Creating Real-Time Applications with Express and Socket.IO
In today’s fast-paced digital world, real-time applications have become increasingly popular. From chat applications to collaborative tools, real-time communication plays a vital role in enhancing user experience. In this blog, we will explore how to create real-time applications using Express, a popular web application framework for Node.js, and Socket.IO, a JavaScript library that enables real-time, bidirectional communication between the server and the client. We will dive into the concepts and provide you with code samples to get you started on building your own real-time applications.
What are Real-Time Applications?
Real-time applications are those that require instant data transmission and updates between the client and the server. These applications enable seamless communication, collaboration, and interaction between multiple users. Examples include chat applications, multiplayer games, collaborative document editors, and stock market tickers.
Introduction to Express
Express is a minimalistic and flexible web application framework for Node.js. It provides a robust set of features for building web applications and APIs. With its simplicity and rich ecosystem, Express is widely adopted for creating web applications of all scales. Its middleware architecture makes it easy to extend and customize functionality according to specific requirements.
Code Sample: Installing Express
ruby $ npm install express
Introduction to Socket.IO
Socket.IO is a JavaScript library that enables real-time, bidirectional communication between the server and the client. It abstracts the complexities of the WebSocket protocol and provides a simple API for event-based communication. Socket.IO is built on top of WebSockets but gracefully falls back to other techniques such as long-polling when WebSocket support is lacking.
Code Sample: Installing Socket.IO
lua $ npm install socket.io
Setting Up the Project
To begin building our real-time application, let’s set up a new Express project and install the required dependencies.
Code Sample: Setting Up the Express Project
javascript const express = require('express'); const app = express(); const server = app.listen(3000, () => { console.log('Server listening on port 3000'); });
Creating Real-Time Communication
To establish real-time communication with Socket.IO, we need to integrate it into our Express application. Let’s modify our previous code to include Socket.IO and handle a basic connection event.
Code Sample: Integrating Socket.IO with Express
javascript const express = require('express'); const app = express(); const http = require('http').createServer(app); const io = require('socket.io')(http); io.on('connection', (socket) => { console.log('A user connected'); }); const server = http.listen(3000, () => { console.log('Server listening on port 3000'); });
Broadcasting and Receiving Messages
Socket.IO enables us to broadcast messages to connected clients or handle messages received from clients. Let’s enhance our code to demonstrate how to send and receive messages in real-time.
Code Sample: Broadcasting and Receiving Messages
javascript io.on('connection', (socket) => { console.log('A user connected'); socket.on('chat message', (msg) => { console.log('Received message:', msg); io.emit('chat message', msg); }); });
Handling Client Disconnection
It’s essential to handle client disconnections gracefully to ensure the stability of our real-time application. Socket.IO provides an event to detect when a client disconnects.
Code Sample: Handling Client Disconnection
javascript io.on('connection', (socket) => { console.log('A user connected'); socket.on('disconnect', () => { console.log('A user disconnected'); }); });
Scaling Real-Time Applications
As the number of users and complexity of your real-time application grows, you may need to scale it to handle the load. One approach is to use a load balancer to distribute client requests among multiple instances of your application. Socket.IO supports Redis or other adapters to enable communication between multiple instances.
Conclusion
Express and Socket.IO are powerful tools for building real-time applications. With Express, you can create robust web applications, and Socket.IO provides seamless bidirectional communication between the server and the client. By understanding the concepts discussed in this blog and utilizing the code samples provided, you can start creating your own real-time applications and deliver dynamic, interactive experiences to your users.
In this blog, we have explored the basics of Express and Socket.IO and learned how to integrate them to create real-time applications. We have covered topics such as setting up the project, establishing real-time communication, broadcasting and receiving messages, handling client disconnections, and scaling real-time applications. Now, armed with this knowledge, you can venture into the world of real-time applications and build your own exciting projects. Happy coding!
Table of Contents
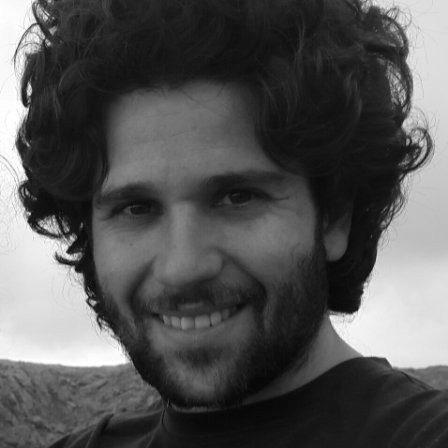
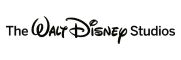