Working with Push Notifications in iOS: Firebase Cloud Messaging
Push notifications have become an integral part of our mobile app experiences. They keep users engaged, informed, and connected to the apps they love. For iOS developers, Firebase Cloud Messaging (FCM) is a robust and user-friendly platform to implement push notifications effortlessly. In this comprehensive guide, we will delve into the world of push notifications in iOS using Firebase Cloud Messaging, covering everything from setup to code samples.
Table of Contents
In this blog post, we’ll explore how to work with push notifications in iOS using Firebase Cloud Messaging. We’ll cover everything from setting up a Firebase project to sending customized notifications and handling advanced topics like silent push notifications and analytics.
So, let’s dive in and learn how to leverage the capabilities of Firebase Cloud Messaging to enhance the user experience of your iOS app.
1. Getting Started with Firebase
Setting up a Firebase Project
To begin working with Firebase Cloud Messaging, you need to have a Firebase project. If you haven’t already created one, follow these steps:
- Go to the Firebase Console.
- Click on “Add Project.”
- Follow the on-screen instructions to set up your project. You will need to provide a project name and choose a project ID.
- Once your project is created, click on it in the Firebase Console.
1.1. Adding Firebase to Your iOS App
With your Firebase project set up, it’s time to integrate Firebase into your iOS app. Here’s how:
- In the Firebase Console, click on “Add App” and select the iOS platform.
- Register your app by providing the iOS bundle ID. This should match the bundle ID of your Xcode project.
- Download the GoogleService-Info.plist file and add it to your Xcode project root directory.
- In your Xcode project, open the AppDelegate.swift file and add the following import statement at the top of the file:
swift import Firebase
5. In the application:didFinishLaunchingWithOptions method of your AppDelegate, initialize Firebase using the following code:
swift FirebaseApp.configure()
Now, your iOS app is connected to Firebase, and you’re ready to implement push notifications.
2. Enabling Push Notifications
Before you can send push notifications to your iOS app, you need to enable push notifications capabilities in your Xcode project.
- In Xcode, open your project and select the target for your app.
- Go to the “Signing & Capabilities” tab.
- Click the “+ Capability” button and search for “Push Notifications.” Click “Add” to enable push notifications for your app.
- Also, add the “Background Modes” capability and enable “Remote notifications.”
Your app is now configured to receive push notifications. Let’s move on to registering for push notifications.
3. Registering for Push Notifications
3.1. Requesting User Permission
Before your app can send push notifications to the user’s device, you need to request their permission. This is typically done when the app is launched for the first time. Here’s how you can request permission to send push notifications:
In your AppDelegate.swift file, add the following code to the application:didFinishLaunchingWithOptions method:
swift UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .sound, .badge]) { (granted, error) in if granted { print("Permission granted for push notifications") } else { print("Permission denied for push notifications") } }
This code requests authorization for alert, sound, and badge notifications. You can customize the options based on your app’s requirements.
3.2. Handling User Responses
To handle the user’s response to the permission request, implement the following method in your AppDelegate.swift file:
swift func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) { let token = deviceToken.map { String(format: "%02.2hhx", $0) }.joined() print("Device token: \(token)") } func application(_ application: UIApplication, didFailToRegisterForRemoteNotificationsWithError error: Error) { print("Failed to register for remote notifications: \(error.localizedDescription)") }
The didRegisterForRemoteNotificationsWithDeviceToken method will be called when the registration is successful, providing you with the device token that you can use to send notifications.
4. Sending Notifications from Firebase Console
Now that your app is set up to receive push notifications and has obtained the device token, you can send notifications from the Firebase Console. Here’s how:
- Go to the Firebase Console and select your project.
- In the left sidebar, click on “Cloud Messaging.”
- Click on the “Send a message” button.
- In the message composer, select the target audience for your notification. You can send it to a specific app version, user segment, or topic.
- Compose your notification message, including the title and body.
- Click the “Next” button to set additional options, such as scheduling the message or adding custom data to it.
- Review your message and click “Publish” to send the notification.
