Building a Fitness Tracking App with Flutter: Sensors and Health Data
In today’s fast-paced world, staying fit and healthy is a top priority for many. With the advent of smartphones and wearable devices, fitness tracking apps have become essential tools for monitoring and improving one’s health.
Table of Contents
Fitness tracking apps have revolutionized the way people monitor their health and wellness. These apps not only help users keep track of their physical activity but also provide valuable insights into their overall well-being. To create a feature-rich fitness tracking app, we will leverage the power of Flutter and its ability to create beautiful and performant cross-platform applications.
In this tutorial, we’ll cover the entire process of building a fitness tracking app, starting from setting up the development environment to accessing sensors data, integrating health data, and storing and analyzing fitness data. By the end of this guide, you’ll have the knowledge and skills to develop your fitness tracking app or enhance an existing one.
1. Setting Up the Flutter Environment
Before we dive into building the app, let’s ensure you have Flutter set up on your development machine. Follow these steps to get started:
Step 1: Install Flutter
Visit the official Flutter installation guide for detailed instructions on installing Flutter on your operating system.
Step 2: Verify Installation
Open a terminal and run the following command to verify your installation:
bash flutter doctor
This command will check if you have all the necessary dependencies installed and provide guidance on resolving any issues.
Step 3: Create a New Flutter Project
Now that you have Flutter installed, create a new Flutter project using the following command:
bash flutter create fitness_tracking_app
Navigate to the project directory:
bash cd fitness_tracking_app
You’re now ready to start building the app!
2. Creating the User Interface
The user interface is a critical component of any fitness tracking app. It should be intuitive, visually appealing, and easy to navigate. Flutter makes it straightforward to create a beautiful UI with its rich set of widgets.
Here’s a sample code snippet to create a simple home screen for your fitness tracking app:
dart import 'package:flutter/material.dart'; void main() { runApp(FitnessTrackingApp()); } class FitnessTrackingApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Fitness Tracker'), ), body: Center( child: Text('Welcome to Fitness Tracker!'), ), ), ); } }
In this code, we define a basic Flutter app with a Material design scaffold containing an app bar and a centered text widget. You can customize this UI to fit your app’s branding and functionality.
3. Accessing Sensors Data
To make your fitness tracking app more accurate and insightful, you can leverage the sensors available on smartphones and wearable devices. Common sensors used in fitness apps include the accelerometer, gyroscope, and GPS. Flutter provides plugins that make it easy to access sensor data.
Let’s take a look at how to access the accelerometer data using the sensors package:
dart import 'package:flutter/material.dart'; import 'package:sensors/sensors.dart'; void main() { runApp(FitnessTrackingApp()); } class FitnessTrackingApp extends StatefulWidget { @override _FitnessTrackingAppState createState() => _FitnessTrackingAppState(); } class _FitnessTrackingAppState extends State<FitnessTrackingApp> { double _accelerometerX = 0.0; double _accelerometerY = 0.0; double _accelerometerZ = 0.0; @override void initState() { super.initState(); accelerometerEvents.listen((AccelerometerEvent event) { setState(() { _accelerometerX = event.x; _accelerometerY = event.y; _accelerometerZ = event.z; }); }); } @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Fitness Tracker'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text('Accelerometer Data'), Text('X: $_accelerometerX'), Text('Y: $_accelerometerY'), Text('Z: $_accelerometerZ'), ], ), ), ), ); } }
In this code, we import the sensors package and create a StatefulWidget to continuously listen to accelerometer data. The accelerometer data is updated in the accelerometerEvents stream, and we display it on the UI.
4. Integrating Health Data
To provide a more comprehensive fitness tracking experience, you can integrate health data from the user’s device. This data can include heart rate, step count, and more. Flutter offers plugins and APIs to access health data on both Android and iOS.
