Building Social Sharing Features in iOS Apps: Integrating Social Media
In today’s digitally interconnected world, social media has become an integral part of our lives. Whether it’s sharing a photo, a funny meme, or a noteworthy article, social media platforms offer a convenient way to spread the word. For iOS app developers, integrating social sharing features can significantly enhance user engagement and extend the reach of their applications. In this comprehensive guide, we’ll explore how to seamlessly integrate social media sharing features into your iOS apps, complete with practical code samples and expert insights.
Table of Contents
1. Why Integrate Social Sharing Features?
Before we dive into the technical aspects, let’s understand why integrating social sharing features is a must for iOS apps.
1.1. Expand Your App’s Reach
By allowing users to share content from your app on their social media profiles, you tap into their existing networks. This can lead to increased visibility and user acquisition, as friends and followers of your users discover your app through shared content.
1.2. Boost User Engagement
Sharing content on social media fosters user engagement. It gives users a reason to interact with your app more frequently and encourages them to create and share their own content, further promoting your app.
1.3. Enhance User Experience
Social sharing features can improve the overall user experience by providing a convenient way for users to share their achievements, favorite products, or memorable moments without leaving your app.
Now that we’ve established the importance of integrating social sharing features, let’s dive into the technical implementation.
2. Choosing Social Media Platforms
The first step in integrating social sharing features into your iOS app is selecting the social media platforms you want to support. The most common choices include Facebook, Twitter, Instagram, and LinkedIn, but you can expand or limit this list based on your app’s target audience and content.
2.1. Facebook Sharing
Facebook is one of the largest social media platforms, making it a popular choice for integration. Users can share text, links, images, and videos to their Facebook profiles, groups, or pages. To integrate Facebook sharing, follow these steps:
Step 1: Create a Facebook App
- Go to the Facebook Developer Portal and create a new app.
- Configure the app with your iOS bundle identifier and other necessary settings.
- Obtain your Facebook App ID.
Step 2: Add the Facebook SDK to Your Project
You can integrate the Facebook SDK using CocoaPods or manually adding the SDK to your Xcode project. Here’s an example using CocoaPods:
ruby pod 'FacebookCore' pod 'FacebookShare'
Step 3: Implement Facebook Sharing
Use the Facebook SDK to share content from your app. Here’s a simple code snippet to share a URL:
swift import FacebookShare let content = ShareLinkContent() content.contentURL = URL(string: "https://example.com") let shareDialog = ShareDialog(fromViewController: self, content: content, delegate: nil) shareDialog.show()
2.2. Twitter Sharing
Twitter is known for its concise and real-time sharing. Users can tweet text, images, and links to their Twitter profiles. To integrate Twitter sharing, follow these steps:
Step 1: Create a Twitter App
- Go to the Twitter Developer Portal and create a new app.
- Configure the app with your iOS bundle identifier and other necessary settings.
- Obtain your Twitter API Key and API Secret Key.
Step 2: Add the TwitterKit SDK to Your Project
You can integrate TwitterKit using CocoaPods or manually adding the SDK to your Xcode project. Here’s an example using CocoaPods:
ruby pod 'TwitterKit'
Step 3: Implement Twitter Sharing
Use TwitterKit to compose and post tweets. Here’s a code snippet to share a tweet with an image:
swift import TwitterKit if TWTRTwitter.sharedInstance().sessionStore.hasLoggedInUsers() { if let composer = TWTRComposerViewController.emptyComposer() { composer.setText("Check out this awesome app!") composer.setImage(UIImage(named: "your_image.png")) composer.delegate = self present(composer, animated: true, completion: nil) } }
2.3. Instagram Sharing
Instagram is a popular platform for sharing photos and videos. Although Instagram’s API has limitations compared to other platforms, you can still allow users to open Instagram with pre-filled captions and images. Here’s a basic implementation:
swift let instagramURL = URL(string: "instagram://app")! if UIApplication.shared.canOpenURL(instagramURL) { let imageData = UIImage(named: "your_image.png")!.jpegData(compressionQuality: 1.0) let caption = "Check out this amazing image!" let writePath = try! FileManager.default.url(for: .documentDirectory, in: .userDomainMask, appropriateFor: nil, create: true).appendingPathComponent("instagram.igo") try? imageData?.write(to: writePath, options: .atomic) let fileURL = URL(string: "file:///path_to_your_image.png")! let docController = UIDocumentInteractionController(url: fileURL) docController.delegate = self docController.annotation = ["InstagramCaption": caption] docController.uti = "com.instagram.exclusivegram" docController.presentOpenInMenu(from: self.view.frame, in: self.view, animated: true) } else { // Instagram is not installed }
2.4. LinkedIn Sharing
LinkedIn is a professional networking platform that allows users to share articles, images, and text updates. To integrate LinkedIn sharing, follow these steps:
Step 1: Create a LinkedIn App
- Go to the LinkedIn Developer Portal and create a new app.
