Building a Recipe App with Flutter: Data Management and Search
In the ever-evolving world of mobile app development, Flutter has emerged as a powerful and versatile framework for building high-quality applications across platforms. Its flexibility and ease of use make it an excellent choice for developing a Recipe App, which requires seamless data management and efficient search functionality. In this comprehensive guide, we will walk you through the process of creating a Recipe App with Flutter, emphasizing data management and search features.
Table of Contents
1. Prerequisites
Before diving into the development process, make sure you have the following prerequisites in place:
1.1. Flutter Installed
Ensure that you have Flutter installed on your system. You can download and install it by following the official documentation: Flutter Installation Guide.
1.2. Development Environment
Set up your preferred development environment, whether it’s Android Studio, Visual Studio Code, or any other IDE that supports Flutter development. Make sure you have all the necessary plugins and extensions installed for a smooth coding experience.
2. Creating a New Flutter Project
Let’s kick off our Recipe App development journey by creating a new Flutter project. Open your terminal and run the following command:
bash flutter create recipe_app
This command will generate a new Flutter project named “recipe_app.” Navigate to the project directory:
bash cd recipe_app
Now that we have our project set up, it’s time to focus on data management.
3. Data Management with Firebase
To build a Recipe App that stores and retrieves recipes, we’ll use Firebase as our backend service. Firebase offers real-time database capabilities and authentication services, making it an ideal choice for this type of application. Follow these steps to set up Firebase in your project:
3.1. Create a Firebase Project
Go to the Firebase Console and create a new project. Follow the on-screen instructions to set it up.
3.2. Add Firebase to Your Flutter App
In your Flutter project, open the pubspec.yaml file and add the Firebase dependencies:
yaml dependencies: flutter: sdk: flutter firebase_core: ^latest_version firebase_auth: ^latest_version cloud_firestore: ^latest_version
Run flutter pub get to fetch the new dependencies.
3.3. Configure Firebase in Your App
Initialize Firebase in your Flutter app by adding the following code in your main.dart:
dart import 'package:firebase_core/firebase_core.dart'; void main() async { WidgetsFlutterBinding.ensureInitialized(); await Firebase.initializeApp(); runApp(MyApp()); }
Now, Firebase is integrated into your Flutter app, and you can start using Firebase services, such as Firestore, for data management.
4. Designing the Data Model
Before we start implementing data management, let’s design the data model for our Recipe App. In this example, we’ll create a simple recipe model with properties like title, description, ingredients, and instructions. Create a new Dart file, recipe_model.dart, and define the Recipe class:
dart class Recipe { final String id; final String title; final String description; final List<String> ingredients; final List<String> instructions; Recipe({ required this.id, required this.title, required this.description, required this.ingredients, required this.instructions, }); }
Now that we have our data model in place, let’s move on to data management.
5. Data Management with Firestore
Firestore is Firebase’s real-time database service, which we’ll use to store and manage our recipe data. First, initialize Firestore in your Flutter app:
dart import 'package:cloud_firestore/cloud_firestore.dart';
final FirebaseFirestore _firestore = FirebaseFirestore.instance;
5.1. Adding Recipes
To add a new recipe to Firestore, create a function like this:
dart Future<void> addRecipe(Recipe recipe) async { try { await _firestore.collection('recipes').add({ 'title': recipe.title, 'description': recipe.description, 'ingredients': recipe.ingredients, 'instructions': recipe.instructions, }); } catch (e) { print('Error adding recipe: $e'); } }
5.2. Fetching Recipes
To retrieve recipes from Firestore, create a function like this:
dart Stream<List<Recipe>> getRecipes() { return _firestore.collection('recipes').snapshots().map((snapshot) { return snapshot.docs.map((doc) { final data = doc.data() as Map<String, dynamic>; return Recipe( id: doc.id, title: data['title'] ?? '', description: data['description'] ?? '', ingredients: List<String>.from(data['ingredients'] ?? []), instructions: List<String>.from(data['instructions'] ?? []), ); }).toList(); }); }
6. Building the Recipe List
With data management in place, it’s time to create the Recipe List screen. We’ll use a ListView.builder to display the list of recipes. Create a new file, recipe_list.dart, and build the UI:
dart import 'package:flutter/material.dart'; import 'package:your_recipe_app/recipe_model.dart'; class RecipeList extends StatelessWidget { final Stream<List<Recipe>> recipes; RecipeList({required this.recipes}); @override Widget build(BuildContext context) { return StreamBuilder<List<Recipe>>( stream: recipes, builder: (context, snapshot) { if (!snapshot.hasData) { return CircularProgressIndicator(); } final recipeList = snapshot.data!; return ListView.builder( itemCount: recipeList.length, itemBuilder: (context, index) { final recipe = recipeList[index]; return ListTile( title: Text(recipe.title), subtitle: Text(recipe.description), onTap: () { // Navigate to the recipe detail page }, ); }, ); }, ); } }
In this code, we create a RecipeList widget that takes a Stream of recipes as a parameter. It listens to changes in the recipe data and updates the UI accordingly.
