Working with Core NFC in iOS: Reading and Writing NFC Tags
Near Field Communication (NFC) technology has become an integral part of our daily lives. From contactless payments to sharing data with a simple tap, NFC is everywhere. In the world of iOS app development, Apple has provided the Core NFC framework to interact with NFC tags. This framework allows iOS apps to read and write NFC tags, opening up a world of possibilities for enhancing user experiences.
Table of Contents
In this comprehensive guide, we’ll dive deep into Core NFC, exploring how to read and write NFC tags within your iOS applications. Whether you’re a seasoned iOS developer or just getting started, we’ll provide step-by-step instructions, code samples, and practical use cases to help you harness the power of NFC in your apps.
1. Understanding Core NFC
Before we jump into the code, let’s get a clear understanding of what Core NFC is and what it can do for your iOS applications.
1.1. What is Core NFC?
Core NFC is a framework introduced by Apple that allows iOS devices to interact with NFC tags. It provides developers with the capability to read and write data on NFC tags using compatible iOS devices, such as iPhones.
1.2. Supported NFC Tag Types
Core NFC supports several NFC tag types, including:
- NFC Data Exchange Format (NDEF) Tags: These tags are commonly used for storing and sharing data, such as URLs, text, or contact information.
- ISO 7816 Tags: These tags are often used for smart cards and have specific application identifiers.
- MIFARE Tags: These are a type of contactless smart card often used in public transportation systems and access control.
- FeliCa Tags: Commonly used in Japan for various applications, including transit, e-money, and access control.
- ISO 15693 Tags: These tags are used for various purposes, including inventory management and library systems.
- Custom Tags: In addition to the above standard tag types, Core NFC also supports custom tag types, enabling you to work with proprietary NFC tags.
1.3. NFC Use Cases
Core NFC opens up a wide range of use cases for iOS applications, including:
- Mobile Payments: Implementing NFC-based payment solutions within your app.
- Access Control: Creating secure access control systems using NFC tags.
- Information Sharing: Enabling users to share information quickly by tapping their devices together.
- Inventory Management: Tracking and managing inventory using NFC tags.
- Authentication: Enhancing app security with NFC-based authentication.
Now that we have a good understanding of what Core NFC is and its potential use cases, let’s start working with it.
2. Reading NFC Tags
Reading NFC tags is a common scenario for many iOS applications. Whether you want to access information stored on an NFC tag or trigger specific actions based on tag data, Core NFC makes it straightforward.
2.1. Prerequisites
Before you start reading NFC tags in your iOS app, you need to ensure the following:
- NFC-Enabled Device: You must have an NFC-enabled iOS device. Core NFC works with iPhones 7 and newer.
- Xcode: Make sure you have Xcode installed on your development machine.
- Provisioning Profile: Create a provisioning profile for your app.
Step 1: Add Core NFC to Your Project
First, open your Xcode project or create a new one if you haven’t already. Then, follow these steps to add Core NFC to your project:
- In Xcode, select your project from the Project Navigator.
- Under the “General” tab, scroll down to the “Frameworks, Libraries, and Embedded Content” section.
- Click the “+” button and search for “Core NFC.”
- Select “Core NFC” from the search results and click “Add.”
Step 2: Request NFC Usage Permission
To read NFC tags, you need to request permission from the user. Open your app’s Info.plist file and add the “NFCReaderUsageDescription” key with a custom message describing why your app needs NFC access. This message will be shown to the user when requesting permission.
