jQuery and Accessibility: Making Your Website Inclusive
In today’s digital landscape, creating an inclusive online experience is not just a consideration – it’s a responsibility. Web accessibility ensures that everyone, regardless of disabilities, can access and interact with your website. With the widespread use of JavaScript libraries like jQuery, developers have powerful tools at their disposal to enhance accessibility and improve user experiences. In this article, we’ll explore how jQuery can be utilized to make your website more inclusive and user-friendly.
Table of Contents
1. Understanding Accessibility
Before diving into the ways jQuery can enhance accessibility, let’s briefly understand what web accessibility entails. Accessibility refers to designing and developing websites and applications that can be used by people with disabilities, including visual, auditory, cognitive, or motor impairments. An accessible website ensures that all users can perceive, navigate, interact with, and contribute to your digital content.
Web Content Accessibility Guidelines (WCAG) outline principles and guidelines for creating accessible web content. These guidelines are categorized into four main principles: Perceivable, Operable, Understandable, and Robust (POUR). Implementing these principles ensures that your website can be used effectively by a diverse range of users.
2. The Role of jQuery in Accessibility
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversing, event handling, and animation. Its utility functions have made it a favorite among developers for enhancing user experiences on websites. While jQuery itself isn’t directly tied to accessibility, it can be employed to make your website more inclusive through various techniques.
2.1. Focus Management
One of the fundamental aspects of web accessibility is keyboard navigation. Users who rely on keyboard input, such as those with motor disabilities, need to navigate through your website seamlessly. jQuery can assist in managing focus by using the .focus() and .blur() methods. When users interact with elements like buttons or form fields, jQuery can help ensure that focus is applied and removed appropriately.
javascript // Adding focus to an element $('#button').on('click', function() { $('#inputField').focus(); }); // Removing focus from an element $('#inputField').on('blur', function() { // Perform validation or other actions });
2.2. Enhancing Semantic Structure
Semantic HTML is the foundation of web accessibility. Using appropriate HTML elements and attributes makes it easier for assistive technologies to interpret and convey content to users. jQuery can be employed to enhance the semantic structure of your webpage by dynamically modifying elements.
For example, if you’re building a collapsible content section, you can use jQuery to toggle ARIA attributes and roles, making the interactive content more understandable to screen readers.
html <div class="accordion"> <button class="accordion-button" aria-expanded="false" aria-controls="sectionContent"> Click to expand </button> <div id="sectionContent" class="accordion-content" aria-hidden="true"> <!-- Content goes here --> </div> </div> javascript $('.accordion-button').on('click', function() { $(this).attr('aria-expanded', function(_, attr) { return attr === 'true' ? 'false' : 'true'; }); $('#sectionContent').attr('aria-hidden', function(_, attr) { return attr === 'true' ? 'false' : 'true'; }); });
2.3. Handling Dynamic Content
Websites often include dynamic content that’s loaded or updated without requiring a full page refresh. When using jQuery to manage such content, it’s essential to consider accessibility implications. Ensure that newly loaded content is announced to screen readers, so users are aware of changes.
javascript // After loading new content dynamically $('#dynamicContent').html(newContent); // Notify screen readers about the content change $('#dynamicContent').attr('aria-live', 'polite');
2.4. Keyboard Accessibility
Creating a seamless keyboard navigation experience is crucial for users who rely on keyboard input. jQuery can help enhance keyboard accessibility by enabling keyboard shortcuts and focusing on interactive elements.
javascript // Triggering a function with a keyboard shortcut $(document).on('keydown', function(event) { if (event.key === 'Enter') { $('#submitButton').click(); } });
2.5. Customizing User Interactions
jQuery enables developers to create custom user interactions while maintaining accessibility. For instance, you can create a visually appealing and accessible tooltip using jQuery.
javascript $('.tooltip-trigger').on('mouseenter focus', function() { const tooltipText = $(this).data('tooltip'); $('<div class="tooltip"></div>') .text(tooltipText) .appendTo('body') .position({ my: 'left center', at: 'right center', of: this, collision: 'fit' }) .hide() .fadeIn(); }); $('.tooltip-trigger').on('mouseleave blur', function() { $('.tooltip').remove(); });
3. Best Practices for jQuery and Accessibility
While jQuery can greatly enhance website accessibility, it’s important to follow best practices to ensure that your efforts yield positive results:
3.1. Progressive Enhancement
Build your website with accessibility in mind from the beginning. Start with a solid HTML and CSS foundation, then enhance it with jQuery to provide dynamic interactions.
3.2. Test with Assistive Technologies
Regularly test your website with screen readers, keyboard navigation, and other assistive technologies to ensure that your jQuery enhancements are effective and usable.
3.3. Keep it Simple
Avoid overusing animations and interactions. Complexity can be confusing for users with cognitive disabilities or those using assistive technologies.
3.4. Provide Alternative Content
For dynamic content updates, provide alternative ways to access the information. For example, if you have a live content update, offer a “View Updates” link that takes users to the updated content.
3.5. Stay Updated
jQuery and accessibility best practices evolve over time. Stay updated with the latest guidelines and techniques to ensure your website remains inclusive.
Conclusion
Making your website accessible is not just a legal requirement; it’s an ethical responsibility. By harnessing the power of jQuery, you can create dynamic and engaging web experiences that cater to a wide range of users. Remember, small changes in your code can have a significant impact on someone’s browsing experience. By following accessibility best practices and utilizing jQuery’s capabilities, you can contribute to a more inclusive digital world. So, let’s code for a brighter and more accessible online future.
Table of Contents
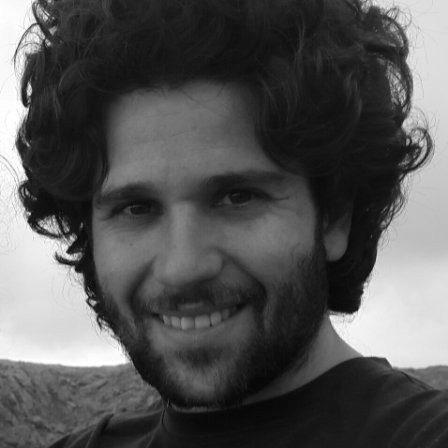
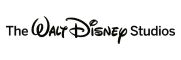