Error Handling in Express: Best Practices and Common Pitfalls
Building robust web applications requires not only writing functional code but also handling errors gracefully. In the world of Node.js and Express, effective error handling is crucial to ensure smooth user experiences and maintain the integrity of your application. In this article, we will dive into the best practices for error handling in Express and explore some common pitfalls developers should be aware of.
1. Understanding the Importance of Error Handling
1.1 Why Is Error Handling Important?
Error handling is the backbone of a reliable application. When users encounter unexpected situations or errors, their experience can quickly turn sour if the application crashes or presents cryptic error messages. Proper error handling ensures that even when things go wrong, your application remains functional and users are presented with understandable explanations.
1.2 Types of Errors in Express Applications
In Express applications, errors can be categorized into two main types: synchronous errors and asynchronous errors. Synchronous errors are those that occur during the execution of synchronous code. They are usually straightforward to handle using try-catch blocks. On the other hand, asynchronous errors occur during asynchronous operations, such as database queries or network requests. Handling asynchronous errors requires a slightly different approach.
2. Best Practices for Error Handling
2.1 Use Built-in Middleware for Errors
Express provides built-in middleware for handling errors. The express.json(), express.urlencoded(), and express.static() middlewares automatically catch errors related to JSON parsing, URL-encoded form data, and serving static files, respectively. Leveraging these built-in error-handling mechanisms can significantly reduce the chances of unexpected crashes.
javascript const express = require('express'); const app = express(); app.use(express.json()); // Handles JSON parsing errors app.use(express.urlencoded({ extended: true })); // Handles URL-encoded data errors app.use(express.static('public')); // Handles static file serving errors // ... Your routes and other middleware app.listen(3000, () => { console.log('Server is running on port 3000'); });
2.2 Centralized Error Handling
Rather than scattering error-handling code throughout your routes and middleware, it’s recommended to centralize error handling in a dedicated middleware function. This approach keeps your codebase clean and makes it easier to manage errors consistently.
javascript app.use((err, req, res, next) => { // Handle the error res.status(500).json({ error: 'Something went wrong' }); });
2.3 Proper Logging of Errors
Logging errors is essential for debugging and monitoring the health of your application. Use a logging library like Winston or Bunyan to log errors along with relevant details such as timestamps, error messages, and stack traces. Storing logs separately from your application can help you analyze errors and make improvements over time.
javascript const winston = require('winston'); const logger = winston.createLogger({ level: 'error', format: winston.format.json(), transports: [ new winston.transports.File({ filename: 'error.log', level: 'error' }), ], }); app.use((err, req, res, next) => { // Log the error logger.error(err.message, { error: err }); // Handle the error res.status(500).json({ error: 'Something went wrong' }); });
2.4 Providing Clear Error Responses
When an error occurs, it’s crucial to provide clear and informative error responses to clients. Include error codes, messages, and any relevant data that can help users and developers understand the issue. This practice enhances transparency and user trust.
javascript app.use((err, req, res, next) => { // Log the error logger.error(err.message, { error: err }); // Handle the error with a detailed response res.status(500).json({ error: 'Internal server error', code: '500-ISE' }); });
3. Common Pitfalls to Avoid
3.1 Swallowing Errors
One common mistake is to catch an error but not handle it properly, effectively “swallowing” the error. This can lead to silent failures and make it challenging to identify and fix issues. Always handle errors meaningfully or let them propagate to higher-level error handlers.
javascript // Incorrect: Swallowing the error app.get('/user/:id', (req, res, next) => { try { const user = getUserById(req.params.id); res.json(user); } catch (err) { // Swallowed error - no proper handling or logging } }); // Correct: Propagate the error app.get('/user/:id', (req, res, next) => { try { const user = getUserById(req.params.id); res.json(user); } catch (err) { next(err); // Pass the error to the error handler } });
3.2 Inadequate Status Codes
Using appropriate HTTP status codes is crucial to communicate the nature of an error. Returning a generic 200 OK status for error responses can mislead clients into thinking that everything is fine. Always use the correct status code to indicate the outcome of the request.
javascript // Incorrect: Returning a 200 status for an error app.use((err, req, res, next) => { res.status(200).json({ error: 'Something went wrong' }); }); // Correct: Returning a proper status code app.use((err, req, res, next) => { res.status(500).json({ error: 'Internal server error' }); });
3.3 Neglecting Asynchronous Error Handling
Async/await is a powerful feature in modern JavaScript, but it requires careful error handling. Neglecting to wrap asynchronous code in a try-catch block can lead to unhandled promise rejections. Always handle errors that occur within async functions to avoid potential application crashes.
javascript app.get('/data', async (req, res, next) => { try { const data = await fetchData(); res.json(data); } catch (err) { next(err); } });
4. Advanced Techniques for Error Handling
4.1 Async/Await Error Handling
When working with asynchronous operations, errors may occur within promises. To handle these errors, use try-catch blocks within async functions. This ensures that promise rejections are caught and properly handled by the error middleware.
javascript app.get('/data', async (req, res, next) => { try { const data = await fetchData(); res.json(data); } catch (err) { next(err); } });
4.2 Error Class Hierarchy
Creating a hierarchy of custom error classes can help you differentiate between different types of errors and handle them more effectively. Extend the built-in Error class to create custom error classes with additional properties and methods.
javascript class AppError extends Error { constructor(message, statusCode) { super(message); this.statusCode = statusCode; } } app.use((err, req, res, next) => { if (err instanceof AppError) { res.status(err.statusCode).json({ error: err.message }); } else { res.status(500).json({ error: 'Internal server error' }); } });
4.3 Custom Error Messages and Codes
To enhance error reporting and troubleshooting, provide custom error messages and codes. These can help developers quickly identify the source of an issue and take appropriate actions to resolve it.
javascript class AppError extends Error { constructor(message, statusCode, errorCode) { super(message); this.statusCode = statusCode; this.errorCode = errorCode; } } app.use((err, req, res, next) => { if (err instanceof AppError) { res.status(err.statusCode).json({ error: err.message, code: err.errorCode }); } else { res.status(500).json({ error: 'Internal server error', code: '500-ISE' }); } });
Conclusion
Error handling is a fundamental aspect of building robust Express applications. By following best practices such as using built-in middleware, centralizing error handling, and providing clear error responses, you can enhance the reliability and user experience of your web applications. Avoiding common pitfalls like swallowing errors, using inadequate status codes, and neglecting asynchronous error handling is equally important. As you become more proficient, consider diving into advanced techniques like async/await error handling, custom error class hierarchies, and personalized error messages and codes. Remember, a well-handled error is not just a technical necessity – it’s a part of providing exceptional user experiences.
Table of Contents
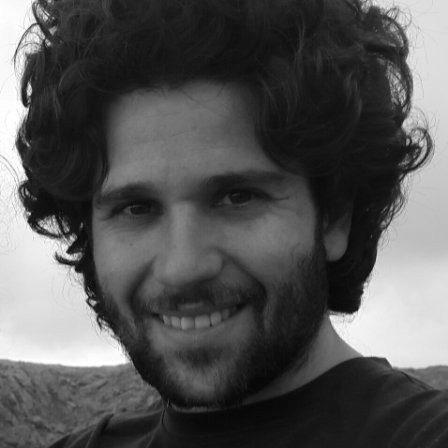
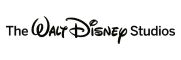