Exploring CoreML Model Deployment in iOS: On-Device and Cloud
In the ever-evolving landscape of iOS app development, integrating machine learning models has become increasingly popular. Apple’s CoreML framework empowers iOS developers to leverage the potential of machine learning seamlessly.
Table of Contents
Machine learning has transformed the way we interact with technology, and iOS app development is no exception. Apple’s CoreML framework empowers iOS developers to integrate machine learning models into their applications seamlessly. This opens up a world of possibilities, from enhancing user experiences to solving complex problems.
In this blog post, we will explore CoreML model deployment in iOS, focusing on two primary methods: on-device deployment and cloud-based deployment. We’ll walk through each approach, highlighting their strengths and use cases, and provide you with practical code samples to get you started.
1. Getting Started with CoreML
1.1 What is CoreML?
CoreML is Apple’s machine learning framework that allows developers to integrate trained machine learning models into their iOS and macOS applications. It provides a user-friendly and efficient way to incorporate AI capabilities, such as image recognition, natural language processing, and sentiment analysis, into your apps.
1.2 Supported Model Formats
CoreML supports various machine learning model formats, including:
- Apple’s .mlmodel format: This is a dedicated format for CoreML models and is the most straightforward to work with.
- ONNX (Open Neural Network Exchange): ONNX is an open standard format for representing machine learning models. You can convert ONNX models to CoreML format using available tools.
- TensorFlow and PyTorch models: With the help of conversion tools, you can bring models created in TensorFlow or PyTorch into the CoreML ecosystem.
1.3 Tools and Resources
Before diving into CoreML model deployment, it’s essential to familiarize yourself with the tools and resources available:
- Xcode: Apple’s integrated development environment (IDE) for iOS and macOS app development.
- CoreML Tools: A set of Python scripts provided by Apple for converting machine learning models to the .mlmodel format.
- Create ML: A tool within Xcode that enables developers to create and train custom machine learning models for specific tasks.
- Apple Developer Documentation: The official resource for CoreML documentation, including detailed guides and API references.
- With these tools in hand, you’re ready to explore CoreML model deployment.
2. On-Device Deployment with CoreML
On-device deployment involves running machine learning models directly on the user’s iOS device. This approach offers several advantages, such as low latency, offline functionality, and enhanced privacy.
2.1 Preparing Your Model
Before deploying a model on the user’s device, you need to prepare it. Here are the steps:
Step 1: Train and Export the Model
Train your machine learning model using your preferred framework, such as TensorFlow or PyTorch. Once the training is complete, export the model in a format compatible with CoreML, such as .mlmodel or ONNX.
Step 2: Convert the Model
If your model is not already in the .mlmodel format, you’ll need to use CoreML Tools or other conversion tools to convert it. This step ensures your model can be loaded and used within your iOS app.
2.2 Integrating CoreML into Your iOS App
Once your model is ready, you can integrate it into your iOS app using Swift or Objective-C. Here’s a simplified example of how to load and use a CoreML model for image recognition:
swift import CoreML // Load the CoreML model guard let model = try? YourModelClass(configuration: MLModelConfiguration()) else { fatalError("Failed to load the model.") } // Create a request to perform image recognition let request = YourModelClassRequest(model: model) // Prepare the input data (e.g., an image) let input = YourModelClassInput(/* input data */) // Perform the prediction do { let prediction = try request.prediction(input: input) // Access the prediction results print(prediction.outputLabel) } catch { print("Error making prediction: \(error)") }
In this code snippet, replace YourModelClass with the actual class name of your CoreML model. This code loads the model, prepares input data, and makes predictions.
2.3 Running the Model on Device
Once you’ve integrated the model into your app, you can deploy it to iOS devices through the App Store. Users can then interact with your app, which leverages the CoreML model for various tasks.
On-device deployment is advantageous when:
- Low Latency is Critical: For real-time tasks like image recognition, running the model on the device reduces latency and provides a snappy user experience.
- Privacy is a Concern: Since data stays on the device, there are fewer privacy and security concerns compared to cloud-based solutions.
- Offline Functionality is Required: Users can use the app and its machine learning features without an internet connection.
However, on-device deployment may have limitations in terms of model size and performance, especially for large and complex models.
3. Cloud-Based Deployment with CoreML
Cloud-based deployment involves running machine learning models on remote servers and making predictions through API requests. This approach offers scalability, flexibility, and the ability to update models without requiring app updates.
3.1 Benefits of Cloud-Based Deployment
Cloud-based deployment provides several advantages:
- Scalability: You can handle a large number of requests and users by scaling your server infrastructure.
- Model Updates: Easily update and improve your machine learning models on the server without requiring users to update the app.
- Lower Device Requirements: Complex models can be run on powerful servers, reducing the computational burden on user devices.
