Exploring Core Bluetooth in iOS: Interacting with Bluetooth Devices
Bluetooth technology has become an integral part of our lives. From wireless headphones to smartwatches and even healthcare devices, Bluetooth is everywhere. If you’re an iOS developer, you can harness the power of Bluetooth in your applications using the Core Bluetooth framework. In this comprehensive guide, we’ll dive into the world of Core Bluetooth, exploring how you can interact with Bluetooth devices in your iOS apps.
Table of Contents
1. What is Core Bluetooth?
1.1. Introduction to Core Bluetooth
Core Bluetooth is a framework provided by Apple that allows iOS and macOS devices to communicate with Bluetooth Low Energy (BLE) devices. BLE is a wireless communication technology designed for low-power devices, making it perfect for battery-operated devices like fitness trackers, smart locks, and IoT sensors. With Core Bluetooth, you can create iOS applications that interact with these BLE devices, opening up a world of possibilities.
1.2. Supported iOS Devices
Core Bluetooth is supported on most iOS devices, but the level of support may vary depending on the hardware. Generally, iPhones and iPads with Bluetooth 4.0 and later versions support Core Bluetooth. To ensure compatibility with your target audience, it’s essential to check the minimum iOS version and Bluetooth version requirements for your project.
1.3. Bluetooth Basics
Before diving into Core Bluetooth, let’s briefly review some fundamental Bluetooth concepts:
- Peripheral: A Bluetooth device that provides data or services to other devices, often referred to as a server.
- Central: A Bluetooth device that initiates connections to peripherals and consumes data or services from them, often referred to as a client.
- Service: A collection of related characteristics that define a particular function of a peripheral.
- Characteristic: A single data point within a service that can be read, written, or subscribed to for notifications.
Now that we have a basic understanding of Bluetooth, let’s get started with Core Bluetooth.
2. Getting Started with Core Bluetooth
2.1. Setting up a New Xcode Project
To begin working with Core Bluetooth, create a new Xcode project or open an existing one. If you’re starting from scratch, you can create a Single View App or choose a template that best suits your project’s needs.
2.2. Importing Core Bluetooth Framework
Core Bluetooth is available as a framework, so you’ll need to import it into your Xcode project. To do this:
- Select your project in the Project Navigator.
- Go to the “General” tab for your target.
- Scroll down to the “Frameworks, Libraries, and Embedded Content” section.
- Click the “+” button to add a framework.
- Search for “CoreBluetooth” and add it to your project.
2.3. Permissions and Capabilities
Before your app can use Bluetooth, you need to specify permissions and capabilities in your project settings. Make sure to include the following entries in your project’s Info.plist file:
- Privacy – Bluetooth Peripheral Usage Description: A message explaining why your app needs access to Bluetooth peripheral devices.
- Privacy – Bluetooth Always Usage Description: If your app requires background Bluetooth access, provide a usage description for this as well.
With the initial setup out of the way, it’s time to start coding!
3. Scanning for Nearby Bluetooth Devices
3.1. Initializing the Central Manager
The Central Manager is the central piece of your Core Bluetooth implementation. It’s responsible for managing the discovery and connection to nearby peripherals. To create a Central Manager instance, declare a property in your view controller:
swift import CoreBluetooth class BluetoothManager: NSObject, CBCentralManagerDelegate { var centralManager: CBCentralManager! override init() { super.init() centralManager = CBCentralManager(delegate: self, queue: nil) } // Implement CBCentralManagerDelegate methods here }
The CBCentralManagerDelegate protocol defines methods for managing the central manager’s state and discovering nearby peripherals.
3.2. Scanning for Peripherals
Once your central manager is initialized, you can start scanning for nearby peripherals using the scanForPeripherals(withServices:options:) method. This method allows you to specify the services you’re interested in discovering. If you want to discover all nearby peripherals, pass nil for the services parameter.
swift func startScanning() { centralManager.scanForPeripherals(withServices: nil, options: nil) }
3.3. Handling Peripheral Discovery
As your app scans for peripherals, the central manager will invoke the centralManager(_:didDiscover:advertisementData:rssi:) delegate method for each discovered peripheral. Here’s how you can implement this method to handle peripheral discovery:
swift func centralManager( _ central: CBCentralManager, didDiscover peripheral: CBPeripheral, advertisementData: [String: Any], rssi RSSI: NSNumber ) { // Handle the discovered peripheral here }
In this method, you can access the discovered peripheral, its advertisement data, and its received signal strength indicator (RSSI). You can use this information to identify and connect to the desired peripheral.
