Building Apple Pay Integration in iOS: Simplifying In-App Payments
In the fast-paced world of mobile app development, providing a seamless and secure payment experience is crucial. Apple Pay has emerged as a powerful tool for simplifying in-app payments on iOS devices. Integrating Apple Pay into your iOS app not only enhances user convenience but also helps build trust and credibility.
Table of Contents
In this comprehensive guide, we will walk you through the process of building Apple Pay integration in your iOS app. Whether you’re creating an e-commerce platform, a food delivery service, or a subscription-based app, this step-by-step tutorial will equip you with the knowledge and code samples you need to streamline in-app payments and deliver an exceptional user experience.
1. Why Integrate Apple Pay?
Before we dive into the technical details, let’s briefly discuss why integrating Apple Pay into your iOS app is a smart choice.
1.1. Enhanced User Experience
Apple Pay offers a frictionless payment process, reducing the need for users to manually enter their payment information. This convenience can significantly improve the overall user experience and increase conversion rates.
1.2. Security and Trust
Apple Pay transactions are highly secure, utilizing biometric authentication (Touch ID or Face ID) and tokenization to protect sensitive data. By integrating Apple Pay, you reassure your users that their financial information is safe.
1.3. Global Reach
Apple Pay is available in numerous countries, making it a valuable addition for apps with an international user base. This broad reach can expand your app’s market potential.
2. Prerequisites
Before we begin the integration process, ensure that you meet the following prerequisites:
- An iOS app project in Xcode.
- A merchant account with Apple for processing payments.
- A valid Apple Developer Program membership.
An understanding of Swift and UIKit.
Step 1: Configure Your Xcode Project
Let’s start by configuring your Xcode project to support Apple Pay.
1. Enable Apple Pay Capabilities
- Open your Xcode project, go to the “Signing & Capabilities” tab, and add the “Apple Pay” capability. This step will enable Apple Pay for your app.
- Enable Apple Pay Capabilities
2. Merchant ID Setup
- In the Apple Developer portal, navigate to “Certificates, Identifiers & Profiles.” Under “Identifiers,” create a new Merchant ID, and associate it with your app.
- Merchant ID Setup
3. Configure Payment Processing
Next, you need to set up your payment processing backend to work with Apple Pay. This involves integrating with a payment gateway like Stripe or Braintree. Refer to the documentation of your chosen payment gateway for detailed instructions on how to configure it.
Step 2: Add Apple Pay Buttons to Your App
Now that your Xcode project is configured, it’s time to add Apple Pay buttons to your app’s user interface. Apple provides a convenient way to do this using PKPaymentButton.
1. Import PassKit
In your view controller where you want to display the Apple Pay button, import the PassKit framework:
swift import PassKit
2. Create the Apple Pay Button
Add the following code to create an Apple Pay button:
swift let paymentButton = PKPaymentButton(paymentButtonType: .plain, paymentButtonStyle: .black) paymentButton.addTarget(self, action: #selector(handleApplePayButtonTap), for: .touchUpInside) view.addSubview(paymentButton)
3. Handle Button Tap
Implement the handleApplePayButtonTap method to handle button taps. This is where you’ll initiate the Apple Pay payment process:
swift @objc func handleApplePayButtonTap() { // Implement the payment process here }
Your app’s view should now display an Apple Pay button that users can tap to make payments.
Step 3: Initialize an Apple Pay Payment Request
Before you can process an Apple Pay payment, you need to create a payment request that specifies the items the user is purchasing and the payment information.
1. Define Payment Items
Create an array of PKPaymentSummaryItem objects to represent the items the user is purchasing. These items will be displayed in the Apple Pay payment sheet:
swift let item1 = PKPaymentSummaryItem(label: "Product 1", amount: NSDecimalNumber(string: "10.00")) let item2 = PKPaymentSummaryItem(label: "Product 2", amount: NSDecimalNumber(string: "5.00")) let total = PKPaymentSummaryItem(label: "Total", amount: NSDecimalNumber(string: "15.00")) let paymentItems = [item1, item2, total]
2. Create a Payment Request
Initialize a PKPaymentRequest object and configure it with your payment items, merchant identifier, and other required information:
swift let paymentRequest = PKPaymentRequest() paymentRequest.merchantIdentifier = "your_merchant_identifier" paymentRequest.supportedNetworks = [.visa, .masterCard, .amex] // Add supported card networks paymentRequest.merchantCapabilities = .capability3DS paymentRequest.countryCode = "US" paymentRequest.currencyCode = "USD" paymentRequest.paymentSummaryItems = paymentItems
Replace “your_merchant_identifier” with your actual merchant identifier.
Step 4: Present the Apple Pay Payment Sheet
With the payment request prepared, you can now present the Apple Pay payment sheet when the user taps the Apple Pay button.
