Optimizing Performance in Express: Tips for Speed and Efficiency
In today’s fast-paced digital landscape, user expectations for seamless and lightning-fast experiences have never been higher. Whether you’re building a web application, API, or a full-fledged website, optimizing the performance of your Express.js application is crucial to meeting these expectations and delivering a competitive user experience. In this guide, we’ll explore a range of strategies, techniques, and code samples that can help you achieve top-tier performance and efficiency with Express.
1. Understanding the Importance of Performance Optimization
When it comes to web applications, performance optimization is not just a nice-to-have feature; it’s a necessity. Slow-loading pages and sluggish response times can lead to user frustration, increased bounce rates, and lost revenue opportunities. On the flip side, a well-optimized Express.js application can:
- Improve user retention by delivering a satisfying user experience.
- Boost search engine rankings due to faster load times and better user engagement.
- Reduce server costs and resource usage, as efficient applications require fewer resources to handle the same load.
2. Profiling and Identifying Performance Bottlenecks
Before diving into optimization strategies, it’s crucial to understand where your application might be experiencing performance bottlenecks. Profiling tools like clinic or New Relic can help you analyze your application’s behavior and identify areas that need improvement. Key areas to look out for include:
2.1. Route Execution Time
Certain routes might be taking longer to execute than others due to complex logic or resource-intensive operations. Identify these routes and consider optimizing their code or offloading tasks to background workers.
javascript app.get('/slow-route', (req, res) => { // Heavy computations or database queries // ... res.send('Slow route executed.'); });
2.2. Database Queries
Inefficient database queries can significantly impact your application’s performance. Use tools like explain to analyze and optimize your queries for better response times.
javascript const users = await db.query('SELECT * FROM users WHERE age > 25');
2.3. Middleware
Middleware functions can add overhead to request processing. Review the middleware stack and remove any unnecessary middleware. Opt for lightweight alternatives when possible.
javascript app.use(cors()); // Consider if CORS is necessary for all routes
3. Caching for Speed
Caching is a powerful technique that can drastically reduce response times by serving precomputed or stored data instead of generating it on-the-fly. Implementing caching strategies can lead to impressive performance gains.
3.1. In-Memory Caching
Use in-memory caching with libraries like node-cache or memory-cache to store frequently accessed data, such as API responses or database query results.
javascript const cache = require('memory-cache'); app.get('/cached-route', (req, res) => { const cachedData = cache.get('cached-key'); if (cachedData) { return res.json(cachedData); } else { const newData = generateData(); cache.put('cached-key', newData, 10000); // Cache for 10 seconds return res.json(newData); } });
3.2. Content Delivery Networks (CDNs)
Offload static assets to CDNs, reducing server load and improving global access speed. This is especially effective for serving images, stylesheets, and client-side scripts.
html <script src="https://cdn.example.com/script.js"></script>
4. Gzip Compression
Compressing your responses before sending them to the client can significantly reduce data transfer time. Express provides built-in middleware for Gzip compression.
javascript const compression = require('compression'); app.use(compression());
5. Load Balancing and Scaling
As your application gains traction, you’ll need to ensure it can handle increased traffic. Load balancing and scaling strategies are essential to maintain performance under heavy loads.
5.1. Load Balancing
Distribute incoming requests across multiple servers using tools like nginx or cloud-based load balancers. This prevents any single server from becoming a bottleneck.
nginx upstream backend { server app-server1; server app-server2; } server { location / { proxy_pass http://backend; } }
5.2. Vertical Scaling
Upgrade your server’s resources (CPU, RAM) to handle increased traffic. Vertical scaling is suitable for applications with sporadic spikes in usage.
5.3. Horizontal Scaling
Add more servers to your infrastructure to distribute the load. Horizontal scaling is suitable for applications with consistently high traffic.
6. Optimizing Database Operations
Databases often play a critical role in web applications, and optimizing database operations can have a substantial impact on overall performance.
6.1. Indexing
Ensure that your database tables are properly indexed to speed up query execution times.
sql CREATE INDEX ON users (email);
6.2. Connection Pooling
Use connection pooling to efficiently manage and reuse database connections, reducing the overhead of connection establishment.
javascript const { Pool } = require('pg'); const pool = new Pool();
7. Asynchronous Code and Concurrency
JavaScript’s asynchronous nature is a double-edged sword. While it enables non-blocking I/O, it also introduces challenges related to concurrency.
7.1. Asynchronous APIs
Prefer asynchronous APIs and functions to avoid blocking the event loop and maximize your application’s responsiveness.
javascript app.get('/async-route', async (req, res) => { const result = await fetchDataFromDatabase(); res.json(result); });
7.2. Worker Threads
Offload CPU-intensive tasks to worker threads to prevent them from blocking the main thread.
javascript const { Worker, isMainThread, parentPort } = require('worker_threads'); if (isMainThread) { // Main thread logic } else { // Worker thread logic }
Conclusion
Optimizing performance in Express applications requires a combination of strategic planning, code-level enhancements, and infrastructure adjustments. By proactively identifying bottlenecks, implementing caching strategies, compressing responses, and scaling your application, you can ensure that your Express.js application delivers a smooth and efficient user experience. Stay vigilant, keep monitoring your application’s performance, and make continuous improvements to provide users with lightning-fast interactions that keep them engaged and satisfied. Your commitment to performance optimization will set your application apart in the competitive digital landscape.
Table of Contents
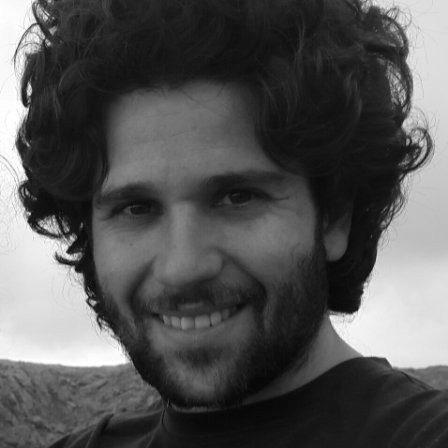
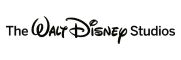