Introduction to Metal in iOS: High-Performance Graphics and Compute
In the ever-evolving world of mobile technology, iOS continues to set the benchmark for excellence. Behind its sleek user interface and seamless user experience lies an impressive array of technologies that make it all possible. Among these, Metal stands out as a powerful framework for high-performance graphics and compute tasks.
Table of Contents
If you’re an iOS developer looking to create visually stunning and responsive applications, understanding Metal is essential. This blog will serve as your comprehensive introduction to Metal in iOS, covering everything from the basics to advanced concepts.
1. What is Metal?
Metal is Apple’s low-level graphics and compute API, designed to harness the full power of the GPU (Graphics Processing Unit) in iOS devices. It was introduced in iOS 8 and has since become a fundamental component of Apple’s ecosystem for developing high-performance apps and games.
1.1. The Need for Metal
Before Metal, iOS developers primarily used OpenGL ES for graphics rendering. While OpenGL ES was powerful, it had some limitations. It was an aging technology, not fully integrated with iOS, and it suffered from performance overhead. Metal was Apple’s answer to these challenges.
Metal provides developers with direct access to the GPU, allowing for efficient graphics and compute operations. This direct control results in reduced overhead and improved performance, making it the go-to choice for demanding applications.
2. Metal vs. OpenGL ES
To better appreciate the capabilities of Metal, let’s compare it to its predecessor, OpenGL ES.
2.1. Performance
One of the primary reasons for Metal’s existence is its superior performance. Metal significantly reduces CPU overhead, making it ideal for complex graphics and compute tasks. This performance boost is particularly noticeable in games and applications that require real-time rendering and responsiveness.
2.2. Efficiency
Metal is designed with efficiency in mind. It minimizes the GPU’s idle time, ensuring that the hardware is utilized to its maximum potential. This results in smoother animations, faster load times, and improved battery life, all of which contribute to a better user experience.
2.3. Integration
Unlike OpenGL ES, Metal is tightly integrated into the iOS ecosystem. It seamlessly works with other iOS technologies, such as Core Animation and Core Image, making it easier to build cohesive and feature-rich applications.
2.4. Modern API
OpenGL ES, while powerful, was showing its age. Metal, on the other hand, is a modern API that takes advantage of the latest GPU features and capabilities. This allows developers to create cutting-edge graphics and effects that were previously out of reach.
3. Getting Started with Metal
Now that we’ve established Metal’s importance and advantages, let’s dive into how you can get started with Metal development in iOS.
3.1. Setting Up Xcode
Before you can start using Metal, you need to set up your development environment. Xcode is the official integrated development environment (IDE) for iOS app development, and it includes all the tools you need for Metal development.
- Install Xcode: If you haven’t already, download and install Xcode from the Mac App Store.
- Create a New Project: Open Xcode and create a new project. Choose the appropriate template for your application, whether it’s a game, graphics app, or something else.
- Import Metal: To use Metal in your project, you’ll need to import the Metal framework. You can do this by adding import Metal at the top of your Swift or Objective-C source files.
3.2. Understanding the Metal Basics
Before diving into coding, it’s essential to understand some fundamental concepts of Metal.
3.3. Metal Device
A Metal device represents the GPU on the iOS device. You can obtain a reference to the default GPU using the MTLCreateSystemDefaultDevice() function.
swift let device = MTLCreateSystemDefaultDevice()
3.4. Metal Command Queue
The command queue is where you submit work for the GPU to execute. It’s responsible for managing the order of commands and ensuring that they are executed efficiently.
swift let commandQueue = device.makeCommandQueue()
3.5. Metal Render Pipeline
The render pipeline is a crucial component for rendering graphics. It defines how vertices and fragments are processed and allows you to specify shaders and rendering configurations.
swift let pipelineDescriptor = MTLRenderPipelineDescriptor() // Configure the pipeline descriptor let pipelineState = try! device.makeRenderPipelineState(descriptor: pipelineDescriptor)
3.6. Metal Texture
Textures are images used in graphics rendering. Metal provides various ways to create and manipulate textures, making it flexible for different use cases.
swift let textureDescriptor = MTLTextureDescriptor.texture2DDescriptor( pixelFormat: .rgba8Unorm, width: 512, height: 512, mipmapped: true ) let texture = device.makeTexture(descriptor: textureDescriptor)
4. Drawing with Metal
Now that you have a basic understanding of Metal’s core concepts, let’s explore how to draw a simple shape using Metal.
