Building Audio and Video Playback in iOS: AVKit Framework
In the world of iOS app development, providing a smooth and immersive audio and video playback experience is paramount. Whether you’re building a music streaming app, a video player, or just integrating multimedia content into your application, the AVKit framework is your go-to tool. In this comprehensive guide, we will explore the capabilities of AVKit and demonstrate how to implement audio and video playback in your iOS app, all while maintaining a great user experience.
Table of Contents
1. What is AVKit?
Apple’s AVKit framework is a powerful and flexible library for handling audio and video playback on iOS devices. It abstracts many of the complexities involved in multimedia playback and provides a high-level API for developers to integrate media into their applications seamlessly. AVKit is built on top of AVFoundation, a lower-level framework, making it more accessible for developers.
2. Key Features of AVKit
Before we dive into the implementation details, let’s explore some of the key features of AVKit:
- User-friendly controls: AVKit provides a user interface (UI) with standard playback controls like play, pause, forward, and rewind. This UI is customizable to match the look and feel of your app.
- AirPlay support: Easily enable AirPlay for your media content, allowing users to stream audio and video to Apple TV and other compatible devices.
- Fullscreen and Picture-in-Picture modes: AVKit allows users to go fullscreen or use Picture-in-Picture mode, enhancing the media consumption experience.
- Subtitle and closed caption support: You can add subtitles and closed captions to your videos, making your content more accessible to a wider audience.
- Background audio playback: Your app can continue to play audio in the background, even when the app is not in the foreground, ensuring a seamless listening experience.
- Adaptive streaming: AVKit supports HTTP Live Streaming (HLS) and can adapt to changing network conditions, providing uninterrupted playback.
Now that we understand what AVKit offers, let’s dive into the implementation.
3. Setting Up Your Xcode Project
To get started with AVKit, create a new Xcode project or open an existing one. Ensure that you have the necessary assets (audio and video files) that you want to include in your app.
3.1. Import AVKit Framework
The first step is to import the AVKit framework into your project. Here’s how you can do it:
- Open your Xcode project.
- Select your project in the Project Navigator.
- Under the “General” tab, find the “Frameworks, Libraries, and Embedded Content” section.
- Click the “+” button and search for “AVKit.”
- Add the AVKit framework to your project.
Now that AVKit is integrated into your project, you’re ready to start using it for audio and video playback.
4. Playing Audio with AVKit
Let’s start by implementing audio playback using AVKit. In this example, we’ll create a simple audio player.
4.1. Import AVKit and AVFoundation
In your view controller where you want to implement audio playback, import both AVKit and AVFoundation:
swift import AVKit import AVFoundation
4.2. Initialize AVPlayerViewController
Next, initialize an AVPlayerViewController in your view controller’s viewDidLoad method:
swift class AudioPlayerViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() // Create an AVPlayerViewController let playerViewController = AVPlayerViewController() // Add it as a child view controller addChild(playerViewController) view.addSubview(playerViewController.view) playerViewController.view.frame = view.bounds playerViewController.view.autoresizingMask = [.flexibleWidth, .flexibleHeight] // Create an AVPlayer with your audio file if let audioURL = Bundle.main.url(forResource: "your_audio_file", withExtension: "mp3") { let player = AVPlayer(url: audioURL) // Set the player for the AVPlayerViewController playerViewController.player = player // Play the audio player.play() } } }
In this code, we first create an instance of AVPlayerViewController and add it as a child view controller. Then, we create an AVPlayer with the URL of your audio file and set it as the player for the AVPlayerViewController. Finally, we start playing the audio using player.play().
4.3. Customize Audio Playback
You can customize the audio playback experience by modifying the AVPlayerViewController instance. For example, you can add playback controls and set the volume:
swift // Show standard playback controls playerViewController.showsPlaybackControls = true // Set the initial volume (0.0 - 1.0) playerViewController.player?.volume = 0.7
These customization options allow you to tailor the audio playback experience to your app’s design and requirements.
5. Playing Video with AVKit
Now, let’s move on to video playback using AVKit. Similar to audio playback, we’ll create a simple video player in this example.
