Building Augmented Reality Filters in iOS: ARKit and Vision Framework
Augmented reality (AR) has taken the tech world by storm, and iOS is at the forefront of this exciting technology. Apple’s ARKit and Vision Framework offer powerful tools for creating immersive AR experiences, including AR filters that can transform the way users interact with the world around them. In this comprehensive guide, we’ll explore how to build captivating AR filters for iOS using ARKit and the Vision Framework, complete with code samples and step-by-step instructions.
Table of Contents
1. Introduction to ARKit and Vision Framework
1.1. What are AR Filters?
AR filters, also known as augmented reality filters, are interactive digital overlays that enhance the real-world environment when viewed through a compatible device, such as a smartphone or AR headset. These filters can add effects like virtual objects, animations, and image recognition to create engaging and immersive experiences for users. AR filters have gained immense popularity in social media apps, gaming, and various industries for marketing and entertainment purposes.
1.2. Why Use ARKit and Vision Framework?
Apple’s ARKit and Vision Framework are essential tools for developers looking to create high-quality AR filters on iOS. ARKit provides a foundation for building AR experiences by enabling motion tracking, environment understanding, and rendering 3D objects in real time. The Vision Framework, on the other hand, offers robust image and facial recognition capabilities, making it ideal for implementing interactive and responsive AR filters.
In this blog, we’ll dive deep into these technologies to create impressive AR filters for iOS.
2. Setting Up the Project
2.1. Prerequisites
Before we start building AR filters, ensure you have the following prerequisites:
- Xcode installed on your Mac.
- An iOS device with ARKit support (iPhone 6s and later).
- Basic knowledge of Swift programming.
2.2. Creating a New ARKit Project
Let’s kickstart our AR filter project by creating a new ARKit project in Xcode:
- Open Xcode and select “File” > “New” > “Project.”
- Choose the “Augmented Reality App” template.
- Enter a product name and other project details.
- Make sure to select the “Swift” language.
- Choose “ARKit” under Content Technology.
- Click “Next” and select a location to save your project.
- Click “Create” to generate the project.
Now you have a basic ARKit project ready to be transformed into an AR filter masterpiece.
3. Understanding ARKit
3.1. Basics of ARKit
ARKit is Apple’s framework for augmented reality development. It enables developers to create AR applications by providing essential features such as:
- World Tracking: ARKit uses the device’s camera and sensors to track the real-world environment, allowing virtual objects to interact with it accurately.
- Scene Understanding: ARKit can recognize and understand the geometry and features of the environment, like horizontal planes (tables, floors) and vertical surfaces (walls).
- Rendering: It provides tools to render 3D objects, animations, and visual effects in real time.
3.2. ARKit Features for Filters
For building AR filters, we’ll primarily focus on the following ARKit features:
- Motion Tracking: This allows us to track the device’s movement accurately, ensuring that our virtual objects stay anchored in the real world.
- Image Tracking: ARKit can recognize and track specific images or objects, making it perfect for image-based AR filters.
- Face Tracking: If you plan to create face filters or effects, ARKit’s face tracking capabilities will be crucial. It can detect and track facial features in real time.
Now that we have a basic understanding of ARKit, let’s move on to leveraging the Vision Framework for image processing.
4. Working with the Vision Framework
4.1. Introduction to Vision Framework
The Vision Framework is a powerful tool for processing images and video in real time. It provides a wide range of capabilities, including image recognition, text detection, face detection, and more. For AR filters, we’ll focus on image recognition, which allows us to identify specific images or patterns in the camera feed.
4.2. Leveraging Vision for Image Processing
To use the Vision Framework in your AR filter project, follow these steps:
Import the Vision framework at the top of your Swift file:
swift import Vision
Set up a Vision request for image recognition:
swift let imageRequestHandler = VNImageRequestHandler(ciImage: ciImage, options: [:]) let imageRequest = VNRecognizeTextRequest { request, error in // Handle image recognition results here } do { try imageRequestHandler.perform([imageRequest]) } catch { print("Error performing image recognition: \(error)") }
Implement the image recognition result handler to process the recognized text or objects:
swift func handleImageRecognitionResults(request: VNRequest, error: Error?) { guard let observations = request.results as? [VNRecognizedObjectObservation] else { return } for observation in observations { // Process recognized objects here } }
This is a basic setup for image recognition using the Vision Framework. You can customize it to recognize specific images or objects relevant to your AR filter.
5. Building Your AR Filter
5.1. Designing the Filter
Before diving into the code, it’s essential to design your AR filter concept. Consider what kind of virtual objects or effects you want to overlay on the real-world environment. Whether it’s face filters, 3D models, or interactive animations, having a clear design in mind will streamline the development process.