4.1. Creating a Metal View
To render graphics with Metal, you’ll need a Metal view. This view subclass is responsible for displaying your graphics on the screen. You can create one in your storyboard or programmatically.
swift let metalView = MetalView(frame: view.bounds, device: device) view.addSubview(metalView)
4.2. Implementing MetalView
Next, you’ll need to implement the MetalView class. This class should adopt the MTKViewDelegate protocol and override its methods for rendering.
swift class MetalView: MTKView { // Implement MTKViewDelegate methods here }
4.3. Rendering a Simple Triangle
Let’s render a basic triangle using Metal. We’ll define the vertices, create a buffer to hold the vertex data, and set up the render pipeline.
swift // Define triangle vertices let vertices: [Float] = [ 0.0, 0.5, 0.0, -0.5, -0.5, 0.0, 0.5, -0.5, 0.0 ] // Create a buffer to hold vertex data let vertexBuffer = device.makeBuffer( bytes: vertices, length: vertices.count * MemoryLayout<Float>.size, options: [] ) // Set up the render pipeline let pipelineDescriptor = MTLRenderPipelineDescriptor() // Configure the pipeline descriptor let pipelineState = try! device.makeRenderPipelineState(descriptor: pipelineDescriptor)
In your MetalView class, implement the draw(in:) method to perform the rendering:
swift func draw(in view: MTKView) { guard let drawable = view.currentDrawable, let renderPassDescriptor = view.currentRenderPassDescriptor else { return } // Create a command buffer let commandBuffer = commandQueue.makeCommandBuffer() // Create a render command encoder let renderEncoder = commandBuffer?.makeRenderCommandEncoder(descriptor: renderPassDescriptor) // Set the render pipeline state renderEncoder?.setRenderPipelineState(pipelineState) // Set the vertex buffer renderEncoder?.setVertexBuffer(vertexBuffer, offset: 0, index: 0) // Draw the triangle renderEncoder?.drawPrimitives(type: .triangle, vertexStart: 0, vertexCount: 3) // End encoding and present the drawable renderEncoder?.endEncoding() commandBuffer?.present(drawable) commandBuffer?.commit() }
4.4. Running the App
With the above code in place, you should be able to run your Metal-based app and see a simple triangle rendered on the screen.
5. Metal Shaders
At the heart of Metal’s power lies the ability to use shaders for advanced graphics rendering. Shaders are small programs that run on the GPU and define how vertices and fragments are processed.
5.1. Types of Shaders
Metal supports two main types of shaders:
- Vertex Shaders: These shaders manipulate the positions of vertices in 3D space. They are responsible for transforming vertex data and passing it to the next stage.
- Fragment Shaders: Fragment shaders, also known as pixel shaders, determine the color of each pixel on the screen. They can apply textures, lighting, and other effects.
5.2. Writing Shaders
Shaders in Metal are typically written in the Metal Shading Language (MSL). MSL is C-like and designed specifically for high-performance GPU programming. Here’s a simple example of a vertex shader in MSL:
metal vertex VertexOut basic_vertex(VertexIn in [[stage_in]]) { VertexOut out; out.position = in.position; return out; }
In this example, the vertex shader simply passes the position of the vertex through to the next stage.
5.3. Using Shaders in Metal
To use shaders in Metal, you’ll need to:
- Compile Shaders: Metal shaders need to be compiled before they can be used in your app. This can be done using the MTLLibrary and MTLFunction classes.
- Set Shaders in the Pipeline: Once compiled, you’ll need to set your shaders in the render pipeline. This is done by creating a pipeline state that references your shader functions.
- Invoke Shaders: Finally, you’ll need to invoke your shaders within your rendering code. This typically involves setting the vertex and fragment shaders in the render encoder.
5.4. Advanced Shader Techniques
Shaders are incredibly versatile and can be used to create a wide range of visual effects. Some advanced shader techniques in Metal include:
- Texture Mapping: Applying textures to 3D models or surfaces for realistic rendering.
- Lighting Models: Implementing lighting models such as Phong or Blinn-Phong for realistic lighting effects.
- Post-processing Effects: Applying post-processing effects like bloom, motion blur, or depth of field.
- Particle Systems: Simulating and rendering particle systems for effects like fire, smoke, or sparks.
- Compute Shaders: Using shaders for general-purpose computing tasks, such as physics simulations or data processing.
