Implementing Drag and Drop in iOS: Enhancing User Interaction
In the ever-evolving world of mobile app development, user interaction is a crucial aspect of creating an engaging and user-friendly experience. One way to improve user interaction in your iOS app is by implementing drag and drop functionality. Drag and drop not only adds a layer of interactivity but also simplifies complex actions, making your app more intuitive and enjoyable to use.
Table of Contents
In this comprehensive guide, we will explore how to implement drag and drop in iOS apps, step-by-step. You’ll learn the fundamental concepts, best practices, and get hands-on with code samples to create a seamless user experience.
1. Understanding Drag and Drop in iOS
Before diving into the implementation details, let’s grasp the basic concepts of drag and drop in iOS. Apple introduced this feature to make it easier for users to interact with on-screen elements. Drag and drop allows users to pick up an item, move it around the screen, and drop it onto another location or container.
In iOS, drag and drop can be used for a variety of purposes, such as rearranging elements, transferring data between apps, or enhancing user creativity in drawing or design applications. It provides a natural and intuitive way for users to interact with digital content.
1.1. Dragging: The Starting Point
Dragging is the action of picking up an item and initiating the drag operation. In iOS, users can initiate a drag operation by pressing and holding on an item until it starts to move. During this phase, the item being dragged typically follows the user’s finger or cursor.
1.2. Dropping: The Endpoint
Dropping is the action of releasing the dragged item onto a target location or container. This is where the drag operation is completed, and the item is placed in its new position. Dropping can trigger various actions depending on the app’s functionality, such as rearranging items or processing data.
Now that we understand the core concepts, let’s proceed with the steps to implement drag and drop in your iOS app.
Step 1: Setting Up Your Xcode Project
To begin implementing drag and drop in your iOS app, open Xcode and create a new project or open an existing one. Ensure that your project is compatible with the iOS version you are targeting, as some drag and drop features may vary across iOS versions.
Step 1.1: Enable Drag and Drop Interaction
In Xcode, open your project’s settings and select the target app. Under the “Signing & Capabilities” tab, make sure the “Drag and Drop” capability is enabled. This allows your app to interact with the drag and drop system.
Step 1.2: Add a UIView to Your Interface
In your app’s interface, add a UIView that you want to make draggable. This UIView can represent an image, a piece of text, or any other item you want users to interact with.
Step 2: Making UIView Draggable
Now that you’ve set up your Xcode project and added a UIView to your interface, it’s time to make the UIView draggable. To do this, you’ll need to implement gesture recognizers and delegate methods.
Step 2.1: Implement Gesture Recognizers
Gesture recognizers are essential for detecting user interactions. In this case, we’ll use a UIPanGestureRecognizer to track the movement of the UIView when the user drags it. Here’s how to implement it:
swift import UIKit class DraggableView: UIView { private var initialCenter: CGPoint? override func awakeFromNib() { super.awakeFromNib() // Add a pan gesture recognizer to the UIView let panGesture = UIPanGestureRecognizer(target: self, action: #selector(handlePan(_:))) self.addGestureRecognizer(panGesture) } @objc private func handlePan(_ gestureRecognizer: UIPanGestureRecognizer) { switch gestureRecognizer.state { case .began: // Save the initial center position initialCenter = self.center case .changed: guard let initialCenter = initialCenter else { return } // Calculate the new center based on the pan gesture let translation = gestureRecognizer.translation(in: self) self.center = CGPoint(x: initialCenter.x + translation.x, y: initialCenter.y + translation.y) case .ended: // Perform any actions you need when the drag ends // For example, check if the UIView was dropped in a valid location // and trigger appropriate actions. break default: break } } }
In the code above, we’ve created a custom DraggableView class that inherits from UIView. We’ve added a UIPanGestureRecognizer to the view, which allows users to drag it around the screen.
Step 2.2: Implement Drag and Drop Delegate Methods
To enable the UIView to participate in drag and drop interactions, you need to implement delegate methods. These methods provide information about the draggable item and specify how it behaves during drag and drop operations.
In your view controller or appropriate delegate class, conform to the UIDragInteractionDelegate and UIDropInteractionDelegate protocols:
swift import UIKit class ViewController: UIViewController, UIDragInteractionDelegate, UIDropInteractionDelegate { // ... }
Now, implement the required delegate methods to specify the behavior of the drag and drop interaction for your UIView:
Implementing Drag Interaction Delegate Methods
swift func dragInteraction(_ interaction: UIDragInteraction, itemsForBeginning session: UIDragSession) -> [UIDragItem] { // Create a UIDragItem representing the dragged object let provider = NSItemProvider(object: "Dragged Item" as NSString) let item = UIDragItem(itemProvider: provider) return [item] }
In the itemsForBeginning method, you create a UIDragItem that represents the item being dragged. You can customize the item’s data and appearance according to your app’s requirements.
