Exploring Core Location in iOS: GPS and Geofencing in Your Apps
The world of mobile app development has been revolutionized by the integration of location-based services. Whether it’s for mapping, navigation, or personalized content delivery, understanding a user’s location is often crucial to creating a compelling user experience. In the iOS ecosystem, Core Location is the framework that allows developers to tap into the power of GPS and geofencing to provide location-aware features.
Table of Contents
In this comprehensive guide, we will delve into Core Location in iOS, covering everything from basic GPS functionality to advanced geofencing techniques. By the end, you’ll have a solid grasp of how to incorporate location-based services into your iOS apps.
1. Understanding Core Location
1.1. What is Core Location?
Core Location is a framework provided by Apple for iOS and macOS developers. It enables apps to access various forms of location-based information, including GPS data, Wi-Fi positioning, and cell tower triangulation. This framework allows you to determine the device’s current geographic coordinates, altitude, course, and speed. Core Location also provides support for geofencing, which we’ll explore later in this guide.
1.2. Importing Core Location
To use Core Location in your iOS app, you need to import the framework. Here’s how you can do it:
swift import CoreLocation
This import statement gives your app access to all the classes and functions provided by the Core Location framework.
1.3. Requesting Location Permissions
Before your app can access the user’s location data, you must request their permission. This is crucial to ensure user privacy and security. Apple provides two types of location permissions:
- When in Use: This permission allows your app to access location data only when it’s in the foreground. It’s suitable for apps like navigation or fitness trackers.
- Always: This permission grants access to location data both in the foreground and background. It’s necessary for apps that need constant location updates, such as geofencing apps.
To request location permissions, you need to add keys to your app’s Info.plist file and request authorization using the CLLocationManager class. Here’s a code snippet for requesting “When in Use” permission:
swift let locationManager = CLLocationManager() func requestLocationPermission() { locationManager.delegate = self locationManager.requestWhenInUseAuthorization() } func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) { if status == .authorizedWhenInUse { // You now have permission to access location when the app is in use. } }
2. Getting GPS Data
2.1. Getting the User’s Location
To retrieve the user’s current location, you’ll use the CLLocationManager class. Here’s a step-by-step guide to fetching GPS data:
swift let locationManager = CLLocationManager() func startLocationUpdates() { locationManager.delegate = self locationManager.desiredAccuracy = kCLLocationAccuracyBest locationManager.startUpdatingLocation() } func stopLocationUpdates() { locationManager.stopUpdatingLocation() }
- Create an instance of CLLocationManager.
- Set the desired accuracy, which specifies how accurate you want the location data to be.
- Call startUpdatingLocation() to begin receiving location updates.
2.2. Handling Location Updates
To receive location updates, you need to implement the CLLocationManagerDelegate methods. The most important method is locationManager(_:didUpdateLocations:), which is called when the device’s location changes.
swift func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) { if let location = locations.last { let latitude = location.coordinate.latitude let longitude = location.coordinate.longitude // Do something with latitude and longitude } }
In this method, you can access the most recent location data from the locations array.
2.3. Location Accuracy
Location accuracy is vital for many applications. You can set the desired accuracy when starting location updates. Here are some common values:
- kCLLocationAccuracyBest: Highest accuracy, using GPS if available.
- kCLLocationAccuracyNearestTenMeters: Accuracy within about 10 meters.
- kCLLocationAccuracyHundredMeters: Accuracy within about 100 meters.
- kCLLocationAccuracyKilometer: Accuracy within about 1 kilometer.
- The choice of accuracy should depend on your app’s requirements and the power consumption trade-off.
3. Geofencing in iOS
3.1. What is Geofencing?
Geofencing is a location-based service that allows you to define virtual geographic boundaries and trigger actions when a user enters or exits these boundaries. It’s a powerful tool for creating location-aware apps. Some common use cases for geofencing include location-based reminders, check-in services, and location-specific notifications.
