Building RESTful APIs with Express: Design Principles and Examples
In today’s interconnected digital world, building robust and efficient APIs is essential for enabling seamless communication between various applications. Representational State Transfer (REST) has emerged as a popular architectural style for designing networked applications. Express.js, a powerful Node.js framework, provides developers with the tools needed to create RESTful APIs that adhere to REST principles. In this article, we’ll delve into the design principles of building RESTful APIs using Express and provide practical examples to illustrate each concept.
1. Understanding REST and API Design:
REST, short for Representational State Transfer, is an architectural style that defines a set of constraints for creating scalable, maintainable, and adaptable networked applications. APIs built following REST principles are known as RESTful APIs. Here are the key design principles of RESTful APIs:
1.1 Client-Server Architecture:
The client-server architecture separates the client’s concerns from the server’s concerns. This separation allows for independent development, easier scaling, and improved portability.
1.2 Statelessness:
Each request from a client to the server must contain all the information necessary to understand and process the request. The server doesn’t store any client state between requests, making the system more resilient and scalable.
1.3 Cacheability:
Responses from the server can be marked as cacheable or non-cacheable. Caching enhances performance and reduces the need for repeated requests to the server.
1.4 Uniform Interface:
This principle defines a uniform way to interact with resources. Resources are identified by URLs, and interactions are performed using standard HTTP methods like GET, POST, PUT, and DELETE.
1.5 Layered System:
A layered system architecture allows for the addition of intermediary servers (proxies, gateways) to improve security, load balancing, or caching, without affecting the client-server interaction.
1.6 Code on Demand (Optional):
While not always used, this principle allows the server to send executable code (e.g., JavaScript) to the client for client-side execution, extending the functionality of the client.
2. Setting Up Express.js:
Before we dive into designing RESTful APIs, let’s set up a basic Express.js application. Ensure you have Node.js and npm (Node Package Manager) installed.
2.1 Initializing a Project:
Create a new directory for your project and navigate to it in the terminal. Run the following commands to initialize a new Node.js project and install Express:
bash npm init -y npm install express
2.2 Creating the Server:
Create a file named app.js (or any other name you prefer) in your project directory. Add the following code to set up a basic Express server:
javascript const express = require('express'); const app = express(); const port = 3000; app.listen(port, () => { console.log(`Server is listening at http://localhost:${port}`); });
You can start the server using the command node app.js.
3. Designing RESTful APIs with Express:
Now that our basic Express server is up and running, let’s start designing RESTful APIs using the Express framework. We’ll cover the main HTTP methods (GET, POST, PUT, DELETE) and their corresponding route handlers.
3.1 Creating Resources (POST):
To create a new resource, such as a blog post, we’ll use the POST method. Let’s create a route to handle creating new blog posts:
javascript app.post('/posts', (req, res) => { // Logic to create a new blog post res.status(201).json({ message: 'Blog post created successfully' }); });
In this example, when a POST request is sent to /posts, the server responds with a JSON message indicating the successful creation of a blog post.
3.2 Retrieving Resources (GET):
The GET method is used to retrieve resources. Let’s create a route to get a list of all blog posts:
javascript app.get('/posts', (req, res) => { // Logic to retrieve a list of blog posts const posts = [...]; // Array of blog posts res.status(200).json(posts); });
Here, the server responds with a JSON array containing all the blog posts.
3.3 Updating Resources (PUT):
To update a resource, we’ll use the PUT method. Let’s create a route to update an existing blog post:
javascript app.put('/posts/:postId', (req, res) => { const postId = req.params.postId; // Logic to update the blog post with postId res.status(200).json({ message: 'Blog post updated successfully' }); });
In this route, :postId is a route parameter that allows us to identify the specific blog post to update.
3.4 Deleting Resources (DELETE):
The DELETE method is used to remove resources. Let’s create a route to delete a blog post:
javascript app.delete('/posts/:postId', (req, res) => { const postId = req.params.postId; // Logic to delete the blog post with postId res.status(204).send(); });
In this example, a successful deletion results in a status code 204 (No Content).
4. Handling Errors and Validation:
Error handling and data validation are crucial aspects of building reliable APIs. Express provides middleware functions to handle these aspects effectively.
4.1 Error Handling Middleware:
Create an error-handling middleware to catch and handle errors that occur during request processing:
javascript app.use((err, req, res, next) => { console.error(err.stack); res.status(500).json({ message: 'Internal Server Error' }); });
This middleware catches errors and responds with an appropriate error message and status code.
4.2 Data Validation with Middleware:
Use middleware to validate incoming data before processing it. For instance, the express-validator library can be used for data validation:
javascript const { body, validationResult } = require('express-validator'); app.post('/posts', body('title').notEmpty().isString(), body('content').notEmpty().isString(), (req, res) => { const errors = validationResult(req); if (!errors.isEmpty()) { return res.status(400).json({ errors: errors.array() }); } // Logic to create a new blog post res.status(201).json({ message: 'Blog post created successfully' }); });
In this example, the express-validator middleware ensures that the title and content fields are not empty and are strings before processing the request.
Conclusion
Building RESTful APIs using Express.js involves adhering to REST principles and using the HTTP methods effectively. By designing resources, handling various HTTP methods, and incorporating error handling and validation, developers can create efficient and reliable APIs that facilitate seamless communication between applications. Express’s flexibility and simplicity make it a powerful tool for crafting RESTful APIs that cater to modern software development needs. As you embark on your journey to build APIs, remember to prioritize scalability, maintainability, and adherence to REST principles for a successful and future-proof application architecture.
Table of Contents
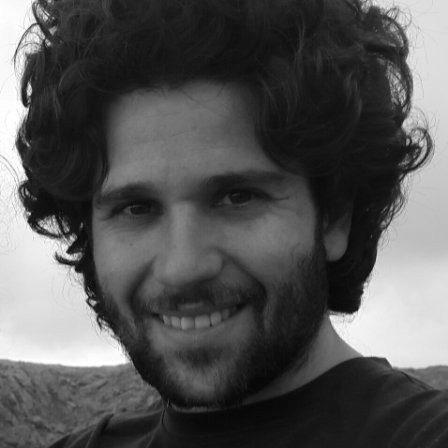
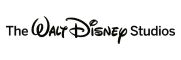