1. Check Apple Pay Availability
Before presenting the payment sheet, it’s a good practice to check if Apple Pay is available on the user’s device and if they have set up payment cards. Add the following code to do this check:
swift if PKPaymentAuthorizationViewController.canMakePayments() { let paymentAuthorizationViewController = PKPaymentAuthorizationViewController(paymentRequest: paymentRequest) if let viewController = paymentAuthorizationViewController { viewController.delegate = self present(viewController, animated: true, completion: nil) } } else { // Apple Pay is not available on this device or no payment cards are set up. // Provide an alternative payment method or inform the user accordingly. }
2. Implement the Delegate Methods
To handle the payment process and receive payment authorization callbacks, conform to the PKPaymentAuthorizationViewControllerDelegate protocol and implement its methods. Here’s an example implementation:
swift extension YourViewController: PKPaymentAuthorizationViewControllerDelegate { func paymentAuthorizationViewController(_ controller: PKPaymentAuthorizationViewController, didAuthorizePayment payment: PKPayment, handler completion: @escaping (PKPaymentAuthorizationStatus) -> Void) { // Handle the payment authorization here. // Send payment information to your backend for processing and validation. // Call the completion handler with the appropriate status. } func paymentAuthorizationViewControllerDidFinish(_ controller: PKPaymentAuthorizationViewController) { // Dismiss the Apple Pay payment sheet. controller.dismiss(animated: true, completion: nil) } }
Step 5: Process the Payment on Your Server
In the didAuthorizePayment delegate method, you should send the payment information to your server for processing and validation. This step is critical for security and ensuring that the payment is legitimate.
1. Send Payment Data to Your Server
Serialize the payment data and send it to your server using a secure HTTPS connection. Your server should then communicate with your payment gateway to process the payment.
swift func paymentAuthorizationViewController(_ controller: PKPaymentAuthorizationViewController, didAuthorizePayment payment: PKPayment, handler completion: @escaping (PKPaymentAuthorizationStatus) -> Void) { // Serialize payment data to send to your server let paymentData = String(data: payment.token.paymentData, encoding: .utf8) // Send payment data to your server over a secure HTTPS connection // After processing on your server and getting a response, handle it accordingly if paymentProcessedSuccessfully { completion(.success) } else { completion(.failure) } }
2. Server-Side Payment Processing
On your server, implement the necessary logic to process the payment using the payment gateway of your choice (e.g., Stripe or Braintree). Your server should handle payment validation, charge creation, and any other required steps.
Once your server confirms the payment’s success or failure, it should send a response back to the app, which you can use to update the UI and inform the user of the transaction outcome.
Step 6: Handle Payment Authorization Status
In the paymentAuthorizationViewController(_:didAuthorizePayment:handler:) method, you return a payment authorization status based on the outcome of your server-side payment processing. Here are the possible status values you can return:
- .success: The payment was successfully authorized and processed.
- .failure: The payment authorization failed.
- .invalidBillingPostalAddress or .invalidShippingContact: The billing or shipping address provided by the user is invalid.
- .PINRequired: The user’s device requires a PIN to authorize the payment.
You should carefully handle these status values to provide appropriate feedback to the user and ensure a smooth payment experience.
Step 7: Handle Payment Completion
After the payment authorization is completed, you should dismiss the Apple Pay payment sheet using the paymentAuthorizationViewControllerDidFinish delegate method. This method gets called regardless of whether the payment was successful or not.
swift func paymentAuthorizationViewControllerDidFinish(_ controller: PKPaymentAuthorizationViewController) { // Dismiss the Apple Pay payment sheet. controller.dismiss(animated: true, completion: nil) }
Conclusion
Congratulations! You’ve successfully integrated Apple Pay into your iOS app, simplifying in-app payments and enhancing the user experience. By following these steps and best practices, you’ve learned how to:
- Configure your Xcode project for Apple Pay.
- Add Apple Pay buttons to your app.
- Initialize an Apple Pay payment request.
- Present the Apple Pay payment sheet.
- Process the payment on your server.
- Handle payment authorization status.
- Provide a smooth payment experience for your users.
Apple Pay integration is a valuable addition to any iOS app, offering a secure and convenient way for users to make payments. Remember to test your integration thoroughly and stay updated with Apple’s guidelines and best practices to ensure a seamless payment experience for your users.
Now that you have the knowledge and code samples, it’s time to take your iOS app to the next level by simplifying in-app payments with Apple Pay. Happy coding!
Table of Contents
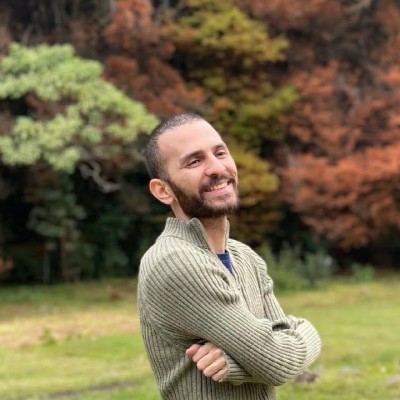
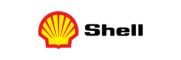