3.2 CoreML in the Cloud
To deploy CoreML models in the cloud, you’ll need a server infrastructure that can host and serve machine learning models. Here’s an overview of the process:
Step 1: Model Hosting
Host your CoreML model on a server that’s accessible via an API. This server can be set up using popular frameworks and libraries like Flask, Django, or FastAPI.
Step 2: API Integration
In your iOS app, integrate code to make HTTP requests to the server’s API. You can use libraries like Alamofire or URLSession for networking tasks.
Step 3: Making Predictions
When your app needs to make predictions, it sends input data to the server via API requests. The server processes the data, runs the CoreML model, and sends back the prediction results.
Here’s an example of how to make an API request for cloud-based CoreML predictions in Swift:
swift import Alamofire let apiUrl = "https://your-model-server.com/predict" let input = ["key1": "value1", "key2": "value2"] AF.request(apiUrl, method: .post, parameters: input, encoding: JSONEncoding.default) .validate() .responseJSON { response in switch response.result { case .success(let value): // Handle the prediction results print(value) case .failure(let error): print("Error: \(error)") } }
This code sends a POST request to the model server with input data and handles the prediction results.
3.3 Deploying a CoreML Model to the Cloud
Deploying a CoreML model to the cloud involves a few additional considerations:
Model Optimization:
Before deploying to the cloud, optimize your CoreML model for server-based inference. You may need to resize input images, batch multiple predictions for efficiency, and choose an appropriate server infrastructure.
Data Security:
Ensure secure data transmission between your app and the server using encryption and authentication mechanisms, especially if sensitive data is involved.
Model Versioning:
Implement a versioning system for your models to enable seamless updates without breaking existing functionality.
Cloud-based deployment is ideal when:
- Scalability is Essential: If your app serves a large user base or experiences unpredictable spikes in usage, cloud-based deployment can easily scale to meet demands.
- Frequent Model Updates are Required: For applications where model updates are frequent (e.g., recommendation systems), cloud deployment allows you to iterate quickly without waiting for app updates.
- Heavy Computational Requirements: Complex models that demand substantial computational resources are better suited for server-based deployment.
4. Comparing On-Device and Cloud Deployment
Both on-device and cloud-based deployment have their strengths and limitations. Let’s compare them in terms of key factors:
4.1 Performance Considerations
On-Device:
- Low latency due to local model execution.
- Suitable for real-time tasks like image recognition.
- Limited by device hardware, which may impact performance for complex models.
Cloud-Based:
- Performance depends on server infrastructure.
- Scalable for handling high request volumes.
- Better suited for computationally intensive models.
4.2 Security and Privacy
On-Device:
- Data remains on the user’s device, enhancing privacy.
- Limited risk of data breaches during transmission.
Cloud-Based:
- Data transmission between app and server requires strong security measures.
- Potential privacy concerns when data is sent to remote servers.
4.3 Offline vs. Online
On-Device:
- Works offline, making it suitable for applications without constant internet access.
- Provides consistent performance regardless of network conditions.
Cloud-Based:
- Requires internet connectivity for model predictions.
- Performance may vary based on network quality and latency.
5. Best Practices
When deploying CoreML models, consider the following best practices:
5.1 Model Optimization
Optimize your models for deployment to ensure efficient execution and minimal resource usage.
5.2 Error Handling
Implement robust error handling in your app to gracefully handle unexpected issues, such as server downtime or network errors.
5.3 Monitoring and Updates
Regularly monitor your deployed models and infrastructure for performance and security. Implement versioning to enable seamless updates when necessary.
Conclusion
CoreML empowers iOS developers to integrate machine learning models seamlessly, enhancing the capabilities and user experiences of their apps. Whether you choose on-device or cloud-based deployment, the choice depends on your specific use case and requirements. By understanding the strengths and limitations of each approach, you can make an informed decision and create AI-powered iOS apps that delight your users.
In this blog post, we’ve explored the world of CoreML model deployment, covering the essential steps and considerations for both on-device and cloud-based deployment. Armed with this knowledge, you can embark on your journey to harness the power of machine learning in iOS app development. Happy coding!
Table of Contents
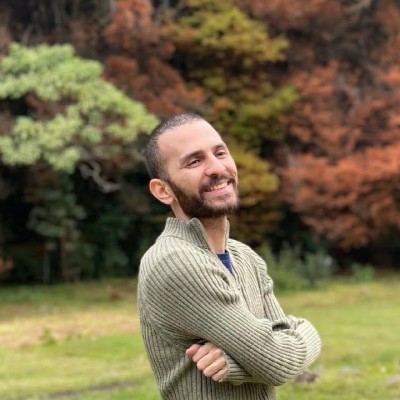
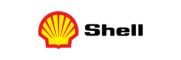