Implementing Core ML in iOS: Machine Learning for Image Classification
In today’s rapidly evolving tech landscape, machine learning is no longer a distant concept confined to research labs. It has permeated our everyday lives, from recommendation systems on streaming platforms to virtual personal assistants. Mobile app development is no exception to this trend, and with the power of Core ML, iOS developers can seamlessly integrate machine learning capabilities into their apps. In this blog, we’ll delve into the fascinating realm of image classification using Core ML in iOS.
Table of Contents
1. Why Image Classification?
Image classification is a fundamental task in computer vision, and it has numerous practical applications. From identifying objects in photos to enabling augmented reality experiences, image classification forms the backbone of many cutting-edge technologies. By understanding how to implement image classification in your iOS apps, you’ll gain valuable insights into the broader world of machine learning.
2. Prerequisites
Before we dive into the implementation details, let’s ensure you have the necessary prerequisites in place:
2.1. Xcode
Make sure you have Xcode, Apple’s integrated development environment (IDE), installed on your Mac. You can download it from the Mac App Store if you haven’t already.
2.2. Basic iOS Development Knowledge
Familiarity with iOS app development using Swift is crucial for this tutorial. If you’re new to iOS development, consider taking introductory courses or reading Apple’s official documentation to get started.
2.3. Machine Learning Model
You’ll need a pre-trained machine learning model for image classification. For this tutorial, we’ll use a model available in the Core ML model zoo, but you can also train your custom model and convert it to the Core ML format.
3. Getting Started with Core ML
Now that we have our prerequisites covered let’s begin implementing image classification using Core ML in iOS. Here are the steps we’ll follow:
Step 1: Create a New Xcode Project
Open Xcode and create a new iOS project. Choose the “Single View App” template as a starting point.
Step 2: Add the Core ML Model to Your Project
Download a pre-trained Core ML model for image classification. You can find various models on the internet, or you can use the Core ML model zoo. Once you have the model, drag and drop it into your Xcode project.
Step 3: Import Core ML and Vision Frameworks
In your Xcode project, open the Swift file where you want to perform image classification. Import the Core ML and Vision frameworks at the top of the file:
swift import CoreML import Vision
These frameworks provide the tools and APIs you need to work with machine learning models and perform image classification.
Step 4: Load the Core ML Model
In your Swift file, load the Core ML model you added to your project. Make sure to replace “YourModelName” with the name of your model file:
swift guard let model = try? VNCoreMLModel(for: YourModelName().model) else { fatalError("Failed to load Core ML model") }
Step 5: Set Up Image Classification
Now, you need to create an image classification request. This request will process the input image and provide the classification results. Add the following code:
swift let classificationRequest = VNCoreMLRequest(model: model) { request, error in guard let results = request.results as? [VNClassificationObservation], let topResult = results.first else { fatalError("Failed to perform image classification") } let className = topResult.identifier let confidence = topResult.confidence print("Class: \(className), Confidence: \(confidence)") }
Step 6: Perform Image Classification
With the classification request set up, you can now use it to classify images. You’ll typically perform image classification when the user selects or captures an image. Here’s an example of how to use the classification request:
swift // Assuming you have a UIImage named 'image' to classify if let ciImage = CIImage(image: image) { let handler = VNImageRequestHandler(ciImage: ciImage) do { try handler.perform([classificationRequest]) } catch { print("Failed to perform image classification: \(error)") } }
Step 7: Display the Results
Finally, you can display the classification results in your app’s user interface. You might want to show the top predicted class and its confidence score in a label or other UI element.
Congratulations! You’ve now implemented image classification using Core ML in your iOS app. This is just the beginning, as you can explore various enhancements and optimizations, such as real-time classification, custom models, and post-processing of classification results.
4. Tips and Best Practices
Before we wrap up, let’s explore some tips and best practices for working with Core ML in iOS:
4.1. Model Size and Performance
Consider the size of the Core ML model you’re using. Larger models may consume more memory and CPU resources, impacting app performance. Be mindful of your app’s target devices and choose models that strike the right balance between accuracy and resource usage.
4.2. Real-Time Classification
If your app requires real-time image classification, optimize your code and model to achieve low latency. You might need to implement techniques like model quantization to reduce inference time.
4.3. Error Handling
Always handle errors gracefully when working with Core ML and Vision frameworks. Machine learning models can fail for various reasons, such as incompatible inputs or model corruption. Implement robust error handling to ensure your app remains stable.
4.4. User Experience
Consider the user experience when implementing image classification. Provide feedback to the user while the classification is in progress, and present the results in a clear and intuitive manner.
4.5. Model Updates
Keep an eye on model updates and improvements. Machine learning models evolve, and newer versions might offer better accuracy or smaller file sizes. Regularly check for updates to stay up-to-date with the latest advancements.
Conclusion
Implementing Core ML in iOS for image classification opens up a world of possibilities for your app development projects. Whether you’re building a photo recognition app, an augmented reality application, or a creative image filter tool, integrating machine learning can add significant value and enhance user experiences. With the steps, code samples, and best practices outlined in this guide, you’re well on your way to harnessing the power of Core ML for image classification in your iOS apps. Happy coding!
Table of Contents
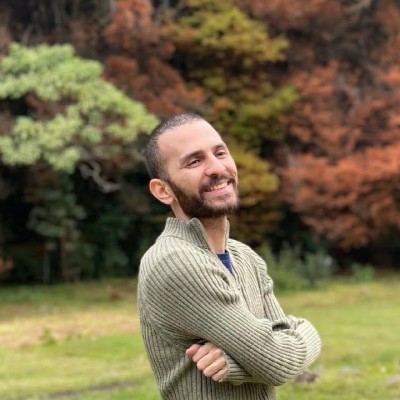
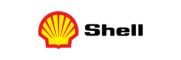