Let’s take a look at how to access step count data using the health package:
dart import 'package:flutter/material.dart'; import 'package:health/health.dart'; void main() { runApp(FitnessTrackingApp()); } class FitnessTrackingApp extends StatefulWidget { @override _FitnessTrackingAppState createState() => _FitnessTrackingAppState(); } class _FitnessTrackingAppState extends State<FitnessTrackingApp> { List<HealthDataPoint> _stepData = []; @override void initState() { super.initState(); _fetchStepData(); } Future<void> _fetchStepData() async { final HealthFactory health = HealthFactory(); try { await health.requestAuthorization(); final List<HealthDataPoint> stepData = await health.getHealthDataFromType(HealthDataType.STEPS); setState(() { _stepData = stepData; }); } catch (e) { print('Error fetching step data: $e'); } } @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Fitness Tracker'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text('Step Count Data'), for (final data in _stepData) Text('Steps: ${data.value} at ${data.date}'), ], ), ), ), ); } }
In this code, we use the health package to request authorization and retrieve step count data. The data is displayed on the UI, allowing users to track their daily steps.
5. Storing and Analyzing Fitness Data
To make your fitness tracking app even more valuable, consider implementing data storage and analysis features. You can use a local database or cloud storage to store user data, and then analyze it to provide insights and trends.
Here’s an example of storing accelerometer data to a local SQLite database using the sqflite package:
dart import 'package:flutter/material.dart'; import 'package:sensors/sensors.dart'; import 'package:sqflite/sqflite.dart'; import 'package:path/path.dart'; void main() { runApp(FitnessTrackingApp()); } class FitnessTrackingApp extends StatefulWidget { @override _FitnessTrackingAppState createState() => _FitnessTrackingAppState(); } class _FitnessTrackingAppState extends State<FitnessTrackingApp> { double _accelerometerX = 0.0; double _accelerometerY = 0.0; double _accelerometerZ = 0.0; late Database _database; @override void initState() { super.initState(); _initDatabase(); accelerometerEvents.listen((AccelerometerEvent event) { setState(() { _accelerometerX = event.x; _accelerometerY = event.y; _accelerometerZ = event.z; }); _saveDataToDatabase(event); }); } Future<void> _initDatabase() async { final databasesPath = await getDatabasesPath(); final path = join(databasesPath, 'fitness_data.db'); _database = await openDatabase( path, version: 1, onCreate: (Database db, int version) async { await db.execute(''' CREATE TABLE accelerometer_data( id INTEGER PRIMARY KEY, x REAL, y REAL, z REAL, timestamp DATETIME DEFAULT CURRENT_TIMESTAMP ) '''); }, ); } Future<void> _saveDataToDatabase(AccelerometerEvent event) async { await _database.insert( 'accelerometer_data', { 'x': event.x, 'y': event.y, 'z': event.z, }, ); } @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Fitness Tracker'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text('Accelerometer Data'), Text('X: $_accelerometerX'), Text('Y: $_accelerometerY'), Text('Z: $_accelerometerZ'), ], ), ), ), ); } }
In this code, we set up a local SQLite database and save accelerometer data to it. This data can later be used for analysis and generating reports for the user.
Conclusion
Building a fitness tracking app with Flutter is an exciting journey that combines mobile development with health and fitness monitoring. In this tutorial, we covered the basics of setting up your Flutter environment, creating a user interface, accessing sensor data, integrating health data, and storing and analyzing fitness data.
With the knowledge and code samples provided here, you have a strong foundation to create your fitness tracking app or expand the capabilities of an existing one. The possibilities are endless, from adding more sensors to implementing machine learning algorithms for advanced data analysis.
Remember to keep user privacy and data security in mind when developing fitness tracking apps, as handling sensitive health data comes with great responsibility. Good luck with your fitness tracking app development journey, and may your app help users lead healthier lives!
Happy coding!
Table of Contents
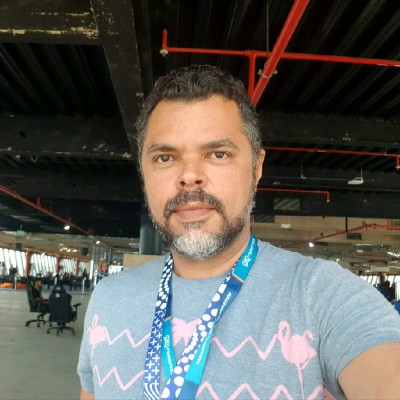
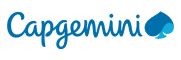