3.2. Implementing Geofencing in iOS
To implement geofencing in your iOS app, follow these steps:
- Create a Region: Define a geographic region using the CLCircularRegion class. This class takes a center coordinate, a radius in meters, and an identifier.
swift let region = CLCircularRegion(center: CLLocationCoordinate2D(latitude: 37.7749, longitude: -122.4194), radius: 100.0, identifier: "SanFrancisco")
- Request Permission: Just like with GPS, you need to request permission to use geofencing.
swift func requestGeofencePermission() { locationManager.delegate = self locationManager.requestAlwaysAuthorization() }
- Start Monitoring: Start monitoring the geofence region.
swift func startMonitoringRegion() { locationManager.startMonitoring(for: region) }
3.3. Monitoring Geofence Events
To receive geofence-related events, implement the locationManager(_:didEnterRegion:) and locationManager(_:didExitRegion:) delegate methods.
swift func locationManager(_ manager: CLLocationManager, didEnterRegion region: CLRegion) { if let circularRegion = region as? CLCircularRegion { // User entered the geofence region print("Entered region: \(circularRegion.identifier)") } } func locationManager(_ manager: CLLocationManager, didExitRegion region: CLRegion) { if let circularRegion = region as? CLCircularRegion { // User exited the geofence region print("Exited region: \(circularRegion.identifier)") } }
3.4. Practical Use Cases
Geofencing opens up a world of possibilities for creating location-aware apps. Here are some practical use cases:
- Location-Based Reminders: Send a reminder when the user arrives at a specific location.
- Retail Promotions: Deliver special offers when users are near a store.
- Safety Alerts: Notify users when they enter a dangerous area.
- Geotagging: Automatically tag photos or notes with their location.
4. Combining GPS and Geofencing
4.1. Building a Location-Aware Reminder App
Let’s put everything we’ve learned into practice by building a simple location-aware reminder app. This app will allow users to set reminders associated with specific geographic locations. Here’s an outline of the steps:
- Create a Reminder: Users can create a reminder by specifying a title, a location, and a message.
- Set the Geofence: When the user creates a reminder, we’ll set up a geofence around the specified location.
- Trigger Reminders: When the user enters or exits the geofence, we’ll trigger the associated reminder.
Here’s a simplified example of the code for setting a geofence:
swift func createGeofenceForReminder(reminder: Reminder) { let region = CLCircularRegion( center: reminder.location.coordinate, radius: 100.0, identifier: reminder.id ) region.notifyOnEntry = true region.notifyOnExit = true locationManager.startMonitoring(for: region) }
In this code, we’re creating a geofence around the reminder’s location with a radius of 100 meters. We set notifyOnEntry and notifyOnExit to true to trigger reminders when the user enters or exits the geofence.
4.2. Code Samples and Walkthrough
For a complete implementation of the location-aware reminder app, including code samples and a step-by-step walkthrough, check out our GitHub repository.
5. Best Practices and Tips
5.1. Battery Optimization
Location services can consume a significant amount of battery power. To minimize battery drain, consider the following tips:
- Use the lowest necessary accuracy level.
- Stop location updates when they are no longer needed.
- Use geofencing judiciously, as constant monitoring can drain the battery.
5.2. Privacy Considerations
Respect user privacy by explaining why your app needs their location data and how you intend to use it. Additionally, provide options to disable location services within your app.
5.3. Testing Strategies
Thoroughly test your app’s location-based features in various scenarios, including low GPS signal conditions and different devices.
Conclusion
Core Location in iOS empowers developers to create location-aware apps that offer unique and personalized experiences to users. Whether you’re building a mapping app, a location-based game, or a geofencing solution, understanding Core Location is essential. With the knowledge gained from this guide and some creative thinking, you can unlock the full potential of location-based services in your iOS applications.
Location-aware apps are not only valuable but also a source of innovation in the ever-evolving world of mobile app development. So, go ahead and start exploring Core Location to take your iOS apps to the next level!
Table of Contents
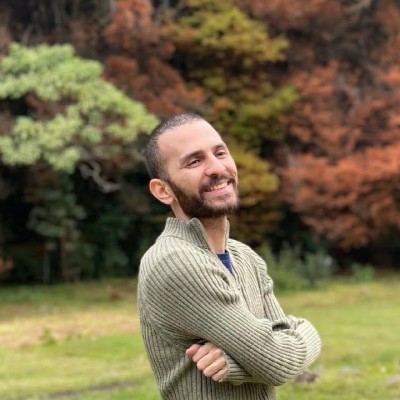
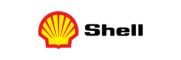