Introduction to Core Audio in iOS: Working with Audio Streams
Audio is an integral part of our digital experience, whether it’s music streaming, phone calls, or multimedia applications. When developing iOS applications that involve audio, you may find yourself needing more control and flexibility than what standard audio playback components can offer. This is where Core Audio, Apple’s powerful audio framework, comes into play. In this blog post, we’ll provide you with an introduction to Core Audio in iOS, focusing on working with audio streams.
Table of Contents
1. What is Core Audio?
Core Audio is a robust framework provided by Apple for working with audio on iOS and macOS platforms. It offers a wide range of features, from basic audio playback to advanced audio processing and synthesis. Core Audio enables developers to interact with audio at a lower level, giving them more control over audio data, processing, and rendering.
2. The Importance of Audio Streams
Audio streams are the lifeblood of any audio-related application. They represent a continuous flow of audio data that can come from various sources, including microphones, audio files, or network connections. Understanding how to work with audio streams is crucial for building applications like music players, voice recorders, real-time audio processing, and more.
In this blog post, we will explore the following key aspects of working with audio streams in iOS using Core Audio:
- Audio Data Representation: Understanding how audio data is represented in digital form.
- Audio Playback: Playing audio streams using Core Audio.
- Audio Recording: Capturing audio from the device’s microphone and saving it to a file.
- Audio Processing: Applying real-time audio effects and processing to audio streams.
3. Audio Data Representation
Before diving into working with audio streams, it’s essential to understand how audio data is represented digitally. In digital audio, sound waves are sampled at regular intervals to create a discrete representation of the continuous waveform. This discrete representation is typically stored as a series of numerical values in an audio buffer.
3.1. Sample Rate and Bit Depth
Two critical attributes of digital audio are the sample rate and bit depth:
- Sample Rate: The sample rate determines how many samples are taken per second. Common sample rates include 44.1 kHz (CD quality) and 48 kHz (common in digital audio).
- Bit Depth: Bit depth, also known as resolution, specifies the number of bits used to represent each sample. Common bit depths are 16-bit (CD quality) and 24-bit (higher quality).
Understanding these concepts is essential because they dictate the quality and accuracy of audio reproduction and processing.
3.2. Audio File Formats
Audio data can be stored in various file formats, each with its advantages and disadvantages. Common audio formats include WAV, MP3, AAC, and FLAC. When working with audio streams, you’ll often encounter these formats and need to understand how to read and write them.
4. Audio Playback
One of the most common use cases for Core Audio is audio playback. Let’s explore how you can play audio streams using Core Audio in iOS.
4.1. Setting Up an Audio Player
To get started with audio playback, you need to set up an audio player. Core Audio provides several ways to accomplish this, but one of the most accessible options is to use the AVAudioPlayer class, which is part of the AVFoundation framework.
Here’s a basic example of setting up an AVAudioPlayer:
swift import AVFoundation if let url = Bundle.main.url(forResource: "sample", withExtension: "mp3") { do { let audioPlayer = try AVAudioPlayer(contentsOf: url) audioPlayer.prepareToPlay() audioPlayer.play() } catch { print("Error loading audio file: \(error.localizedDescription)") } }
In this example, we create an AVAudioPlayer instance with the URL to an audio file (“sample.mp3”) and then call prepareToPlay() to prepare the player for playback. Finally, we call play() to start playing the audio.
4.2. Advanced Playback with Audio Units
While AVAudioPlayer is a convenient way to play audio, it may not offer the level of control required for more advanced audio applications. For fine-grained control over audio playback, you can use Audio Units, which are low-level audio processing units provided by Core Audio.
Here’s a simplified example of setting up audio playback using Audio Units:
swift import AudioToolbox var audioUnit: AudioUnit? var status = noErr // Create a description for the default output audio unit var ioUnitDescription = AudioComponentDescription( componentType: kAudioUnitType_Output, componentSubType: kAudioUnitSubType_RemoteIO, componentManufacturer: kAudioUnitManufacturer_Apple, componentFlags: 0, componentFlagsMask: 0 ) // Find and instantiate the default output audio unit let ioUnitComponent = AudioComponentFindNext(nil, &ioUnitDescription) status = AudioComponentInstanceNew(ioUnitComponent!, &audioUnit) if status == noErr { // Initialize the audio unit status = AudioUnitInitialize(audioUnit!) // Start audio playback status = AudioOutputUnitStart(audioUnit!) } if status != noErr { print("Error initializing audio unit: \(status)") }
In this example, we use Audio Units to set up audio playback. We create an audio unit description for the default output unit, find and instantiate it, initialize the audio unit, and start audio playback. This approach offers more control but requires a deeper understanding of Core Audio.
5. Audio Recording
In addition to audio playback, Core Audio also enables you to capture audio from various sources, such as the device’s microphone. Let’s explore how to record audio in iOS.
