Building Interactive Maps in iOS: Map Annotations and Overlays
In the world of mobile app development, maps play a crucial role in enhancing user experiences. Whether you’re building a location-based service, a navigation app, or simply want to provide users with geographical context, integrating interactive maps into your iOS app is a powerful way to engage your audience.
Table of Contents
Apple’s iOS SDK provides developers with robust tools for working with maps, allowing you to create customized and interactive mapping solutions. In this comprehensive guide, we will dive into the world of iOS map development, focusing specifically on map annotations and overlays. By the end of this tutorial, you’ll have the knowledge and code samples you need to create stunning and interactive maps within your iOS app.
1. Getting Started with MapKit
MapKit is the framework provided by Apple for integrating maps and location services into iOS applications. Before we delve into annotations and overlays, let’s start by setting up a basic map using MapKit.
1.1. Setting Up a MapView
To begin, create a new iOS project or open an existing one. Then, follow these steps:
- Open your storyboard and add a MapView to your view controller’s view.
- Create an outlet for the MapView in your view controller, connecting it to your interface builder.
swift import UIKit import MapKit class MapViewController: UIViewController { @IBOutlet weak var mapView: MKMapView! override func viewDidLoad() { super.viewDidLoad() // Set initial map properties here } }
2. Adding Map Annotations
Map annotations are a great way to mark specific points of interest on your map. These can be custom markers, pins, or even callouts with additional information. Let’s add a simple map annotation to our map view.
2.1. Creating a Custom Annotation Class
First, create a custom class for your annotation. In this example, we’ll create a basic CustomAnnotation class that conforms to the MKAnnotation protocol.
swift import Foundation import MapKit class CustomAnnotation: NSObject, MKAnnotation { var coordinate: CLLocationCoordinate2D var title: String? var subtitle: String? init(coordinate: CLLocationCoordinate2D, title: String?, subtitle: String?) { self.coordinate = coordinate self.title = title self.subtitle = subtitle } }
2.2. Adding Annotations to the Map
Now, let’s add the annotation to our map view in the MapViewController’s viewDidLoad() method:
swift override func viewDidLoad() { super.viewDidLoad() // Set initial map properties let initialLocation = CLLocationCoordinate2D(latitude: 37.7749, longitude: -122.4194) // San Francisco let annotation = CustomAnnotation(coordinate: initialLocation, title: "San Francisco", subtitle: "Golden Gate City") mapView.addAnnotation(annotation) // Set the map's region to focus on the annotation let region = MKCoordinateRegion(center: initialLocation, latitudinalMeters: 10000, longitudinalMeters: 10000) mapView.setRegion(region, animated: true) }
Run your app, and you’ll see a map with a pin at the specified location.
2.3. Customizing Map Annotations
You can customize the appearance of your map annotations by implementing the viewFor annotation method of the MKMapViewDelegate. This allows you to use custom images, colors, and callout views for your annotations.
swift func mapView(_ mapView: MKMapView, viewFor annotation: MKAnnotation) -> MKAnnotationView? { if let customAnnotation = annotation as? CustomAnnotation { let identifier = "CustomPin" var annotationView = mapView.dequeueReusableAnnotationView(withIdentifier: identifier) as? MKPinAnnotationView if annotationView == nil { annotationView = MKPinAnnotationView(annotation: customAnnotation, reuseIdentifier: identifier) annotationView?.canShowCallout = true annotationView?.pinTintColor = .blue // Customize pin color } else { annotationView?.annotation = customAnnotation } return annotationView } return nil }
This code checks if the annotation is of type CustomAnnotation, and if so, it provides a custom appearance for the annotation. You can replace the pin with a custom image, change the pin’s color, or add additional callout views as needed.
3. Working with Map Overlays
Map overlays allow you to display custom shapes, lines, and polygons on your map. This can be useful for highlighting areas, drawing routes, or visualizing data. Let’s explore how to add overlays to your iOS map.
