Introduction to Core Text in iOS: Advanced Text Layout and Rendering
When it comes to building rich and visually captivating iOS applications, text is an integral part of the user experience. Whether you’re creating a sleek e-reader, a sophisticated text editor, or a beautifully designed magazine app, having precise control over text layout and rendering is paramount. Apple’s Core Text framework is the key to unlocking advanced text handling in iOS, allowing you to create sophisticated text-driven interfaces and deliver polished, professional content to your users.
Table of Contents
In this comprehensive guide, we will explore the world of Core Text in iOS, covering its essential features, advanced techniques, and real-world examples. By the end of this blog, you’ll have a solid understanding of how to leverage Core Text for text layout and rendering, enabling you to take your iOS app’s user experience to the next level.
1. Understanding Core Text: A Brief Overview
1.1. What is Core Text?
Core Text is a powerful text handling framework introduced by Apple in iOS 3.2. It provides a high-level API for advanced text layout and rendering, making it possible to work with complex text formatting, fonts, and custom text rendering. Core Text is particularly useful when you need precise control over text positioning, custom fonts, and typographic features.
1.2. Why Use Core Text?
While UIKit offers basic text rendering capabilities, Core Text goes beyond the basics, allowing developers to create rich, typographically sophisticated text layouts. Here are some key reasons to consider using Core Text in your iOS applications:
- Advanced Text Formatting: Core Text supports complex text formatting, including multi-column layouts, custom line spacing, and precise control over character and paragraph attributes.
- Custom Fonts: With Core Text, you can easily use custom fonts, making it perfect for applications with unique branding or design requirements.
- High-Performance Rendering: Core Text is designed for high-performance text rendering, making it suitable for applications with large amounts of text content.
- Accessibility: Core Text integrates seamlessly with iOS’s accessibility features, ensuring that your text is accessible to all users.
- Rich Text Editing: Core Text can be used to build powerful text editing interfaces, enabling features like text selection, highlighting, and more.
2. Getting Started with Core Text
Now that we understand the importance of Core Text, let’s dive into the basics and see how to get started with this framework.
2.1. Importing Core Text
To use Core Text in your iOS project, you’ll need to import the Core Text framework. You can do this by adding the following import statement to your Swift or Objective-C source file:
swift import CoreText
2.2. Creating a Core Text View
To display text using Core Text, you’ll typically use a custom view that subclasses UIView or UILabel. This custom view will handle the Core Text rendering for you. Here’s a simple example of how to create a Core Text view in Swift:
swift import UIKit import CoreText class CoreTextView: UIView { override func draw(_ rect: CGRect) { super.draw(rect) // Create a Core Text context guard let context = UIGraphicsGetCurrentContext() else { return } // Configure your text properties (font, color, etc.) let textColor = UIColor.black.cgColor let font = CTFontCreateWithName("Helvetica" as CFString, 24, nil) // Create a Core Text attributed string let text = NSAttributedString(string: "Hello, Core Text!", attributes: [ .font: font, .foregroundColor: textColor ]) // Create a Core Text framesetter let framesetter = CTFramesetterCreateWithAttributedString(text as CFAttributedString) // Create a path for the text to be drawn in let path = CGPath(rect: rect, transform: nil) // Create a frame that fits the entire path let frame = CTFramesetterCreateFrame(framesetter, CFRange(location: 0, length: 0), path, nil) // Draw the frame CTFrameDraw(frame, context) } }
In this example, we create a custom CoreTextView that draws a simple “Hello, Core Text!” message on the screen. We configure the font, color, and other text properties, create an attributed string, and then use Core Text to draw the text in the specified rectangle.
3. Advanced Text Layout with Core Text
Now that we’ve covered the basics, let’s explore some advanced text layout techniques you can achieve with Core Text.
3.1. Custom Text Positioning
Core Text allows you to precisely control the positioning of text within a layout. You can adjust the position of individual characters, lines, or entire paragraphs. This level of control is invaluable when working with complex text layouts.
swift // Custom character positioning let text = NSAttributedString(string: "Hello", attributes: [ .font: font, .foregroundColor: textColor, .kern: 5.0 // Adjust character spacing ]) // Custom line spacing let paragraphStyle = NSMutableParagraphStyle() paragraphStyle.lineSpacing = 10 let text = NSAttributedString(string: "Hello\nWorld", attributes: [ .font: font, .foregroundColor: textColor, .paragraphStyle: paragraphStyle ]) // Custom paragraph spacing let paragraphStyle = NSMutableParagraphStyle() paragraphStyle.paragraphSpacing = 20 let text = NSAttributedString(string: "Hello\n\nWorld", attributes: [ .font: font, .foregroundColor: textColor, .paragraphStyle: paragraphStyle ])
In these examples, we demonstrate how to adjust character spacing, line spacing, and paragraph spacing using Core Text. You can fine-tune these attributes to achieve the desired text layout.