xml <key>NFCReaderUsageDescription</key> <string>We need NFC access to read and write NFC tags for inventory management.</string>
Step 3: Implement NFC Reading
Now, let’s dive into the code for reading NFC tags. Create a Swift file or add the following code to an existing one:
swift import UIKit import CoreNFC class NFCReaderViewController: UIViewController, NFCNDEFReaderSessionDelegate { var nfcSession: NFCNDEFReaderSession? override func viewDidLoad() { super.viewDidLoad() } @IBAction func startNFCReading(_ sender: UIButton) { nfcSession = NFCNDEFReaderSession(delegate: self, queue: nil, invalidateAfterFirstRead: false) nfcSession?.alertMessage = "Hold your iPhone near an NFC tag." nfcSession?.begin() } func readerSession(_ session: NFCNDEFReaderSession, didDetectNDEFs messages: [NFCNDEFMessage]) { // Handle detected NFC messages for message in messages { for record in message.records { // Process each record on the NFC tag let payload = String(data: record.payload, encoding: .utf8) print("NFC Tag Data: \(payload ?? "No data")") } } } func readerSession(_ session: NFCNDEFReaderSession, didInvalidateWithError error: Error) { // Handle errors if let nsError = error as NSError? { if nsError.code != 200 { print("NFC session invalidated with error: \(nsError.localizedDescription)") } } } }
In this code, we create an instance of NFCNDEFReaderSession and start the NFC reading session when the user taps a button. When an NFC tag is detected, the didDetectNDEFs method is called, allowing you to access the data on the tag. You can customize the handling of NFC tag data according to your app’s requirements.
Step 4: Start the NFC Reading Session
To start the NFC reading session, you need to present the NFCReaderViewController and call the startNFCReading method when the user taps a button. Here’s how you can present the view controller:
swift let nfcReaderVC = NFCReaderViewController() self.present(nfcReaderVC, animated: true, completion: nil)
That’s it! You’ve now implemented NFC reading in your iOS app. When the user holds their NFC-enabled device near an NFC tag, your app will be able to read the tag’s data and process it as needed.
3. Writing NFC Tags
Writing NFC tags with Core NFC is just as straightforward as reading them. You can write various types of data to NFC tags, such as URLs, text, or custom payloads.
3.1. Prerequisites
Before you start writing NFC tags in your iOS app, make sure you’ve completed the following prerequisites:
- NFC-Enabled Device: Ensure that you have an NFC-enabled iOS device for testing. NFC writing requires an iPhone XR or newer.
- Xcode: Have Xcode installed on your development machine.
- Provisioning Profile: Create a provisioning profile for your app.
Step 1: Add Core NFC to Your Project
If you’ve already added Core NFC to your project for reading NFC tags, you can skip this step. Otherwise, follow the same steps mentioned in the “Add Core NFC to Your Project” section under “Reading NFC Tags.”
Step 2: Request NFC Usage Permission
Similar to reading NFC tags, you need to request permission from the user to write NFC tags. Open your app’s Info.plist file and add the “NFCWriterUsageDescription” key with a custom message explaining why your app needs NFC write access.
xml <key>NFCWriterUsageDescription</key> <string>We need NFC access to write data to NFC tags for inventory management.</string>
Step 3: Implement NFC Writing
Now, let’s implement the code for writing data to NFC tags. Create a Swift file or add the following code to an existing one:
swift import UIKit import CoreNFC class NFCWriterViewController: UIViewController, NFCNDEFReaderSessionDelegate { var nfcSession: NFCNDEFReaderSession? override func viewDidLoad() { super.viewDidLoad() } @IBAction func startNFCWriting(_ sender: UIButton) { nfcSession = NFCNDEFReaderSession(delegate: self, queue: nil, invalidateAfterFirstRead: false) nfcSession?.alertMessage = "Hold your iPhone near an NFC tag to write data." nfcSession?.begin() } func readerSession(_ session: NFCNDEFReaderSession, didDetectNDEFs messages: [NFCNDEFMessage]) { // This method will not be used for writing NFC tags } func readerSession(_ session: NFCNDEFReaderSession, didInvalidateWithError error: Error) { // Handle errors if let nsError = error as NSError? { if nsError.code != 200 { print("NFC session invalidated with error: \(nsError.localizedDescription)") } } } }
In this code, we create an instance of NFCNDEFReaderSession as before, but this time, we are using it to write NFC tags. When the user taps a button to start writing, the didDetectNDEFs method will not be used, as it is specific to reading NFC tags. Instead, you will handle the writing process differently.
