Testing Express Applications: Strategies and Tools
In the world of web development, creating a robust and reliable application is paramount. As your application grows in complexity, ensuring its functionality and stability becomes more challenging. This is where testing comes into play. In this article, we’ll delve into the strategies and tools that can help you effectively test your Express applications, guaranteeing a seamless user experience.
1. Why Test Express Applications?
Before we dive into testing strategies and tools, let’s understand why testing Express applications is crucial. Testing serves several vital purposes:
1.1. Bug Detection and Prevention
Bugs and issues can arise at any stage of development. By conducting thorough testing, you can catch and fix these problems before they reach your users, preventing potential disasters down the line.
1.2. Reliability
Users expect applications to function seamlessly. Comprehensive testing helps ensure that your application is reliable and stable, contributing to positive user experiences.
1.3. Maintainability
As your application evolves, changes in code can inadvertently introduce new bugs. Proper testing ensures that modifications to the codebase do not break existing functionality.
2. Strategies for Testing Express Applications
Effectively testing an Express application requires a well-thought-out strategy. Let’s explore some key strategies that can help you achieve comprehensive test coverage.
2.1. Unit Testing
Unit testing involves testing individual components or functions in isolation. For an Express application, this could mean testing specific routes, middleware functions, or utility modules. By isolating each piece of functionality, you can identify issues in a controlled environment.
javascript // Example of a unit test using Mocha and Chai const assert = require('chai').assert; const app = require('../app'); // Your Express app const request = require('supertest')(app); describe('GET /api/users', () => { it('should return a list of users', async () => { const response = await request.get('/api/users'); assert.equal(response.status, 200); assert.isArray(response.body); }); });
2.2. Integration Testing
Integration testing focuses on testing the interactions between different components of your application. This ensures that the various parts work together harmoniously. In the context of Express, integration testing might involve testing how routes interact with the database or how middleware functions affect the request-response cycle.
javascript // Example of an integration test using Mocha and Supertest describe('POST /api/posts', () => { it('should create a new post', async () => { const newPost = { title: 'New Post', content: 'Lorem ipsum dolor sit amet.' }; const response = await request.post('/api/posts').send(newPost); assert.equal(response.status, 201); assert.equal(response.body.title, newPost.title); }); });
2.3. End-to-End Testing
End-to-end testing simulates user interactions with your application, covering entire user journeys. Tools like Selenium, Puppeteer, or Cypress can be used to automate these tests, ensuring that the entire application works as expected.
javascript // Example of an end-to-end test using Cypress describe('User Login', () => { it('should allow a user to log in', () => { cy.visit('/login'); cy.get('[data-testid=username]').type('user123'); cy.get('[data-testid=password]').type('password123'); cy.get('[data-testid=login-button]').click(); cy.url().should('eq', 'https://example.com/dashboard'); }); });
3. Essential Tools for Testing Express Applications
To facilitate effective testing, you’ll need the right tools. Here are some essential tools that can simplify and enhance your testing process:
3.1. Mocha
Mocha is a popular testing framework that provides a clean and structured way to write tests. It supports various testing styles, including unit, integration, and asynchronous testing. With its rich ecosystem of plugins, Mocha is a versatile choice for testing Express applications.
javascript // Example of a Mocha test suite describe('Calculator', () => { it('should add two numbers', () => { assert.equal(calculator.add(2, 3), 5); }); it('should subtract two numbers', () => { assert.equal(calculator.subtract(5, 3), 2); }); });
3.2. Chai
Chai is an assertion library that pairs well with Mocha. It provides various assertion styles, allowing you to choose the one that suits your testing preferences. Chai’s fluent syntax makes your test assertions more readable and expressive.
javascript // Example of using Chai assertions in a test it('should return a valid user object', () => { const user = getUserById(1); expect(user).to.have.property('username').that.is.a('string'); expect(user).to.have.property('email').that.includes('@'); });
3.3. Supertest
Supertest is a library designed specifically for testing HTTP endpoints. It allows you to make HTTP requests to your Express app and assert the responses. This is incredibly useful for testing routes and APIs.
javascript // Example of testing an Express route using Supertest it('should return a 404 status for an invalid route', async () => { const response = await request.get('/invalid-route'); assert.equal(response.status, 404); });
3.4. Cypress
Cypress is a powerful end-to-end testing framework that provides an interactive and real-time testing experience. It allows you to visually see your tests running in a browser while performing actions like clicking buttons, filling forms, and more.
javascript // Example of a Cypress test describe('User Registration', () => { it('should allow a user to register', () => { cy.visit('/register'); cy.get('[data-testid=username]').type('newuser'); cy.get('[data-testid=email]').type('newuser@example.com'); cy.get('[data-testid=password]').type('password123'); cy.get('[data-testid=register-button]').click(); cy.url().should('eq', 'https://example.com/dashboard'); }); });
4. Best Practices for Testing Express Applications
While strategies and tools are essential, adopting best practices is equally important. Here are some guidelines to ensure effective testing of your Express applications:
4.1. Isolate Dependencies
When writing tests, aim to isolate dependencies such as databases or external services. Use techniques like mocking or stubbing to create controlled environments for your tests.
4.2. Test Edge Cases
Don’t just test the happy path. Be sure to test edge cases, boundary conditions, and error scenarios to ensure your application handles them gracefully.
4.3. Regular Test Execution
Integrate testing into your development workflow. Run your tests regularly to catch issues early and prevent regressions.
4.4. Continuous Integration
Utilize continuous integration tools like Jenkins, Travis CI, or GitHub Actions to automate test execution whenever new code is pushed to your repository.
4.5. Test Documentation
Maintain documentation for your tests. Clearly document the purpose of each test suite and the expected behavior being tested.
Conclusion
Testing is an integral part of the software development process, ensuring the reliability, functionality, and stability of your Express applications. By employing well-defined testing strategies and utilizing the right tools, you can catch bugs early, create maintainable code, and provide users with a seamless experience. Remember, effective testing requires a commitment to best practices and a mindset focused on delivering high-quality software.
Table of Contents
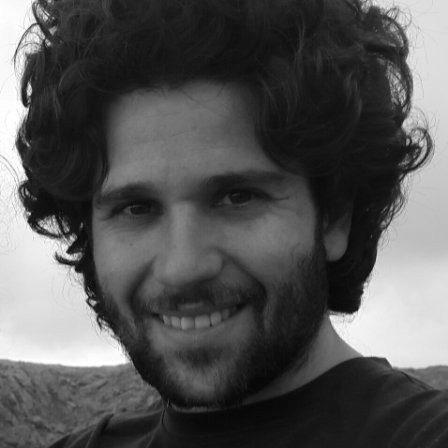
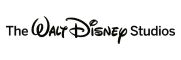