Exploring Core Haptics in iOS: Creating Immersive Tactile Feedback
In the ever-evolving world of mobile app development, providing a seamless and immersive user experience is key to success. One often overlooked aspect of this experience is haptic feedback. Haptics, or tactile feedback, can greatly enhance the user interface by making it more engaging and intuitive. With iOS, Apple introduced Core Haptics, a framework that allows developers to create custom haptic feedback experiences. In this blog, we’ll explore Core Haptics and learn how to leverage it to create immersive tactile feedback in your iOS apps.
Table of Contents
1. Understanding Core Haptics
1.1. What Is Core Haptics?
Core Haptics is a framework introduced by Apple in iOS 13. It enables developers to create and manage custom haptic feedback patterns. Unlike the basic vibration patterns, Core Haptics allows for more nuanced and personalized tactile experiences. With Core Haptics, you can control the intensity, duration, and frequency of haptic feedback, making it a powerful tool for enhancing your app’s user interface.
1.2. Why Use Core Haptics?
Before we dive into the technical details, let’s explore why Core Haptics is a valuable addition to your iOS app development toolkit.
- Immersive User Experience: Haptic feedback can create a more immersive and enjoyable user experience by providing physical feedback for on-screen actions.
- Accessibility: Haptics can improve accessibility by providing tactile cues that assist users with disabilities in navigating your app.
- Brand Differentiation: Custom haptic feedback can set your app apart from competitors and reinforce your brand identity.
- Enhanced Interactivity: Haptics can make your app feel more interactive and responsive, increasing user engagement.
Now that we understand the importance of Core Haptics, let’s dive into how to implement it in your iOS app.
2. Getting Started with Core Haptics
2.1. Prerequisites
To work with Core Haptics, you’ll need the following:
- A Mac running macOS Catalina (10.15) or later.
- Xcode 11 or later.
- An iOS device with iOS 13 or later for testing, as Core Haptics doesn’t work in simulators.
2.2. Adding Core Haptics to Your Project
- Open your Xcode project or create a new one.
- Import the Core Haptics framework by adding the following import statement to your Swift file:
swift import CoreHaptics
3. To use Core Haptics, you need to request permission from the user. Add the UIFeedbackGenerator capability to your app by enabling the “Haptic Feedback” capability in your Xcode project settings.
4. Import Core Haptics and create an instance of CHHapticEngine:
swift var engine: CHHapticEngine! do { engine = try CHHapticEngine() try engine.start() } catch { print("Error starting haptic engine: \(error.localizedDescription)") }
5. With your engine set up, you can now create custom haptic feedback patterns and play them at specific times in your app.
3. Creating Custom Haptic Feedback Patterns
One of the exciting features of Core Haptics is the ability to create your own custom haptic feedback patterns. This level of customization allows you to tailor the tactile experience to your app’s specific needs.
3.1. Creating a Haptic Event
To create a custom haptic event, you’ll need to define a CHHapticEvent object. This object encapsulates the haptic feedback’s properties, such as intensity and duration.
swift // Define a haptic event let intensity = CHHapticEventParameter(parameterID: .hapticIntensity, value: 1.0) let sharpness = CHHapticEventParameter(parameterID: .hapticSharpness, value: 1.0) let event = CHHapticEvent(eventType: .hapticTransient, parameters: [intensity, sharpness], relativeTime: 0)
In the code above, we’ve created a haptic event with maximum intensity and sharpness, resulting in a strong and sharp tactile feedback.
3.2. Creating a Haptic Pattern
Once you have defined your haptic event(s), you can group them into a CHHapticPattern. This pattern can represent a single haptic feedback or a sequence of events.
swift // Define a haptic pattern let pattern = try? CHHapticPattern(events: [event], parameters: [])
3.3. Playing the Haptic Feedback
Now that you have your haptic pattern, you can play it using your haptic engine:
swift // Play the haptic pattern do { let player = try engine.makePlayer(with: pattern!) try player.start(atTime: CHHapticTimeImmediate) } catch { print("Error playing haptic pattern: \(error.localizedDescription)") }
Congratulations! You’ve just created and played a custom haptic feedback pattern in your iOS app.
4. Exploring Haptic Feedback Styles
Core Haptics provides various feedback styles that you can use to enhance different parts of your app. Let’s explore some of these styles and their use cases.