Step 4: Start the NFC Writing Session
To start the NFC writing session, you can present the NFCWriterViewController and call the startNFCWriting method when the user taps a button, similar to how we did it for reading NFC tags:
swift let nfcWriterVC = NFCWriterViewController() self.present(nfcWriterVC, animated: true, completion: nil)
Step 5: Write Data to NFC Tags
To write data to NFC tags, you’ll need to use the NFCNDEFTag class. Here’s an example of how you can write a URL to an NFC tag:
swift import CoreNFC class NFCWriterViewController: UIViewController, NFCNDEFReaderSessionDelegate { // ... Previous code ... func readerSession(_ session: NFCNDEFReaderSession, didBecomeActive tags: [NFCNDEFTag]) { guard let tag = tags.first else { session.invalidate(errorMessage: "No NFC tag detected.") return } let urlPayload = NFCNDEFPayload.wellKnownTypeURIPayload(string: "https://www.example.com") let urlRecord = NFCNDEFMessage(records: [urlPayload]) tag.writeNDEF(urlRecord) { error in if let error = error { print("Error writing to NFC tag: \(error.localizedDescription)") } else { print("Data written to NFC tag successfully.") } } session.invalidate() } // ... Remaining code ... }
In this code, we check if an NFC tag is detected, and if so, we create an NFCNDEFMessage with a URL payload and write it to the tag. You can customize the data you write to the tag according to your app’s requirements.
With these steps, you can enable your iOS app to write data to NFC tags. This capability can be incredibly useful for applications involving inventory management, marketing, or any scenario where you want to share data quickly via NFC.
4. Practical Use Cases
Now that you have learned how to read and write NFC tags using Core NFC in iOS, let’s explore some practical use cases where this technology can be applied.
4.1. Inventory Management
NFC tags can be used to track and manage inventory efficiently. Attach NFC tags to products or assets, and use your iOS app to read and update their status, location, and other relevant data.
4.2. Mobile Payments
Implement NFC-based mobile payment solutions within your app. Allow users to make contactless payments by tapping their NFC-enabled devices at supported payment terminals.
4.3. Access Control
Enhance security by using NFC tags for access control systems. Employees or users can gain access to specific areas by tapping their NFC-enabled devices on readers.
4.4. Information Sharing
Facilitate seamless information sharing among users. For example, in a networking app, users can exchange contact information by tapping their devices together.
4.5. Smart Posters
Create interactive marketing campaigns using NFC-enabled smart posters. Users can tap their devices on posters to access exclusive content or promotions.
4.6. Authentication
Implement two-factor authentication using NFC tags. Users can use their NFC-enabled devices as physical tokens to enhance app security.
Conclusion
Working with Core NFC in iOS allows you to tap into the exciting world of NFC technology. Whether you want to read data from NFC tags, write data to them, or explore various practical use cases, Core NFC provides a robust framework for your iOS app development needs.
In this guide, you’ve learned how to integrate Core NFC into your iOS projects, read and write NFC tags, and explored potential use cases. As you continue to develop your iOS applications, consider how NFC technology can enhance user experiences and open up new possibilities for your app’s functionality.
Unlock the potential of NFC in your iOS apps and stay at the forefront of mobile technology by incorporating Core NFC into your development toolkit. NFC-enabled devices are becoming increasingly common, making it an exciting time to leverage this technology in your projects. Happy coding!
Table of Contents
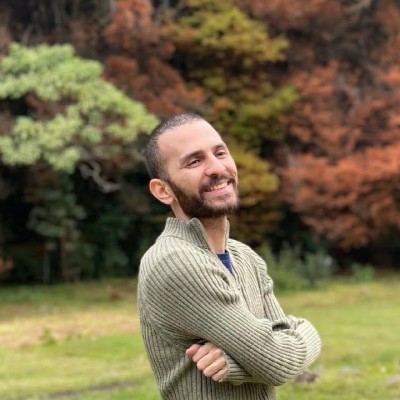
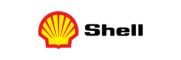