Your iOS app should receive the notification, and you’ll see it displayed on the device’s screen.
5. Customizing Push Notifications
While sending basic notifications is useful, you can enhance your user experience by customizing push notifications and handling user interactions. Here’s how you can do that:
5.1. Adding Data to Notifications
Push notifications can carry custom data along with the message. This data can be used to provide additional context or trigger specific actions in your app. To send data along with your notification, you can include a data payload when sending a message from the Firebase Console. Here’s an example of a JSON payload:
json { "to": "<device-token>", "data": { "customKey": "customValue", "anotherKey": "anotherValue" } }
In your iOS app, you can retrieve this data in the didReceiveRemoteNotification method of your AppDelegate:
swift func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable: Any]) { if let customValue = userInfo["customKey"] as? String { print("Received customValue: \(customValue)") } // Handle other data as needed }
5.2. Handling Notification Actions
iOS allows you to add actions to your push notifications, which users can interact with directly from the notification banner or lock screen. To implement this, you need to create a notification category and attach it to your notification.
Here’s a high-level overview of the steps:
Define your notification category and actions in your AppDelegate.swift file:
swift import UserNotifications // Define the notification category let category = UNNotificationCategory(identifier: "MyNotificationCategory", actions: [], intentIdentifiers: [], options: []) // Register the notification category UNUserNotificationCenter.current().setNotificationCategories([category])
When sending a notification from the Firebase Console, include the category ID in the payload:
json { "to": "<device-token>", "data": { "customKey": "customValue", "category": "MyNotificationCategory" } }
In your AppDelegate.swift file, implement the userNotificationCenter(_:didReceive:withCompletionHandler:) method to handle user interactions with notifications:
swift func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) { if response.actionIdentifier == UNNotificationDefaultActionIdentifier { // The user tapped the notification without selecting an action } else { // Handle custom actions here } completionHandler() }
By implementing custom actions, you can give users the ability to perform specific actions directly from your push notifications.
6. Advanced Topics
6.1. Push Notification Analytics
Understanding how users engage with your push notifications is crucial for optimizing your messaging strategy. Firebase Cloud Messaging provides analytics that can help you track notification delivery, open rates, and user engagement. You can access this data in the Firebase Console to gain insights into the effectiveness of your notifications.
6.2. Silent Push Notifications
Silent push notifications are notifications that don’t display a message to the user but can trigger background tasks in your app. These are useful for tasks like syncing data, updating content, or performing other background operations without disturbing the user. You can configure your app to handle silent push notifications by specifying the content-available key in the notification payload.
6.3. Handling Remote Notifications
In some cases, your app may not be running when a push notification arrives. In such scenarios, your app can still receive and handle the notification by implementing the application:didReceiveRemoteNotification:fetchCompletionHandler method in your AppDelegate. This allows your app to perform necessary actions even when it’s not in the foreground.
Conclusion
Firebase Cloud Messaging is a powerful tool for implementing push notifications in iOS apps. It provides a user-friendly interface, seamless integration with Firebase projects, and advanced features for customizing and analyzing notifications. By following the steps outlined in this guide and exploring advanced topics, you can take full advantage of push notifications to engage and delight your app’s users.
Incorporating push notifications effectively can significantly enhance the user experience, increase user retention, and drive user engagement in your iOS app. So, start leveraging Firebase Cloud Messaging today to keep your users informed and engaged with timely notifications. Your users will thank you, and your app will thrive as a result.
Table of Contents
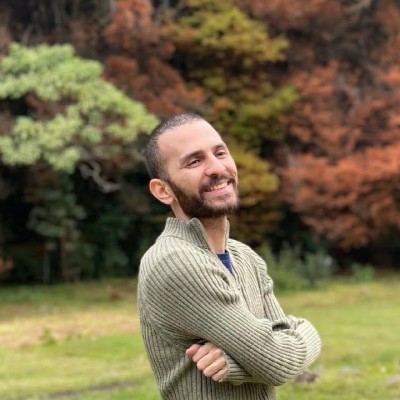
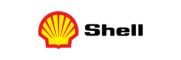