding: 'base32' }); // Send the verification code to the user (e.g., via SMS or email)
9. Preventing Common Security Vulnerabilities
- Cross-Site Scripting (XSS): Sanitize user inputs and use libraries like helmet to set appropriate security headers.
- Cross-Site Request Forgery (CSRF): Use CSRF tokens and validate requests to prevent unauthorized actions.
- Injection Attacks: Use parameterized queries when interacting with databases to prevent SQL injection attacks.
- Sensitive Data Exposure: Encrypt sensitive data and keep API keys and passwords in secure environment variables.
Conclusion
Implementing user authentication and access control in your Express.js application is crucial for ensuring a secure and reliable user experience. By following the steps outlined in this blog post, you’ve learned how to set up user registration, authenticate users, and manage their access based on roles and permissions. Remember to stay informed about the latest security practices and regularly update your application’s security measures to protect against evolving threats. With the knowledge gained from this blog post, you are now well-equipped to build robust and secure web applications that prioritize user privacy and data integrity.
Table of Contents
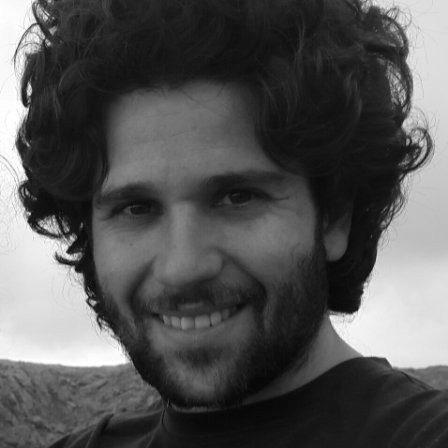
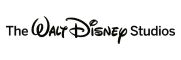