Implementing Drop Interaction Delegate Methods
swift func dropInteraction(_ interaction: UIDropInteraction, performDrop session: UIDropSession) { // Handle the drop operation here // You can access the dropped items and perform actions accordingly for item in session.items { item.itemProvider.loadObject(ofClass: NSString.self) { (object, error) in if let string = object as? NSString { DispatchQueue.main.async { // Handle the dropped string data // For example, update your interface with the dropped content } } } } }
In the performDrop method, you handle the drop operation. You can access the dropped items and their data, then perform actions based on the dropped content.
Step 3: Testing Your Drag and Drop Implementation
With the drag and drop code in place, it’s time to test your implementation. Build and run your app on a simulator or physical device to ensure that the drag and drop functionality works as expected.
During testing, pay attention to the following:
- Smoothness: Ensure that the dragging motion is smooth and follows the user’s finger or cursor accurately.
- Interaction: Test the drop interaction to confirm that the dropped items behave as intended.
- User Feedback: Consider adding visual feedback, such as animations or highlighting, to enhance the user experience during drag and drop.
Step 4: Enhancing User Experience
While the basic drag and drop functionality is now implemented in your iOS app, you can take several steps to enhance the user experience further.
4.1: Customization
Customize the appearance and behavior of the dragged item by modifying the UIDragItem object in the itemsForBeginning method. You can change the preview image, add metadata, or apply animations to make the drag-and-drop interaction more engaging.
4.2: Feedback and Animation
Provide visual and auditory feedback during the drag and drop process to inform users about the current state of the operation. This can include changing the appearance of the dragged item, highlighting valid drop zones, or playing sounds.
4.3: Undo and Redo
Consider adding undo and redo functionality to allow users to revert their drag and drop actions if they make a mistake. This can be especially useful in productivity or creative apps.
4.4: Accessibility
Ensure that your drag and drop implementation is accessible to all users. Implement voiceover support, provide clear labels and hints, and ensure that users with disabilities can perform drag and drop actions using assistive technologies.
Step 5: Handling Multiple Items
In some cases, your app may need to support dragging and dropping multiple items simultaneously. To achieve this, you can modify your drag and drop implementation to handle multiple UIDragItem objects and manage their interactions independently.
Here’s a simplified example of handling multiple items:
swift func dragInteraction(_ interaction: UIDragInteraction, itemsForBeginning session: UIDragSession) -> [UIDragItem] { var dragItems: [UIDragItem] = [] // Create and add multiple UIDragItem objects to the array for itemData in dataToDrag { let provider = NSItemProvider(object: itemData as NSString) let item = UIDragItem(itemProvider: provider) // Customize each item as needed item.localObject = itemData dragItems.append(item) } return dragItems }
In this example, we loop through an array of data to create multiple UIDragItem objects, each representing a different item. This allows users to drag and drop multiple items at once.
Conclusion
Implementing drag and drop functionality in your iOS app can significantly enhance user interaction and make your app more intuitive and user-friendly. By following the steps outlined in this guide, you can create a seamless drag and drop experience that meets the needs of your users.
Remember to customize the drag and drop behavior to suit your app’s specific requirements and consider accessibility and user feedback to ensure a delightful user experience. Whether you’re building a productivity app, a game, or a creative tool, drag and drop can be a powerful addition to your iOS app’s toolkit.
Start experimenting with drag and drop in your iOS app today and unlock a world of interactive possibilities that will delight your users and set your app apart from the competition.
Table of Contents
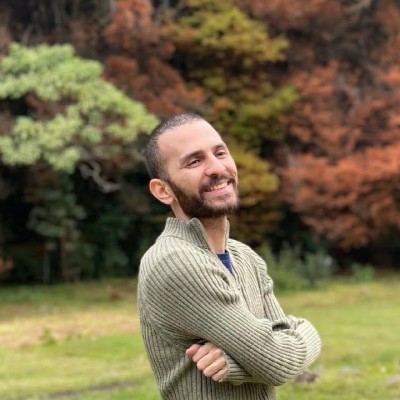
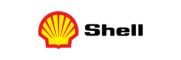