3.1. Creating a Custom Overlay
To create a custom overlay, you’ll need to define a class that conforms to the MKOverlay protocol. Let’s create a simple polygon overlay:
swift import Foundation import MapKit class CustomPolygonOverlay: NSObject, MKOverlay { var coordinate: CLLocationCoordinate2D var boundingMapRect: MKMapRect init(coordinates: [CLLocationCoordinate2D]) { self.coordinate = coordinates[0] // Calculate the bounding map rect for the overlay var topLeft = coordinates[0] var bottomRight = coordinates[0] for coordinate in coordinates { topLeft.latitude = min(topLeft.latitude, coordinate.latitude) topLeft.longitude = min(topLeft.longitude, coordinate.longitude) bottomRight.latitude = max(bottomRight.latitude, coordinate.latitude) bottomRight.longitude = max(bottomRight.longitude, coordinate.longitude) } let topLeftMapPoint = MKMapPoint(topLeft) let bottomRightMapPoint = MKMapPoint(bottomRight) boundingMapRect = MKMapRect(x: topLeftMapPoint.x, y: topLeftMapPoint.y, width: bottomRightMapPoint.x - topLeftMapPoint.x, height: bottomRightMapPoint.y - topLeftMapPoint.y) } }
3.2. Adding Overlays to the Map
Now, let’s add the custom overlay to our map view. In the MapViewController, add the following code to set up the overlay:
swift override func viewDidLoad() { super.viewDidLoad() // Set initial map properties let initialLocation = CLLocationCoordinate2D(latitude: 37.7749, longitude: -122.4194) // San Francisco let annotation = CustomAnnotation(coordinate: initialLocation, title: "San Francisco", subtitle: "Golden Gate City") mapView.addAnnotation(annotation) // Set the map's region to focus on the annotation let region = MKCoordinateRegion(center: initialLocation, latitudinalMeters: 10000, longitudinalMeters: 10000) mapView.setRegion(region, animated: true) // Create coordinates for a polygon overlay let polygonCoordinates = [ CLLocationCoordinate2D(latitude: 37.7749, longitude: -122.4194), CLLocationCoordinate2D(latitude: 37.8049, longitude: -122.4652), CLLocationCoordinate2D(latitude: 37.7249, longitude: -122.5107) ] // Create the custom overlay let polygonOverlay = CustomPolygonOverlay(coordinates: polygonCoordinates) // Add the overlay to the map view mapView.addOverlay(polygonOverlay) }
3.3. Rendering Overlays
To render the overlay, you’ll need to implement the mapView(_ mapView: MKMapView, rendererFor overlay: MKOverlay) -> MKOverlayRenderer method in your MapViewController. This method defines how the overlay should be displayed on the map.
swift func mapView(_ mapView: MKMapView, rendererFor overlay: MKOverlay) -> MKOverlayRenderer { if let polygonOverlay = overlay as? CustomPolygonOverlay { let renderer = MKPolygonRenderer(overlay: polygonOverlay) renderer.fillColor = UIColor.red.withAlphaComponent(0.5) // Set overlay fill color renderer.strokeColor = UIColor.blue // Set overlay border color renderer.lineWidth = 2 // Set overlay border width return renderer } return MKOverlayRenderer(overlay: overlay) }
In this code, we check if the overlay is of type CustomPolygonOverlay and customize its appearance accordingly. You can modify the fillColor, strokeColor, and lineWidth properties to achieve the desired visual effect for your overlay.
Conclusion
In this blog post, we’ve explored the fundamentals of building interactive maps in iOS using MapKit. We’ve covered adding map annotations and overlays, allowing you to create customized and engaging mapping experiences for your app users.
As you continue to develop your iOS app, consider the endless possibilities of map integration. You can expand upon these concepts by adding more advanced features like user location tracking, geofencing, and real-time map updates. The world of iOS map development is both exciting and dynamic, offering numerous opportunities to enhance your app’s functionality and user engagement.
Feel free to experiment with different map styles, custom annotations, and overlays to create a map that aligns with your app’s unique requirements. By combining your creativity with the powerful tools provided by Apple’s MapKit framework, you can deliver a truly immersive and interactive map experience to your iOS app users. Happy mapping!
Table of Contents
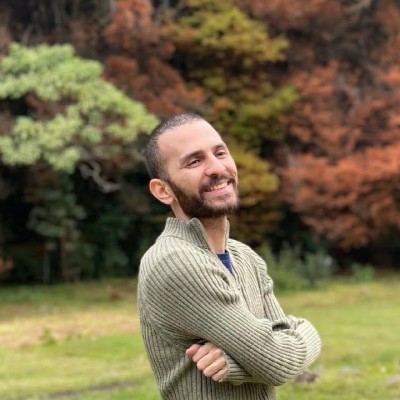
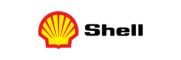