7. Implementing Recipe Detail View
To view the details of a recipe, we need to create a Recipe Detail screen. Create a new file, recipe_detail.dart, and build the UI:
dart import 'package:flutter/material.dart'; import 'package:your_recipe_app/recipe_model.dart'; class RecipeDetail extends StatelessWidget { final Recipe recipe; RecipeDetail({required this.recipe}); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(recipe.title), ), body: SingleChildScrollView( padding: EdgeInsets.all(16.0), child: Column( crossAxisAlignment: CrossAxisAlignment.start, children: [ Text( recipe.description, style: TextStyle(fontSize: 18.0), ), SizedBox(height: 16.0), Text( 'Ingredients:', style: TextStyle(fontSize: 16.0, fontWeight: FontWeight.bold), ), Column( crossAxisAlignment: CrossAxisAlignment.start, children: recipe.ingredients .map((ingredient) => Text('- $ingredient')) .toList(), ), SizedBox(height: 16.0), Text( 'Instructions:', style: TextStyle(fontSize: 16.0, fontWeight: FontWeight.bold), ), Column( crossAxisAlignment: CrossAxisAlignment.start, children: recipe.instructions .asMap() .entries .map((entry) => Text('${entry.key + 1}. ${entry.value}')) .toList(), ), ], ), ), ); } }
This code defines the RecipeDetail widget, which displays the recipe’s title, description, ingredients, and instructions in a detailed view.
8. Implementing Recipe Search
Now that we have the Recipe List and Recipe Detail views ready, let’s add a search functionality to our Recipe App. We’ll implement a simple search bar at the top of the Recipe List screen.
8.1. Adding a Search Bar
In the RecipeList widget, add a TextField at the top for searching recipes:
dart TextField( onChanged: (query) { // Handle search query }, decoration: InputDecoration( hintText: 'Search recipes', prefixIcon: Icon(Icons.search), border: OutlineInputBorder( borderRadius: BorderRadius.circular(10.0), ), ), ),
8.2. Implementing Recipe Search Logic
To implement the recipe search logic, update the onChanged callback of the TextField:
dart Stream<List<Recipe>> _searchRecipes(String query) { return recipes.map((recipeList) { return recipeList.where((recipe) { final title = recipe.title.toLowerCase(); final description = recipe.description.toLowerCase(); return title.contains(query.toLowerCase()) || description.contains(query.toLowerCase()); }).toList(); }); } TextField( onChanged: (query) { final searchedRecipes = _searchRecipes(query); // Update the UI with searchedRecipes }, // ... ),
Now, as the user types in the search bar, the TextField calls _searchRecipes(query) to filter the recipes based on the query.
Conclusion
Congratulations! You’ve learned how to build a Recipe App with Flutter, focusing on data management and search functionality. We covered setting up Firebase for data storage, designing a data model, managing data with Firestore, building the Recipe List and Recipe Detail views, and implementing recipe search.
This is just the beginning of your Flutter journey. You can further enhance your app by adding features like user authentication, recipe ratings, and more. Flutter’s flexibility and extensive ecosystem make it a powerful tool for creating feature-rich mobile applications.
Happy coding, and enjoy building your Recipe App with Flutter!
Table of Contents
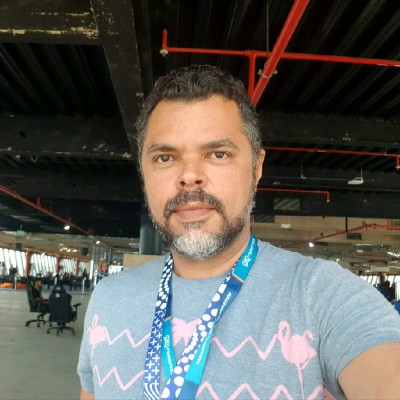
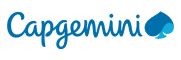