5.2. Implementing Image Recognition
To integrate image recognition into your AR filter, follow these steps:
Capture the camera feed using ARKit and convert it into a CIImage:
swift guard let currentFrame = sceneView.session.currentFrame, let pixelBuffer = currentFrame.capturedImage else { return } let ciImage = CIImage(cvPixelBuffer: pixelBuffer)
Set up the Vision Framework request as previously explained.
In the image recognition result handler, determine when and how to trigger your AR filter based on the recognized objects. For example, you might want to overlay a virtual object when a specific image is detected:
swift for observation in observations { if let recognizedObject = observation.labels.first { let detectedObjectName = recognizedObject.identifier if detectedObjectName == "yourTargetImage" { // Trigger your AR filter here } } }
5.3. Overlaying Virtual Objects
Now that you have the foundation in place, it’s time to overlay virtual objects onto the real-world environment. ARKit makes this relatively straightforward:
Create a 3D model or object using SceneKit or any other 3D modeling tool.
Add the virtual object to the AR scene at the appropriate position and orientation:
swift let virtualObject = SCNNode() // Set the position, rotation, and scale of the virtual object sceneView.scene.rootNode.addChildNode(virtualObject)
To ensure that the virtual object stays anchored to the real world, update its position and orientation using ARKit’s motion tracking capabilities.
With these steps, you can create AR filters that react to recognized images and overlay virtual objects seamlessly.
6. Testing Your AR Filter
6.1. Running the App on iOS Device
Before you can test your AR filter, you’ll need to deploy your app to an iOS device. Follow these steps:
- Connect your iOS device to your Mac using a USB cable.
- In Xcode, select your connected device as the deployment target.
- Click the “Build and Run” button (??) to install and run your app on the iOS device.
- Once the app is running, point the device’s camera at the target image or objects to trigger your AR filter.
6.2. Debugging and Fine-Tuning
During testing, you may encounter issues such as tracking inaccuracies or unexpected behavior. Use Xcode’s debugging tools to identify and resolve these issues. Common debugging techniques include:
- Adding print statements to your code to log relevant information.
- Using Xcode’s visual debugger to inspect 3D scene contents and view ARKit diagnostics.
- Fine-tune your AR filter’s performance and responsiveness based on user testing and feedback. This may involve adjusting the object’s scale, position, or rotation, or optimizing image recognition accuracy.
7. Deployment and Optimization
7.1. Preparing for App Store
Once you’re satisfied with your AR filter, it’s time to prepare your app for submission to the App Store. Ensure that you have:
- Created compelling app icons and screenshots.
- Written clear and concise app descriptions.
- Tested your app on multiple iOS devices to ensure compatibility.
- Follow Apple’s App Store guidelines and submission process to publish your app and make your AR filter accessible to a broader audience.
7.2. Performance Optimization Tips
Optimizing the performance of your AR filter is crucial for a smooth user experience. Consider the following tips:
- Use lightweight 3D models to reduce rendering overhead.
- Minimize the number of active virtual objects in the scene to conserve resources.
- Implement occlusion handling to make virtual objects appear behind real-world objects when necessary.
- Optimize image recognition models for faster processing.
By following these optimization strategies, you can ensure that your AR filter runs efficiently on a wide range of iOS devices.
Conclusion
In this guide, we’ve explored how to build augmented reality filters for iOS using ARKit and the Vision Framework. We started by setting up a new ARKit project, understanding the basics of ARKit, and leveraging the Vision Framework for image recognition. We then delved into the process of designing and implementing AR filters, testing them on iOS devices, and optimizing their performance for a seamless user experience.
Augmented reality filters have become a powerful tool for engaging users in various applications, from social media to gaming and marketing. By harnessing the capabilities of ARKit and the Vision Framework, you can create captivating and interactive AR filters that enhance user experiences and set your iOS app apart.
Now, armed with the knowledge and tools presented in this guide, you’re ready to embark on your journey of building immersive AR filters for iOS. Experiment, innovate, and create AR experiences that leave users amazed and entertained. The world of augmented reality is at your fingertips, waiting for your creativity to bring it to life. Happy coding!
Table of Contents
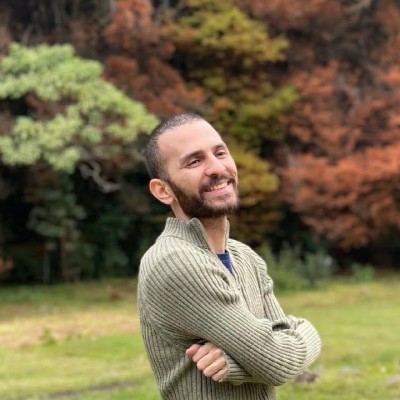
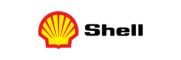