With the scanning process in place, your app can now discover nearby Bluetooth devices. In the next section, we’ll explore how to connect to these devices.
4. Connecting to Bluetooth Devices
4.1. Peripheral State Changes
Before establishing a connection to a peripheral, you need to be aware of its state changes. The central manager informs you about these changes through the centralManager(_:didUpdate:advertisementData:rssi:) delegate method.
swift func centralManager( _ central: CBCentralManager, didUpdate state: CBManagerState ) { switch state { case .poweredOn: // Bluetooth is powered on and ready to use case .poweredOff: // Bluetooth is powered off; notify the user to enable it case .resetting: // Bluetooth is resetting; handle reconnection logic case .unauthorized: // The app is not authorized to use Bluetooth case .unknown: // Bluetooth state is unknown case .unsupported: // Bluetooth is unsupported on this device @unknown default: break } }
Handle each state accordingly. If Bluetooth is powered on, your app can proceed with connecting to peripherals.
4.2. Establishing a Connection
To establish a connection to a peripheral, you first need to stop scanning if it’s currently active. Then, use the connect(_:options:) method of the central manager:
swift func connectToPeripheral(_ peripheral: CBPeripheral) { centralManager.stopScan() // Stop scanning to save power centralManager.connect(peripheral, options: nil) }
4.3. Managing Connections
Once a connection is established, you’ll receive a callback in the centralManager(_:didConnect:) delegate method. You should also implement the centralManager(_:didFailToConnect:error:) and centralManager(_:didDisconnectPeripheral:error:) delegate methods to handle connection failures and disconnections gracefully.
swift func centralManager( _ central: CBCentralManager, didConnect peripheral: CBPeripheral ) { // Successfully connected to the peripheral } func centralManager( _ central: CBCentralManager, didFailToConnect peripheral: CBPeripheral, error: Error? ) { // Failed to connect to the peripheral; handle the error } func centralManager( _ central: CBCentralManager, didDisconnectPeripheral peripheral: CBPeripheral, error: Error? ) { // Peripheral disconnected; handle the disconnection }
With these methods in place, your app can establish and manage connections with Bluetooth devices. But to interact with these devices, you need to discover their services and characteristics.
5. Discovering Services and Characteristics
5.1. Understanding Services and Characteristics
In the world of Bluetooth, services and characteristics define the functionality and data provided by a peripheral. Services group related characteristics, and each characteristic represents a specific data point or action that your app can read, write, or subscribe to for notifications.
Before you can interact with a peripheral, you need to discover its services and characteristics. This is typically done after successfully connecting to the peripheral.
5.2. Discovering Services
To discover the services provided by a peripheral, you can use the discoverServices(_:) method of the connected CBPeripheral instance:
swift func discoverServices(for peripheral: CBPeripheral) { peripheral.delegate = self // Set the peripheral's delegate peripheral.discoverServices(nil) // Discover all services }
By calling discoverServices(_:) with nil as the parameter, you request the peripheral to discover all available services. Alternatively, you can specify an array of CBUUID objects to limit the discovery to specific services.
5.3. Exploring Characteristics
Once the peripheral discovers its services, you’ll receive a callback in the peripheral(_:didDiscoverServices:) delegate method:
swift func peripheral( _ peripheral: CBPeripheral, didDiscoverServices error: Error? ) { guard error == nil else { // Handle the error return } for service in peripheral.services ?? [] { // Explore characteristics within each service peripheral.discoverCharacteristics(nil, for: service) } }
In this method, you can loop through the discovered services and use the discoverCharacteristics(_:for:) method to explore the characteristics within each service. Again, passing nil as the parameter will discover all characteristics for the given service.
With the services and characteristics discovered, you’re now ready to interact with Bluetooth devices.
