Advanced jQuery Techniques for Professional Web Development
In the ever-evolving world of web development, staying ahead of the curve is essential. jQuery, a fast, small, and feature-rich JavaScript library, has been a staple tool for front-end developers since its inception. While many developers are familiar with its basics, there are numerous advanced techniques that can take your web development skills to new heights. In this article, we’ll delve into some advanced jQuery techniques that professionals can leverage to create dynamic, interactive, and efficient websites.
Table of Contents
1. Dynamic Content Manipulation
1.1. Modifying HTML and Text
In professional web development, the ability to manipulate content on the fly is crucial. jQuery provides several methods to dynamically modify HTML and text. For instance, the .html() function allows you to change the inner HTML of an element. Take a look at this code snippet:
javascript $("#myElement").html("<p>New content here</p>");
1.2. Working with Attributes and Properties
Beyond altering content, you can manipulate attributes and properties of elements using jQuery. The .attr() function lets you get or set attributes, while the .prop() function does the same for properties. Here’s an example:
javascript $("#myImage").attr("src", "new_image.jpg"); $("#myCheckbox").prop("checked", true);
1.3. Creating Elements on the Fly
jQuery’s ability to create elements dynamically simplifies many complex tasks. The .append() and .prepend() functions allow you to insert content at the end or beginning of an element, respectively. The following code demonstrates adding a new paragraph to a div:
javascript $("#myDiv").append("<p>New paragraph</p>");
2. AJAX and Data Manipulation
2.1. Making Asynchronous Requests
Asynchronous JavaScript and XML (AJAX) is pivotal for seamless user experiences. jQuery’s AJAX functions, such as .ajax() and .get(), enable you to fetch data from servers without requiring a full page refresh. Below is an example using the .get() method:
javascript $.get("data.json", function(data) { // Process the data });
2.2. Handling Responses Effectively
Handling AJAX responses is equally important. jQuery simplifies this with its built-in functions. The .done(), .fail(), and .always() functions allow you to handle different response scenarios:
javascript $.get("data.json") .done(function(data) { // Handle successful response }) .fail(function(error) { // Handle error }) .always(function() { // Always execute, regardless of success or failure });
2.3. Manipulating Data with AJAX
With retrieved data, you can dynamically update your web page. For instance, if you’re dealing with JSON data, jQuery’s .each() function helps iterate through arrays:
javascript $.get("data.json", function(data) { $.each(data, function(index, item) { // Process each item }); });
3. Animations and Effects
3.1. Smooth Transitions with Animation
jQuery provides powerful animation functions to create smooth transitions. The .fadeIn(), .fadeOut(), .slideDown(), and .slideUp() functions add visual appeal to your website:
javascript $("#myElement").fadeIn(); $("#myElement").slideUp();
3.2. Custom Animations with animate()
For more intricate animations, the .animate() function reigns supreme. It lets you define custom animations with properties, duration, and easing:
javascript $("#myElement").animate({ opacity: 0.5, left: "+=50", height: "toggle" }, 1000, "linear");
3.3. Chaining Animations for Fluidity
jQuery’s method chaining capability enhances code readability and maintainability. You can chain animations together to create fluid user experiences:
javascript $("#myElement") .slideUp() .fadeIn() .animate({ opacity: 0.8 }, 500);
4. Event Handling Beyond Basics
4.1. Event Delegation for Efficiency
Event delegation is a crucial technique for improving performance, especially for dynamically generated content. Instead of binding events directly to elements, you bind them to a higher-level parent element:
javascript $("#parentElement").on("click", ".childElement", function() { // Event handler code });
4.2. Working with Custom Events
jQuery allows you to create and trigger custom events. This is useful for implementing complex interactions between components:
javascript $("#myButton").on("customEvent", function() { // Handle custom event }); $("#myButton").trigger("customEvent");
4.3. Unbinding and Namespacing Events
Unbinding events when they’re no longer needed prevents memory leaks. jQuery’s .off() function is handy for this. Additionally, you can namespace events to manage them efficiently:
javascript $("#myButton").on("click.myNamespace", function() { // Event handler code }); $("#myButton").off("click.myNamespace");
5. Performance Optimization
5.1. Caching for Efficiency
In jQuery, selecting elements can be resource-intensive. Caching selected elements in variables enhances performance:
javascript var $myElement = $("#myElement"); // Later in the code $myElement.hide();
5.2. Minimizing Repaints and Reflows
Frequent DOM updates can trigger repaints and reflows, impacting performance. Combining multiple updates into a single operation reduces this issue:
javascript $("#myElement").css({ "background-color": "blue", "color": "white", "font-size": "16px" });
5.3. Using Event Delegation for Performance
We’ve mentioned event delegation before, but it’s worth reiterating for its performance benefits. Instead of attaching events to numerous elements, delegate them to higher-level containers:
javascript $("#parentContainer").on("click", ".clickableItem", function() { // Event handling code });
Conclusion
As a professional web developer, mastering advanced jQuery techniques opens doors to creating more dynamic, interactive, and efficient websites. From manipulating content and data with AJAX to crafting animations and optimizing performance, jQuery remains a versatile tool in your arsenal. By implementing these advanced techniques, you’ll elevate your web development skills and create memorable user experiences that stand out in today’s competitive digital landscape. So dive into these techniques, experiment, and watch your development prowess reach new heights. Happy coding!
Table of Contents
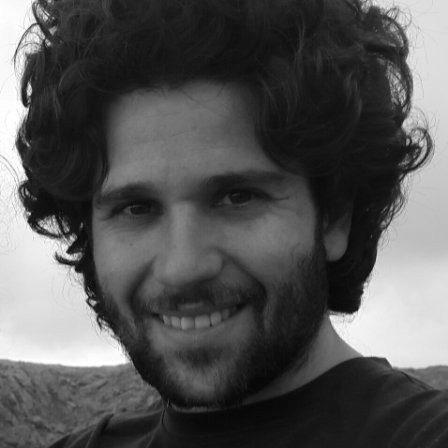
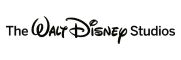