3.2. Multi-Column Layouts
Core Text makes it relatively straightforward to create multi-column text layouts, a common requirement in magazine-style apps or complex documents.
swift func createMultiColumnLayout(context: CGContext, rect: CGRect) { // Create a Core Text attributed string with your content // Create a path for the columns let path = CGPath(rect: rect, transform: nil) // Create a framesetter let framesetter = CTFramesetterCreateWithAttributedString(attributedString) // Create a frame for each column let columnCount = 2 let columnWidth = rect.width / CGFloat(columnCount) for columnIndex in 0..<columnCount { // Adjust the origin of the frame for each column let columnOrigin = CGPoint(x: rect.origin.x + CGFloat(columnIndex) * columnWidth, y: rect.origin.y) let columnRect = CGRect(origin: columnOrigin, size: CGSize(width: columnWidth, height: rect.height)) // Create a frame that fits the column let frame = CTFramesetterCreateFrame(framesetter, CFRange(location: 0, length: 0), path, nil) // Draw the frame CTFrameDraw(frame, context) } }
In this example, we create a multi-column text layout using Core Text. We divide the text into columns, each with its own frame, and then draw each frame in the specified position.
3.3. Text Exclusion Paths
Core Text also supports text exclusion paths, which allow you to wrap text around custom shapes or images. This feature is useful for creating text layouts with irregular shapes.
swift func createTextExclusionPath(context: CGContext, rect: CGRect, exclusionPath: UIBezierPath) { // Create a Core Text attributed string with your content // Create a path for the text let path = CGPath(rect: rect, transform: nil) // Create a framesetter let framesetter = CTFramesetterCreateWithAttributedString(attributedString) // Create a frame that excludes the specified path let frame = CTFramesetterCreateFrame(framesetter, CFRange(location: 0, length: 0), path, nil) // Draw the frame CTFrameDraw(frame, context) // Apply the exclusion path exclusionPath.addClip() }
In this example, we create a text exclusion path using Core Text. The text flows around the specified path, allowing for creative text layouts that wrap around images or other irregular shapes.
4. Custom Fonts and Typography
Core Text provides excellent support for custom fonts and typography, allowing you to create visually stunning text layouts.
4.1. Using Custom Fonts
To use custom fonts with Core Text, you’ll need to load the font and create a CTFont instance. Here’s an example of how to use a custom font in your Core Text layout:
swift // Load a custom font file (OTF or TTF) if let customFontPath = Bundle.main.path(forResource: "CustomFont", ofType: "otf"), let customFontData = NSData(contentsOfFile: customFontPath) { let customFontDataProvider = CGDataProvider(data: customFontData) if let customFont = CGFont(customFontDataProvider) { // Create a CTFont instance let fontSize: CGFloat = 24.0 let ctFont = CTFontCreateWithGraphicsFont(customFont, fontSize, nil, nil) // Use the custom font in your attributed string let text = NSAttributedString(string: "Custom Font Example", attributes: [ .font: ctFont, .foregroundColor: textColor ]) } }
In this example, we load a custom font file and create a CTFont instance from it. You can then use this custom font in your attributed string.
4.2. Typography Features
Core Text also allows you to access advanced typography features, such as ligatures, kerning, and more. Here’s how to enable ligatures and kerning for your text:
swift // Enable ligatures and kerning let text = NSAttributedString(string: "Typography", attributes: [ .font: font, .foregroundColor: textColor, .ligature: 1, // Enable ligatures .kern: 5.0 // Adjust kerning ])
In this example, we enable ligatures and adjust kerning for the text. Core Text provides precise control over these typographic features, allowing you to fine-tune the appearance of your text.
5. Real-World Examples
To illustrate the power of Core Text in real-world scenarios, let’s explore a couple of practical examples.
5.1. Creating a Rich Text Editor
Imagine you’re building a rich text editor for your iOS app, similar to the one you find in word processing software. Core Text can be a game-changer for this task, as it provides the foundation for handling text formatting, font selection, and advanced layout.