6. Metal for Compute
While Metal is known for its graphics capabilities, it’s also a powerful framework for general-purpose GPU compute tasks. This makes it a valuable tool for a wide range of applications beyond graphics rendering.
6.1. GPU Compute Basics
In the context of Metal, GPU compute refers to using the GPU for non-graphics tasks. These tasks can include complex calculations, simulations, and data processing. By offloading these tasks to the GPU, you can leverage its parallel processing power for significant performance improvements.
6.2. Writing Compute Kernels
Compute tasks in Metal are implemented using compute kernels. A compute kernel is a function written in the Metal Shading Language (MSL) that is executed in parallel by the GPU.
Here’s a simple example of a compute kernel that adds two arrays element-wise:
metal kernel void add_arrays( device float* inputA [[buffer(0)]], device float* inputB [[buffer(1)]], device float* output [[buffer(2)]], uint id [[thread_position_in_grid]] ) { output[id] = inputA[id] + inputB[id]; }
In this example, the add_arrays kernel takes two input arrays and produces an output array by adding corresponding elements in parallel.
6.3. Dispatching Compute Work
To execute a compute kernel, you’ll need to dispatch it using a compute command encoder. The command encoder specifies the number of threads and threadgroups to use for the computation.
swift let commandBuffer = commandQueue.makeCommandBuffer() let computeEncoder = commandBuffer?.makeComputeCommandEncoder() computeEncoder?.setComputePipelineState(computePipelineState) computeEncoder?.setBuffer(inputBufferA, offset: 0, index: 0) computeEncoder?.setBuffer(inputBufferB, offset: 0, index: 1) computeEncoder?.setBuffer(outputBuffer, offset: 0, index: 2) let gridSize = MTLSize(width: count, height: 1, depth: 1) let threadgroupSize = MTLSize(width: 256, height: 1, depth: 1) computeEncoder?.dispatchThreads(gridSize, threadsPerThreadgroup: threadgroupSize) computeEncoder?.endEncoding() commandBuffer?.commit()
In this code, we’re dispatching the compute kernel with a specified grid size and threadgroup size to parallelize the computation.
6.4. Use Cases for GPU Compute
GPU compute with Metal can be applied to various use cases, including:
- Image and Video Processing: Enhancing and analyzing images and videos, such as applying filters or performing object recognition.
- Physics Simulations: Simulating physical phenomena like fluid dynamics, particle systems, or rigid body dynamics.
- Scientific Computing: Solving complex mathematical problems and simulations in fields like physics, chemistry, and biology.
- Machine Learning: Accelerating machine learning inference tasks using GPU compute.
- Data Parallelism: Performing parallel data processing tasks, such as sorting or searching.
7. Metal Beyond iOS
While Metal was initially designed for iOS, its significance has extended beyond Apple’s mobile devices. Apple introduced Metal for macOS, which allows developers to create high-performance graphics and compute applications on Macs. This means that the skills and knowledge you gain in Metal development for iOS can be applied to macOS development as well.
Additionally, Metal has found its way into other Apple products, such as Apple TV and watchOS, making it a versatile framework for a wide range of Apple platforms.
Conclusion
Metal is a powerful framework that unlocks the full potential of GPU acceleration on iOS and macOS devices. Whether you’re developing graphics-intensive games or harnessing the GPU for compute tasks, Metal provides the performance and flexibility you need.
In this introductory guide, we’ve covered the basics of Metal, from setting up your development environment to rendering graphics and performing GPU compute. As you continue your journey into Metal development, you’ll discover a world of possibilities for creating high-performance, visually stunning applications.
With its tight integration into the Apple ecosystem, modern API design, and support for advanced shader techniques, Metal is a key technology for developers looking to push the boundaries of what’s possible on Apple devices. So, dive in, experiment, and unlock the full potential of Metal in your iOS and macOS applications.
Table of Contents
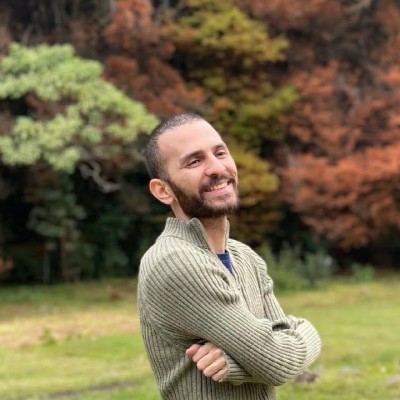
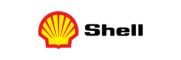