Building a Fitness Tracking App with Flutter: Sensors and Health Data
In today’s fast-paced world, staying fit and healthy is a priority for many. With the rise of smartphones and wearable devices, fitness tracking apps have become an integral part of people’s wellness journeys. These apps not only help individuals monitor their physical activities but also provide valuable insights into their overall health. If you’re an aspiring app developer looking to create a fitness tracking app, this guide will walk you through the process using Flutter, Google’s open-source UI framework.
Table of Contents
1. Why Flutter?
Flutter is an excellent choice for building cross-platform mobile apps that deliver a native-like experience. It allows developers to create a single codebase that can run on both iOS and Android platforms, reducing development time and effort. Flutter’s rich set of customizable widgets and its fast performance make it an ideal framework for crafting visually appealing fitness tracking apps.
2. Setting Up the Project
Before we dive into the specifics of sensors and health data integration, let’s set up our Flutter project:
Install Flutter: If you haven’t already, install Flutter by following the instructions on the official Flutter website.
Create a New Flutter Project: Use the following command to create a new Flutter project:
bash flutter create fitness_tracking_app
Dependencies: In your pubspec.yaml file, add the necessary dependencies:
yaml dependencies: flutter: sdk: flutter health: ^5.0.0 sensors: ^0.7.0
Run flutter pub get to install the dependencies.
3. Integrating Sensors for Data Collection
A key feature of any fitness tracking app is the ability to collect data from various sensors present in the user’s device. Flutter provides a package called sensors that allows you to access data from sensors such as accelerometers, gyroscopes, and more. Here’s how you can integrate sensor data into your fitness tracking app:
3.1. Import the Package
In your Dart file, import the sensors package:
dart import 'package:sensors/sensors.dart';
3.2. Access Sensor Data
Let’s say you want to access accelerometer data. Here’s how you can do it:
dart StreamSubscription<AccelerometerEvent> accelerometerSubscription; accelerometerSubscription = accelerometerEvents.listen((AccelerometerEvent event) { // Access accelerometer data double x = event.x; double y = event.y; double z = event.z; }); // Don't forget to cancel the subscription when it's no longer needed accelerometerSubscription.cancel();
Similarly, you can access data from other sensors like gyroscope and magnetometer.
4. Managing Health Data
Incorporating health data into your fitness tracking app is essential for providing users with a holistic view of their well-being. The health package in Flutter allows you to retrieve health-related information from the device with the user’s consent.
4.1. Import the Package
Start by importing the health package:
dart import 'package:health/health.dart';
4.2. Requesting Data Permissions
Before accessing health data, you need to request the necessary permissions from the user:
dart Future<void> requestPermission() async { final HealthFactory health = HealthFactory(); bool granted = await health.requestAuthorization(); if (granted) { // Permission granted, you can now fetch health data } else { // Permission denied, handle accordingly } }
4.3. Fetching Health Data
Once you have the necessary permissions, you can fetch health data such as step count, heart rate, and more:
dart Future<void> fetchHealthData() async { final HealthFactory health = HealthFactory(); List<HealthDataType> types = [ HealthDataType.STEPS, HealthDataType.HEART_RATE, // Add more data types as needed ]; DateTime now = DateTime.now(); DateTime start = DateTime(now.year, now.month, now.day - 7); DateTime end = now; try { List<HealthDataPoint> healthData = await health.getHealthDataFromTypes(types, start, end); // Process and display the health data } catch (e) { // Handle errors } }
5. Designing the User Interface
A visually appealing and user-friendly interface is crucial for a fitness tracking app. Flutter’s widget-based approach makes it convenient to create beautiful UI elements. Here’s a simple example of designing a step count display:
dart import 'package:flutter/material.dart'; class StepCountDisplay extends StatelessWidget { final int stepCount; StepCountDisplay(this.stepCount); @override Widget build(BuildContext context) { return Container( padding: EdgeInsets.all(16.0), decoration: BoxDecoration( color: Colors.blue, borderRadius: BorderRadius.circular(10.0), ), child: Column( children: [ Icon(Icons.directions_walk, color: Colors.white, size: 48.0), SizedBox(height: 8.0), Text( '$stepCount Steps', style: TextStyle(color: Colors.white, fontSize: 24.0), ), ], ), ); } }
Conclusion
In this tutorial, we explored the process of building a fitness tracking app using Flutter. We covered sensor integration to gather data such as accelerometer readings and discussed how to incorporate health data like step counts and heart rates. Remember that this is just a starting point, and there’s a world of possibilities to explore in terms of features and functionalities. As you continue your journey in app development, keep exploring Flutter’s capabilities and the numerous packages it offers to enhance your app even further. So go ahead, start coding, and contribute to the fitness and wellness of users worldwide!
Table of Contents
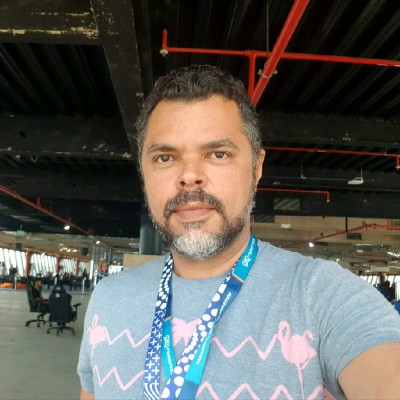
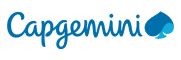