- Configure the app with your iOS bundle identifier and other necessary settings.
- Obtain your LinkedIn Client ID and Client Secret.
Step 2: Add the LinkedIn SDK to Your Project
LinkedIn provides an official SDK for iOS app integration. You can add it to your project using CocoaPods or manually.
Step 3: Implement LinkedIn Sharing
Use the LinkedIn SDK to share content from your app. Here’s an example code snippet to share an article:
swift import LinkedInShare let shareText = "Check out this interesting article!" let shareURL = URL(string: "https://example.com/article")! let shareContent = ShareContent(url: shareURL, title: "Article Title", description: "A great article on iOS app development", thumbnailURL: URL(string: "https://example.com/thumbnail.png")) let linkedInViewController = ShareDialog(content: shareContent) present(linkedInViewController, animated: true, completion: nil)
3. Creating a Unified Sharing Experience
Now that you’ve integrated social sharing for various platforms, it’s important to provide a unified and user-friendly sharing experience within your app. Consider the following tips:
3.1. Use Share Sheets
Share sheets are a standard iOS UI component that allows users to choose where and how they want to share content. By leveraging share sheets, you provide a consistent and intuitive sharing experience.
Here’s how you can create a simple share sheet:
swift let shareText = "Check out this amazing content!" let shareURL = URL(string: "https://example.com")! let activityViewController = UIActivityViewController(activityItems: [shareText, shareURL], applicationActivities: nil) activityViewController.popoverPresentationController?.sourceView = self.view self.present(activityViewController, animated: true, completion: nil)
This code presents a share sheet with options to share text and a URL.
3.2. Customize Share Content
Tailor the shared content to match the platform’s requirements. For example, when sharing on Instagram, you may want to focus on images, while on LinkedIn, you might prioritize article titles and descriptions. Customizing the content ensures a more appealing and relevant share.
3.3. Implement Error Handling
Handle errors gracefully. If a user cancels sharing or there’s a network issue, provide clear feedback and options for the user to try again. Good error handling enhances the overall user experience.
4. Tracking Shares and Analytics
To measure the impact of social sharing in your iOS app, it’s essential to track shares and gather analytics. This data can help you understand user behavior and refine your sharing strategy. Here are some key metrics to track:
4.1. Share Count
Keep track of how many times users share content from your app on each social media platform. This metric provides insight into the popularity of your app’s content.
4.2. Referral Traffic
Analyze the traffic generated from shared links. Identify which social media platforms are driving the most traffic to your app and optimize your content for those platforms.
4.3. Conversion Rate
Measure how many users who clicked on shared content actually installed your app or performed desired actions within the app. This helps you assess the effectiveness of your social sharing strategy.
To track these metrics, you can use analytics tools like Google Analytics, Firebase Analytics, or custom event tracking within your app.
5. Security and Privacy Considerations
When integrating social sharing features, it’s crucial to prioritize user privacy and security. Here are some best practices to follow:
5.1. Request Appropriate Permissions
Ensure that your app requests the necessary permissions from users before posting on their behalf. Always follow platform-specific guidelines for user consent.
5.2. Secure API Keys and Tokens
Protect your API keys, tokens, and secrets. Store them securely in your app’s code or use services like Keychain to safeguard sensitive information.
5.3. Respect User Data
Respect user data and privacy. Clearly communicate how and why you’re using their data and allow them to opt out of sharing if they wish.
Conclusion
Integrating social sharing features into your iOS app can be a game-changer for user engagement and app growth. By following platform-specific guidelines and providing a seamless sharing experience, you can harness the power of social media to expand your app’s reach and create a more interactive user community.
Remember to track and analyze your sharing metrics to continually refine your strategy. Prioritize user privacy and security throughout the process to build trust with your audience.
Now that you have the knowledge and code samples to get started, take the leap and enhance your iOS app with social sharing capabilities. Your users will thank you for making it easier to share their favorite moments and discoveries with the world. Happy coding!
Table of Contents
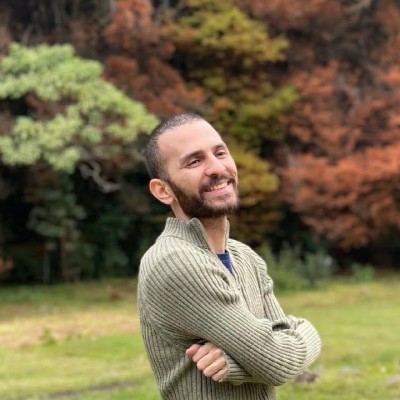
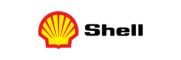