6. Interacting with Bluetooth Devices
6.1. Reading and Writing Characteristics
To interact with a characteristic, you can use the readValue(for:) and writeValue(_:for:type:) methods provided by the CBPeripheral instance:
swift func readCharacteristic(_ characteristic: CBCharacteristic) { peripheral.readValue(for: characteristic) } func writeValue(_ value: Data, to characteristic: CBCharacteristic) { peripheral.writeValue(value, for: characteristic, type: .withResponse) }
Reading a characteristic’s value is straightforward, but writing requires a bit more consideration. When writing to a characteristic, you can choose between two types:
- .withResponse: This option expects the peripheral to confirm that it received the data correctly. Use this when you need to ensure data integrity.
- .withoutResponse: This option sends the data without waiting for confirmation, which can be faster but may not guarantee delivery.
6.2. Receiving Notifications
Some characteristics provide notifications, allowing the peripheral to send data to your app whenever the characteristic’s value changes. To enable notifications for a characteristic, use the setNotifyValue(_:for:) method:
swift func enableNotifications(for characteristic: CBCharacteristic) { peripheral.setNotifyValue(true, for: characteristic) }
Once notifications are enabled, you’ll receive updates in the peripheral(_:didUpdateValueFor:error:) delegate method:
swift func peripheral( _ peripheral: CBPeripheral, didUpdateValueFor characteristic: CBCharacteristic, error: Error? ) { if let error = error { // Handle the error return } // Process the updated characteristic value }
6.3. Handling Errors
When working with Bluetooth devices, it’s crucial to handle errors gracefully. Errors can occur during connection, data transfer, or any other interaction with peripherals. Always check for error conditions and provide appropriate feedback to the user.
7. Advanced Core Bluetooth Concepts
7.1. Background Execution
In some cases, you may need your app to communicate with Bluetooth peripherals in the background. For example, a fitness tracking app might want to collect data from a heart rate monitor even when the app is not actively running. To support background execution, you’ll need to set up background modes and manage background tasks carefully.
7.2. Bluetooth Low Energy (BLE)
Core Bluetooth primarily focuses on Bluetooth Low Energy (BLE) devices. BLE is a power-efficient variant of Bluetooth designed for devices with limited battery capacity. Understanding BLE’s characteristics and limitations is essential for building efficient and reliable Bluetooth applications.
7.3. Peripheral Mode
While we’ve mainly discussed the central role in Bluetooth communication, iOS devices can also act as peripherals. In peripheral mode, your iOS app can advertise itself and provide services to other central devices. This mode is useful for scenarios where your app needs to share data or functionality with nearby devices.
8. Real-World Examples
Now that you’ve learned the basics of Core Bluetooth, let’s explore a couple of real-world examples to demonstrate its practical application.
8.1. Building a Bluetooth Heart Rate Monitor
Imagine you’re building a fitness app, and you want to integrate a Bluetooth heart rate monitor. You can use Core Bluetooth to connect to the heart rate monitor, read its heart rate data, and display it in your app in real-time.
8.2. Creating a Bluetooth-controlled Toy
Let’s say you’re developing a children’s app, and you want to create an interactive Bluetooth-controlled toy. You can use Core Bluetooth to connect to the toy, send commands to control its movement or lights, and provide an engaging experience for young users.
By leveraging Core Bluetooth, you can add exciting and innovative features to your iOS applications.
Conclusion
Core Bluetooth is a powerful framework that empowers iOS developers to interact with Bluetooth devices seamlessly. Whether you’re building a fitness app, a home automation solution, or a fun Bluetooth-controlled toy, Core Bluetooth opens up a world of possibilities. With this comprehensive guide, you now have the knowledge and tools to start exploring and creating amazing Bluetooth-enabled iOS applications. So, go ahead and start building your own Bluetooth-connected experiences!
Table of Contents
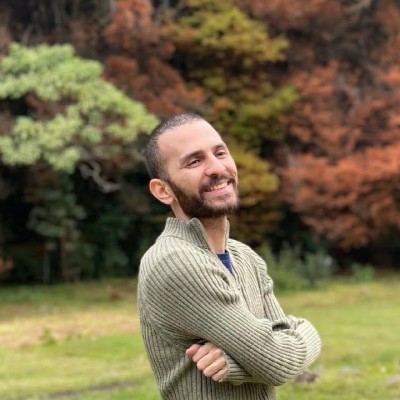
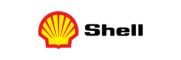