4.1. Transient Haptics
Transient haptics are short bursts of tactile feedback that are suitable for notifying users about quick actions or events. For example, you can use transient haptics to confirm a button press.
swift // Create a transient haptic pattern let transientPattern = try? CHHapticPattern(events: [event], parameters: [])
4.2. Continuous Haptics
Continuous haptics, as the name suggests, provide ongoing tactile feedback. These are ideal for simulating continuous actions or effects, such as scrolling or ongoing background tasks.
swift // Create a continuous haptic pattern let continuousPattern = try? CHHapticPattern(events: [event, event, event], parameters: [])
4.3. Pattern Loops
You can also create haptic patterns that loop, allowing you to provide extended or repeating feedback. This is useful for scenarios where you want to maintain a continuous tactile experience, like during a game or while recording audio.
swift // Create a looping haptic pattern let loopedPattern = try? CHHapticPattern(events: [event], parameters: [], duration: 5.0)
4.4. Custom Feedback
While the predefined styles are useful, you can combine and customize haptic events to create entirely unique feedback experiences tailored to your app’s requirements.
swift // Create a custom haptic pattern with multiple events let customPattern = try? CHHapticPattern(events: [event1, event2, event3], parameters: [])
5. Fine-Tuning Haptic Feedback
Core Haptics allows you to fine-tune the tactile experience by adjusting various parameters. Let’s explore some of the key parameters you can modify.
5.1. Intensity
The intensity parameter controls the strength of the haptic feedback. You can make it subtle or powerful, depending on your app’s context.
swift let intensity = CHHapticEventParameter(parameterID: .hapticIntensity, value: 0.5) // Half intensity
5.2. Sharpness
Sharpness determines how sharp or smooth the haptic feedback feels. A high sharpness value results in a sharper, more defined sensation.
swift let sharpness = CHHapticEventParameter(parameterID: .hapticSharpness, value: 0.2) // Lower sharpness
5.3. Duration
The duration parameter specifies how long the haptic feedback lasts. Shorter durations are suitable for quick interactions, while longer durations can be used for sustained feedback.
swift let event = CHHapticEvent(eventType: .hapticTransient, parameters: [intensity, sharpness], relativeTime: 0, duration: 0.2) // 0.2 seconds duration
6. Implementing Core Haptics in Your App
Now that you’ve learned the basics of Core Haptics and how to create custom feedback, let’s explore how to integrate it into a practical example.
Example: Adding Haptic Feedback to a Button
Suppose you have a button in your app, and you want to provide haptic feedback when the user taps it. Here’s how you can achieve this:
swift @IBAction func buttonTapped(_ sender: UIButton) { // Create a haptic event let intensity = CHHapticEventParameter(parameterID: .hapticIntensity, value: 1.0) let sharpness = CHHapticEventParameter(parameterID: .hapticSharpness, value: 1.0) let event = CHHapticEvent(eventType: .hapticTransient, parameters: [intensity, sharpness], relativeTime: 0) // Create a haptic pattern let pattern = try? CHHapticPattern(events: [event], parameters: []) // Play the haptic pattern do { let player = try engine.makePlayer(with: pattern!) try player.start(atTime: CHHapticTimeImmediate) } catch { print("Error playing haptic pattern: \(error.localizedDescription)") } }
In this example, we’ve added a haptic event and pattern creation to the button’s action method. Now, when the button is tapped, the user will receive haptic feedback.
Conclusion
Haptic feedback is a powerful tool for enhancing the user experience in your iOS apps. With Core Haptics, you have the flexibility to create custom tactile feedback patterns that align with your app’s unique requirements. Whether you want to provide subtle cues, simulate continuous actions, or create entirely new tactile experiences, Core Haptics empowers you to do so with ease.
By implementing haptic feedback, you can make your app more engaging, accessible, and memorable, ultimately setting it apart in the competitive world of mobile app development. So, start exploring Core Haptics today, and take your iOS app’s user experience to the next level!
Table of Contents
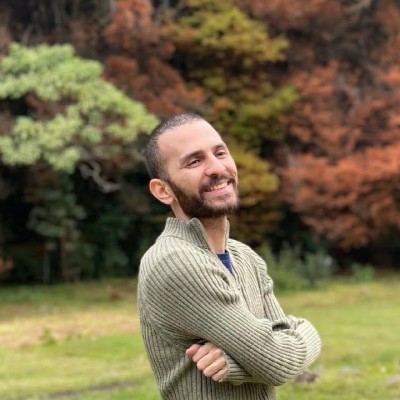
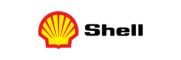