Exploring Express Routing: Handling Requests and Building APIs
In the world of web development, building efficient and scalable APIs is a crucial task. The Express.js framework has emerged as a go-to choice for many developers due to its simplicity and flexibility in creating server-side applications. At the heart of Express lies its powerful routing system, which forms the backbone of any API-driven application. In this blog, we’ll dive deep into Express routing, uncovering its intricacies and showcasing how to handle requests and construct APIs effectively.
Understanding Routing in Express
Routing in Express refers to the process of determining how an application responds to a client request for a particular endpoint (URL) with a specific HTTP request method (e.g., GET, POST, PUT, DELETE). Express makes this process incredibly intuitive by providing a routing mechanism that allows you to define routes, associate middleware functions, and handle requests with precision.
Basic Routing
Let’s start by looking at the fundamentals of routing in Express. At its core, routing involves defining a route using the app object (an instance of express()) and specifying an HTTP method. Here’s an example:
javascript const express = require('express'); const app = express(); app.get('/', (req, res) => { res.send('Hello, Express!'); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this example, we’ve defined a route for the root URL (‘/’) using the app.get() method. When a GET request is made to the root URL, the callback function (req, res) is executed, sending back the response ‘Hello, Express!’. This is the basic structure of an Express route.
Route Parameters
Route parameters allow us to capture values from the URL and use them in our application logic. This is particularly useful when building dynamic endpoints, such as user profiles or product pages. Let’s see how route parameters work:
javascript app.get('/users/:userId', (req, res) => { const userId = req.params.userId; res.send(`Fetching data for user with ID: ${userId}`); });
In this example, we’ve defined a route with a parameter :userId. When a request is made to a URL like /users/123, the parameter value 123 is captured and accessible via req.params.userId.
Middleware in Routing
Middleware functions are an essential part of Express routing. They are functions that have access to the request (req) and response (res) objects and can perform tasks before the final request handler is executed. Middleware functions are useful for tasks like authentication, validation, logging, and more.
javascript const logMiddleware = (req, res, next) => { console.log(`[${new Date().toISOString()}] ${req.method} ${req.url}`); next(); // Call next to move to the next middleware or route handler }; app.use(logMiddleware); app.get('/secure', (req, res) => { res.send('This is a secure route'); });
In this example, the logMiddleware function logs the timestamp, HTTP method, and URL of each incoming request. The next() function call passes control to the next middleware or route handler.
Building APIs with Express
Now that we have a solid understanding of the basics, let’s explore how Express routing can be leveraged to build robust APIs. APIs consist of multiple endpoints that serve different purposes. Express makes it easy to organize these endpoints and apply specific logic to each one.
API Versioning
As APIs evolve, it’s crucial to maintain backward compatibility while introducing new features. API versioning allows you to achieve this by including version information in the URL. Here’s an example of versioned routing:
javascript const v1Router = express.Router(); const v2Router = express.Router(); v1Router.get('/users', (req, res) => { res.send('API v1: List of users'); }); v2Router.get('/users', (req, res) => { res.send('API v2: List of users with enhanced data'); }); app.use('/v1', v1Router); app.use('/v2', v2Router);
In this scenario, requests to /v1/users and /v2/users will be routed to different handlers, allowing you to maintain separate implementations for different API versions.
Error Handling
Robust error handling is a critical aspect of any API. Express provides middleware to handle errors and respond with appropriate status codes and error messages. You can define a custom error handler as follows:
javascript const errorHandler = (err, req, res, next) => { console.error(err.stack); res.status(500).send('Something went wrong!'); }; app.use(errorHandler);
By placing this middleware at the end of your routes, any errors that occur in previous middleware or route handlers will be caught and processed by the errorHandler.
CRUD Operations with Express
RESTful APIs often involve CRUD (Create, Read, Update, Delete) operations. Express makes implementing these operations straightforward through its routing system.
javascript const database = []; // Placeholder for demonstration app.get('/items', (req, res) => { res.json(database); }); app.post('/items', (req, res) => { const newItem = req.body; database.push(newItem); res.status(201).json(newItem); }); app.put('/items/:id', (req, res) => { const itemId = req.params.id; const updatedItem = req.body; // Logic to update item in the database res.json(updatedItem); }); app.delete('/items/:id', (req, res) => { const itemId = req.params.id; // Logic to delete item from the database res.sendStatus(204); });
In this example, we’ve implemented the CRUD operations using different HTTP methods and route handlers. Remember that these handlers should interact with a database or data source to perform the actual operations.
Conclusion
Express routing is at the core of building efficient and reliable server-side applications. From handling basic routes to constructing complex APIs, the flexibility and simplicity of Express make it a powerful tool for web developers. Understanding the concepts of routing, middleware, and API design principles empowers you to create scalable and maintainable applications that serve as the backbone of modern web services. As you continue your journey with Express, keep exploring its features and best practices to craft exceptional APIs and deliver seamless experiences to your users.
Table of Contents
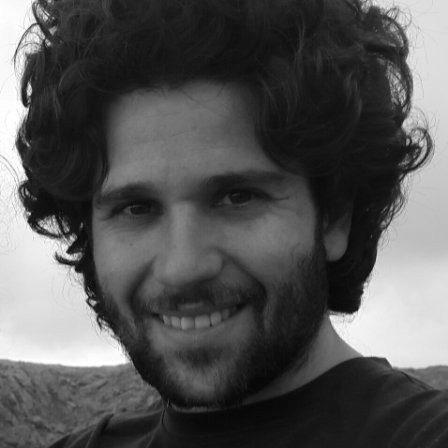
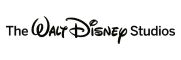