5.1. Initialize AVPlayerViewController for Video
In your view controller where you want to implement video playback, initialize an AVPlayerViewController:
swift class VideoPlayerViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() // Create an AVPlayerViewController let playerViewController = AVPlayerViewController() // Add it as a child view controller addChild(playerViewController) view.addSubview(playerViewController.view) playerViewController.view.frame = view.bounds playerViewController.view.autoresizingMask = [.flexibleWidth, .flexibleHeight] // Create an AVPlayer with your video file if let videoURL = Bundle.main.url(forResource: "your_video_file", withExtension: "mp4") { let player = AVPlayer(url: videoURL) // Set the player for the AVPlayerViewController playerViewController.player = player // Play the video player.play() } } }
This code is quite similar to the audio playback example. We create an AVPlayerViewController, add it as a child view controller, create an AVPlayer with the URL of your video file, set it as the player for the AVPlayerViewController, and start playing the video.
5.2. Video Playback Customization
Just like with audio playback, you can customize the video playback experience. Here are some common customizations:
5.2.1. Customizing Video Controls
You can show or hide the standard playback controls based on your app’s design:
swift // Show standard playback controls playerViewController.showsPlaybackControls = true // Hide playback controls playerViewController.showsPlaybackControls = false
5.2.2. Fullscreen and Picture-in-Picture
AVKit makes it easy to enable fullscreen and Picture-in-Picture modes for your video player:
swift // Allow fullscreen mode playerViewController.enterFullScreen(animated: true) // Enable Picture-in-Picture mode playerViewController.allowsPictureInPicturePlayback = true
5.2.3. Adding Subtitles
If your video has subtitles, you can add them to the player:
swift if let subtitleURL = Bundle.main.url(forResource: "your_subtitle_file", withExtension: "srt") { let subtitle = AVPlayerItem(url: subtitleURL) player.currentItem?.externalSubtitles = [subtitle] }
This allows you to enhance the accessibility of your video content.
6. Advanced Features and Best Practices
As you get more comfortable with AVKit, you may want to explore advanced features and best practices to provide an even better media playback experience in your iOS app.
6.1. Adaptive Streaming
Adaptive streaming, often used with HTTP Live Streaming (HLS), is a technique that adjusts the quality of audio and video streams based on the viewer’s network conditions. AVKit makes it easy to implement adaptive streaming for your media content.
swift if let streamURL = URL(string: "https://example.com/adaptive_stream.m3u8") { let player = AVPlayer(url: streamURL) playerViewController.player = player player.play() }
By using adaptive streaming, you can ensure that your content plays smoothly even on fluctuating network connections.
6.2. Background Audio Playback
To enable background audio playback, you need to make some configurations in your app’s settings:
- In your Xcode project, go to the “Signing & Capabilities” tab.
- Under “Background Modes,” enable “Audio, AirPlay, and Picture in Picture.”
- This setting allows your app to continue playing audio even when it’s in the background, providing a seamless listening experience for users.
6.3. AirPlay Support
AirPlay allows users to stream audio and video from your app to Apple TV and other compatible devices. To support AirPlay, you don’t need to do much. AVKit handles it automatically if your app has the necessary configurations.
Ensure that your app’s audio and video routes are set correctly to enable AirPlay:
swift do { try AVAudioSession.sharedInstance().setCategory(.playback, mode: .default, options: .allowAirPlay) } catch { print("Failed to set audio session category.") }
By setting the audio session category to allow AirPlay, your app will work seamlessly with AirPlay-enabled devices.
Conclusion
The AVKit framework is a powerful tool for building audio and video playback features in your iOS apps. With its user-friendly controls, support for adaptive streaming, background audio playback, and other advanced features, you can create a seamless media experience for your users.
In this guide, we’ve covered the basics of using AVKit to implement audio and video playback. As you explore more complex scenarios and tailor the playback experience to your app’s needs, you’ll discover the true potential of AVKit in enhancing your iOS applications.
Whether you’re building a music streaming app, a video player, or simply integrating multimedia content into your app, AVKit is your reliable companion for delivering high-quality audio and video experiences to iOS users. So go ahead, dive into AVKit, and start creating amazing media playback experiences in your iOS apps today!
Table of Contents
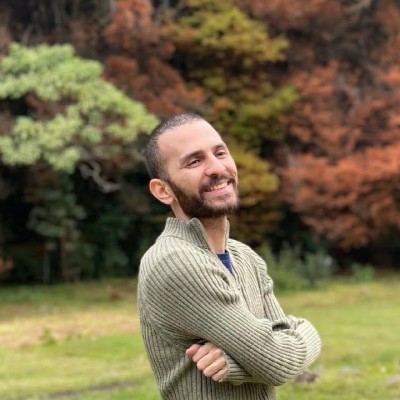
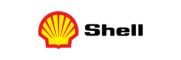