Here are some steps you would take:
- Text Input: Implement a text input mechanism to capture user input, such as keyboard interactions and text selections.
- Attributed Text: Convert user input into attributed text, where you specify formatting attributes like font, color, and style.
- Core Text Rendering: Utilize Core Text to render the attributed text on the screen. You can apply the attributes and layout information to ensure the text is displayed correctly.
- Text Editing: Implement features like text selection, copy and paste, undo/redo, and more using Core Text’s capabilities for text manipulation.
- Customization: Allow users to customize the text appearance, such as font size, color, and alignment, and update the Core Text rendering accordingly.
With Core Text, you have the flexibility to create a polished and feature-rich text editor for your iOS app, giving users the ability to create and format text documents effectively.
5.2. Building a Magazine-Style App
Suppose you’re developing a magazine-style app that showcases articles, images, and multimedia content. Core Text can be your go-to solution for crafting visually appealing and interactive layouts.
Here’s how you can utilize Core Text:
- Content Integration: Import articles and content into your app, including text, images, and multimedia elements.
- Text Layout: Use Core Text to lay out the text content, allowing for multi-column layouts, custom fonts, and precise text positioning.
- Text Exclusion Paths: Employ text exclusion paths to wrap text around images, creating visually engaging layouts with seamless integration of images and text.
- Typography: Leverage Core Text’s typography features to ensure that the text is not only well-structured but also visually appealing, with ligatures, kerning, and other typographic enhancements.
- Interactivity: Implement interactive elements within your text, such as hyperlinks, embedded videos, and interactive graphics. Core Text can handle complex text interactions seamlessly.
By combining Core Text’s capabilities with other iOS technologies, you can create a stunning magazine app that delivers an engaging and immersive reading experience to your users.
6. Best Practices and Tips
Before we wrap up our exploration of Core Text, let’s go over some best practices and tips to make the most of this powerful framework:
6.1. Manage Memory Efficiently
Core Text can involve the creation of various objects, such as fonts, framesetters, and frames. Ensure that you release these objects properly to avoid memory leaks. Use Swift’s ARC (Automatic Reference Counting) for memory management whenever possible.
6.2. Handle Text Selection and Interactivity
If your app requires text selection, highlighting, or interaction (e.g., tapping on hyperlinks), you’ll need to implement custom logic for these features. Core Text provides the foundation for handling these interactions, but you’ll need to manage user input and gestures.
6.3. Test on Different Devices and Text Sizes
Ensure that your Core Text-based layouts look good and function correctly on various iOS devices and text size settings. Consider using Dynamic Type and responsive design practices to accommodate different user preferences.
6.4. Combine with Other Technologies
Core Text is just one piece of the puzzle. Depending on your app’s requirements, you may need to combine it with other iOS technologies, such as UIKit, Core Animation, or WebKit, to create a comprehensive user experience.
6.5. Keep Accessibility in Mind
Accessibility is crucial. Use Core Text in a way that ensures your text content is accessible to all users, including those with disabilities. Pay attention to VoiceOver compatibility and provide alternative descriptions for non-text content.
Conclusion
Core Text is a remarkable framework that empowers iOS developers to take full control of text layout and rendering, opening up a world of possibilities for creating visually stunning and feature-rich applications. Whether you’re building a text-intensive editor, a magazine-style app, or any application that demands precise text manipulation, Core Text is a valuable tool in your iOS development arsenal.
As you embark on your Core Text journey, remember to start with the basics, gradually explore advanced features, and apply best practices for optimal performance and user experience. With dedication and creativity, you can harness the power of Core Text to create iOS applications that leave a lasting impression on your users.
Table of Contents
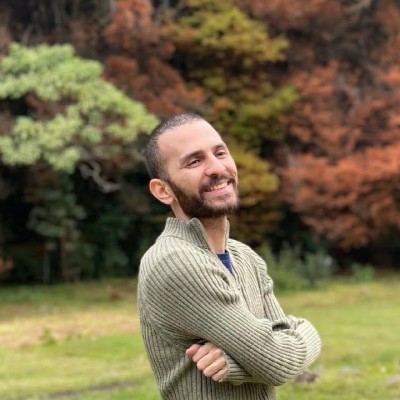
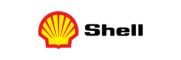