5.1. Setting Up an Audio Recorder
Similar to audio playback, you can use the AVFoundation framework to set up an audio recorder. Here’s an example of how to record audio:
swift import AVFoundation var audioRecorder: AVAudioRecorder! let audioSession = AVAudioSession.sharedInstance() do { try audioSession.setCategory(.playAndRecord, mode: .default) try audioSession.setActive(true) let audioSettings: [String: Any] = [ AVFormatIDKey: Int(kAudioFormatMPEG4AAC), AVSampleRateKey: 44100.0, AVNumberOfChannelsKey: 2, AVEncoderAudioQualityKey: AVAudioQuality.high.rawValue ] let documentsDirectory = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask)[0] let audioFileURL = documentsDirectory.appendingPathComponent("myRecording.m4a") audioRecorder = try AVAudioRecorder(url: audioFileURL, settings: audioSettings) audioRecorder.record() } catch { print("Error setting up audio recording: \(error.localizedDescription)") }
In this example, we set up an audio session, configure audio settings, specify the output file location, create an AVAudioRecorder instance, and start recording audio.
5.2. Advanced Recording with Audio Queue Services
If you need more control over audio recording, especially in scenarios where real-time processing or custom data handling is required, you can use Audio Queue Services provided by Core Audio.
Here’s a simplified example of setting up audio recording with Audio Queue Services:
swift import AudioToolbox var audioQueue: AudioQueueRef? var audioQueueBuffer: [AudioQueueBufferRef?] = [] // Audio stream format var audioFormat = AudioStreamBasicDescription( mSampleRate: 44100.0, mFormatID: kAudioFormatLinearPCM, mFormatFlags: kAudioFormatFlagIsSignedInteger | kAudioFormatFlagIsPacked, mBytesPerPacket: 2, mFramesPerPacket: 1, mBytesPerFrame: 2, mChannelsPerFrame: 1, mBitsPerChannel: 16, mReserved: 0 ) // Create an audio queue AudioQueueNewInput(&audioFormat, { (inUserData, inAQ, inBuffer, inStartTime, inNumPackets, inPacketDesc) in // Handle audio data here }, nil, nil, nil, 0, &audioQueue) // Allocate and enqueue audio buffers for _ in 0..<3 { var buffer: AudioQueueBufferRef? AudioQueueAllocateBuffer(audioQueue!, 44100, &buffer) AudioQueueEnqueueBuffer(audioQueue!, buffer!, 0, nil) } // Start audio recording AudioQueueStart(audioQueue!, nil)
In this example, we use Audio Queue Services to set up audio recording. We define the audio stream format, create an audio queue, allocate and enqueue audio buffers, and start audio recording. This approach provides more control over the recording process.
6. Audio Processing
Core Audio also allows you to apply real-time audio effects and processing to audio streams. This is especially useful for building applications like audio editors or live sound processing tools.
6.1. Audio Units for Processing
Audio Units, as mentioned earlier, are versatile components for audio processing. You can use them not only for playback but also for applying effects and processing to audio streams. Here’s a simplified example of adding an audio effect using an Audio Unit:
swift import AudioToolbox var audioUnit: AudioUnit? var status = noErr // Create a description for an audio effect unit (e.g., reverb) var effectDescription = AudioComponentDescription( componentType: kAudioUnitType_Effect, componentSubType: kAudioUnitSubType_Reverb2, componentManufacturer: kAudioUnitManufacturer_Apple, componentFlags: 0, componentFlagsMask: 0 ) // Find and instantiate the audio effect unit let effectComponent = AudioComponentFindNext(nil, &effectDescription) status = AudioComponentInstanceNew(effectComponent!, &audioUnit) if status == noErr { // Initialize the audio unit status = AudioUnitInitialize(audioUnit!) // Connect the audio unit to the audio graph // (not shown in this simplified example) } if status != noErr { print("Error initializing audio effect unit: \(status)") }
In this example, we create an audio effect unit description, find and instantiate the audio unit, initialize it, and connect it to the audio graph. You can then use this audio unit to apply real-time effects to audio streams.
Conclusion
Core Audio is a powerful framework that empowers iOS developers to work with audio streams at a low level, providing control and flexibility. In this blog post, we’ve covered the fundamental concepts of audio data representation, audio playback, audio recording, and audio processing using Core Audio in iOS. Armed with this knowledge, you can create audio-related applications with enhanced capabilities and fine-tuned audio experiences.
Whether you’re building a music player, a voice recorder, or a real-time audio processing app, Core Audio is your gateway to the world of audio on iOS. As you delve deeper into Core Audio, you’ll discover even more possibilities for creating immersive and innovative audio applications.
So, go ahead and explore the world of Core Audio, and let your creativity in audio development flourish. Your journey into the realm of iOS audio streams has just begun!
Table of Contents
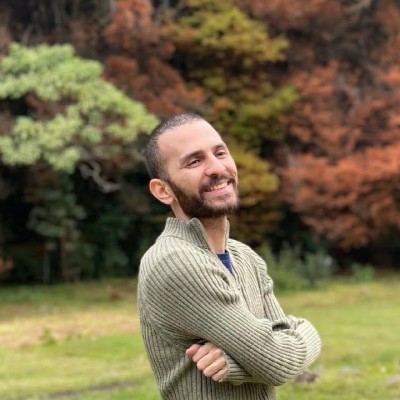
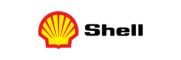