Express and GraphQL: Building Efficient and Flexible APIs
In the ever-evolving landscape of web development, APIs play a crucial role in enabling seamless communication between different components of an application. Traditional REST APIs have been widely used, but they often come with limitations in terms of flexibility and efficiency. This is where GraphQL steps in, offering a novel approach to API design that empowers developers to build efficient and flexible APIs. In this blog, we will explore how to leverage the Express framework and GraphQL to construct APIs that cater to modern development needs.
Understanding the Express Framework
Before delving into GraphQL, let’s briefly touch upon the significance of the Express framework. Express.js, a minimal and flexible Node.js web application framework, is known for its simplicity and robustness. It facilitates the creation of web applications and APIs by providing a range of utilities for handling routes, middleware, and requests. Let’s start by setting up a basic Express application:
Setting Up an Express Application
javascript const express = require('express'); const app = express(); const PORT = 3000; app.get('/', (req, res) => { res.send('Welcome to our Express API!'); }); app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
In this example, we import the express module, create an instance of the app, define a route to handle requests to the root URL, and start the server on port 3000.
The Power of GraphQL
While REST APIs have been the go-to solution for many years, they come with some inherent limitations. One of the most common issues is over-fetching or under-fetching of data, which can lead to inefficient data transfer. GraphQL addresses these problems by allowing clients to specify the exact shape and structure of the data they need. This reduces the amount of over-fetching and provides a more optimized way to retrieve and update data.
Getting Started with GraphQL
To demonstrate the capabilities of GraphQL, let’s create a simple GraphQL server using Express. We’ll use the express-graphql middleware to integrate GraphQL into our Express application.
Setting Up a GraphQL Server
javascript const express = require('express'); const { graphqlHTTP } = require('express-graphql'); const { GraphQLSchema, GraphQLObjectType, GraphQLString } = require('graphql'); const app = express(); const PORT = 3000; const RootQueryType = new GraphQLObjectType({ name: 'Query', description: 'Root Query', fields: () => ({ hello: { type: GraphQLString, resolve: () => 'Hello, GraphQL!', }, }), }); const schema = new GraphQLSchema({ query: RootQueryType, }); app.use('/graphql', graphqlHTTP({ schema: schema, graphiql: true, })); app.listen(PORT, () => { console.log(`GraphQL server is running on port ${PORT}`); });
In this example, we import the necessary GraphQL modules, define a simple schema with a RootQueryType, and set up the Express app to use the graphqlHTTP middleware. The GraphiQL interface is enabled, allowing you to explore and test the API interactively.
Creating Flexible Queries with GraphQL
One of the standout features of GraphQL is its ability to allow clients to request only the data they need. This is achieved through the flexibility of queries. Clients can specify the fields they require, and the server responds with exactly that data. Let’s take a look at how this works:
graphql { hello } When you execute this query using GraphiQL, the server responds with: json Copy code { "data": { "hello": "Hello, GraphQL!" } }
Notice that the response matches the structure of the query. This eliminates unnecessary data and enhances the efficiency of data transfer.
Efficient Data Fetching
In a real-world scenario, applications often deal with complex data structures involving multiple entities. GraphQL excels in handling such situations by allowing clients to request data from multiple sources in a single query. This concept is known as “batching.”
Consider the following example where we have a simple data structure involving users and their respective posts:
javascript const users = [ { id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, ]; const posts = [ { id: 1, userId: 1, title: 'Post by Alice' }, { id: 2, userId: 2, title: 'Post by Bob' }, ];
With GraphQL, a client can request both a user’s details and their posts in a single query:
graphql { user(id: 1) { name posts { title } } }
The server responds with:
json { "data": { "user": { "name": "Alice", "posts": [ { "title": "Post by Alice" } ] } } }
This ability to fetch related data in a single query not only simplifies the client’s work but also reduces the number of requests needed, enhancing overall performance.
Mutations for Data Manipulation
While queries are used to fetch data, mutations handle data manipulation. Mutations allow clients to perform actions like creating, updating, or deleting data. This makes GraphQL a comprehensive solution for both retrieving and modifying information.
Defining Mutations
Let’s extend our GraphQL schema to include a mutation for creating a new post:
javascript const { GraphQLObjectType, GraphQLString, GraphQLNonNull, } = require('graphql'); const RootMutationType = new GraphQLObjectType({ name: 'Mutation', description: 'Root Mutation', fields: () => ({ createPost: { type: PostType, args: { title: { type: GraphQLNonNull(GraphQLString) }, }, resolve: (parent, args) => { const newPost = { id: posts.length + 1, title: args.title }; posts.push(newPost); return newPost; }, }, }), });
In this example, we’ve added a RootMutationType with a single mutation field createPost. Clients can use this mutation to create a new post by providing the title. The server responds with the newly created post.
Executing Mutations
To test the mutation, you can use the following query in GraphiQL:
graphql mutation { createPost(title: "New Post Title") { id title } }
The server responds with:
json { "data": { "createPost": { "id": 3, "title": "New Post Title" } } }
This showcases how GraphQL simplifies the process of data manipulation while maintaining a consistent and intuitive API structure.
Middleware Integration
Express offers a wide range of middleware options that can be seamlessly integrated into your GraphQL application. Middleware functions can be used for tasks such as authentication, logging, error handling, and more. By using middleware, you can enhance the security, performance, and reliability of your GraphQL API.
Conclusion
Express and GraphQL together provide a powerful combination for building efficient and flexible APIs. While Express offers a solid foundation for web application development, GraphQL revolutionizes API design by offering a client-centric approach to data retrieval and manipulation. Its ability to optimize data transfer, fetch related data in a single query, and simplify data manipulation tasks makes it a compelling choice for modern web development.
As you embark on your journey to build APIs with Express and GraphQL, remember to explore the various features and best practices these technologies offer. By harnessing their potential, you can create APIs that not only meet the demands of today’s applications but also provide a foundation for future growth and innovation.
Table of Contents
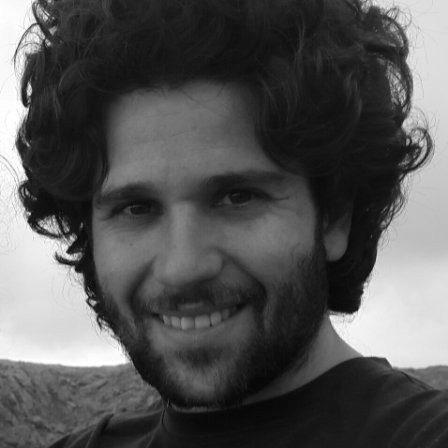
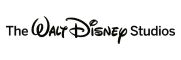