Express and React: Building Single-Page Applications
In today’s digital landscape, single-page applications (SPAs) have become increasingly popular due to their ability to provide a smooth and interactive user experience. Combining the power of a backend framework like Express.js with the frontend capabilities of React, developers can create robust SPAs that deliver a dynamic and responsive user interface. In this article, we’ll delve into the world of building SPAs using Express and React, exploring the integration of these technologies, the advantages they offer, and a step-by-step guide to building your own SPA.
Understanding Single-Page Applications:
Single-Page Applications (SPAs) are web applications that load a single HTML page and dynamically update its content as users interact with the application. Traditional multi-page applications require the browser to load new pages from the server, leading to slower user experiences. SPAs, on the other hand, use client-side scripting to update the page without requiring a full page reload. This results in faster and more responsive applications.
Benefits of SPAs:
SPAs offer several advantages, including reduced page load times, smoother navigation, and enhanced user experiences. They are particularly well-suited for applications that require real-time updates, such as social media platforms, messaging apps, and collaboration tools.
Introduction to Express.js and React:
Express.js is a popular backend framework for building web applications and APIs using Node.js. It simplifies the process of handling routes, middleware, and requests, making it an ideal choice for building the backend of SPAs. On the other hand, React is a JavaScript library for building user interfaces. Its component-based architecture and virtual DOM enable developers to create interactive and reusable frontend components.
Setting Up the Development Environment:
To get started, ensure you have Node.js and npm (Node Package Manager) installed. You can install Express.js and create an Express application using the Express Generator. Similarly, you can use Create React App to set up a React application with all the necessary configurations in place.
bash # Installing Express Generator globally npm install -g express-generator # Creating an Express application express my-express-app # Installing Create React App globally npm install -g create-react-app # Creating a React application create-react-app my-react-app
Building the Backend with Express.js:
In the Express application, define routes for different URL endpoints. Use Express middleware to handle requests, process data, and interact with databases if necessary. For instance, you can create routes to handle API requests for fetching and updating data.
javascript // Sample Express route for fetching data app.get('/api/data', (req, res) => { // Fetch data from the database or other sources const data = fetchData(); res.json(data); });
Developing the Frontend with React:
In the React application, create components that represent different parts of your UI. Use React state and props to manage data and pass information between components. Fetch data from the Express backend using JavaScript’s fetch or third-party libraries like axios.
jsx // Sample React component fetching data from Express API import React, { useState, useEffect } from 'react'; function App() { const [data, setData] = useState([]); useEffect(() => { fetch('/api/data') .then(response => response.json()) .then(data => setData(data)); }, []); return ( <div> {data.map(item => ( <div key={item.id}>{item.name}</div> ))} </div> ); } export default App;
Integrating Express.js and React:
To connect the Express backend and React frontend, you need to handle CORS (Cross-Origin Resource Sharing) to allow communication between different origins. You can also use a proxy configuration in the React app to forward API requests to the Express server during development.
json // React app's package.json for proxying requests { // ... "proxy": "http://localhost:3001" }
Creating a Dynamic User Experience:
Implement client-side routing using libraries like react-router to enable navigation without reloading the entire page. Handle form submissions using React state and event handlers. For real-time updates, consider integrating WebSockets using libraries like socket.io.
Optimizing Performance and SEO:
Improve performance by code splitting, which allows loading only the required JavaScript for a specific route. For enhanced SEO, consider server-side rendering (SSR) using technologies like Next.js with Express. Implement SEO-friendly metadata such as title and meta description tags to ensure your SPA is discoverable by search engines.
Deploying the SPA:
Build production-ready code for both the Express backend and the React frontend. Deploy the Express app to a hosting service that supports Node.js applications. For the React app, you can deploy it to platforms like Netlify, Vercel, or GitHub Pages.
Conclusion
Building single-page applications with Express.js and React empowers developers to create dynamic, interactive, and responsive web experiences. The combination of a powerful backend framework and a flexible frontend library allows for seamless communication between server and client, resulting in efficient and engaging user interfaces. By understanding the integration process and following best practices, you can successfully create and deploy SPAs that cater to modern web development demands. So, dive into the world of Express.js and React, and unlock the potential to build exceptional single-page applications.
Table of Contents
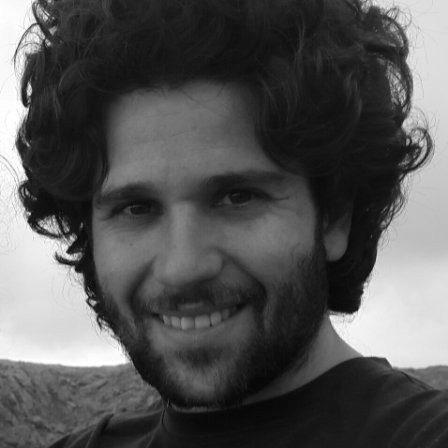
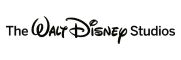