Simplifying AJAX Calls with jQuery AJAX Methods
In the realm of web development, asynchronous requests play a pivotal role in creating dynamic and interactive web applications. AJAX, which stands for Asynchronous JavaScript and XML, is a technique that allows you to send and receive data from a server without having to reload the entire web page. While AJAX is a powerful tool in a developer’s arsenal, it can sometimes be daunting, especially for beginners. This is where jQuery AJAX methods come to the rescue.
Table of Contents
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversal and manipulation, event handling, animation, and, most importantly, AJAX interactions. In this blog, we will dive deep into jQuery AJAX methods and explore how they can make your life as a developer easier.
1. Why jQuery AJAX Methods?
Before we jump into the technicalities, let’s understand why you might want to use jQuery AJAX methods in the first place.
1.1. Simplicity
jQuery abstracts away many of the complexities of raw JavaScript AJAX requests, making it easier to understand and implement. It provides a high-level interface that simplifies the process of making asynchronous requests.
1.2. Cross-Browser Compatibility
Cross-browser compatibility can be a headache when working with raw JavaScript AJAX. jQuery handles these browser-specific quirks for you, ensuring that your code works consistently across different browsers.
1.3. Powerful Callbacks
jQuery provides a robust set of callback functions that allow you to respond to different stages of the AJAX request, such as when the request is complete, successful, or encounters an error. This makes it easier to handle and process the server’s response.
Now that we have a clear understanding of why jQuery AJAX methods are beneficial, let’s dive into some of the most commonly used methods and their usage.
2. The Common jQuery AJAX Methods
jQuery provides several methods to perform AJAX requests, but four of the most commonly used ones are:
- $.ajax()
- $.get()
- $.post()
- $.getJSON()
Let’s explore each of these methods in detail.
2.1. $.ajax()
The $.ajax() method is the most versatile and customizable AJAX method provided by jQuery. It allows you to make HTTP requests with various options and handle the responses with callbacks.
Example:
javascript $.ajax({ url: 'https://api.example.com/data', method: 'GET', dataType: 'json', success: function(response) { // Handle a successful response here }, error: function(xhr, status, error) { // Handle errors here } });
In this example, we’re making a GET request to https://api.example.com/data, expecting a JSON response. If the request is successful, the success callback is invoked; otherwise, the error callback handles any errors that occur.
2.2. $.get()
The $.get() method is a shorthand for making GET requests. It simplifies the code for cases where you just need to fetch data from the server.
Example:
javascript $.get('https://api.example.com/data', function(response) { // Handle the response here });
This code achieves the same result as the previous example but with less verbosity. It automatically assumes a GET request and simplifies the syntax for handling the response.
2.3. $.post()
Similar to $.get(), the $.post() method is a shorthand for making POST requests. It simplifies sending data to the server.
Example:
javascript $.post('https://api.example.com/post-data', { key1: 'value1', key2: 'value2' }, function(response) { // Handle the response here });
In this example, we’re sending data to the server as part of the POST request. The server can then process this data and respond accordingly.
2.4. $.getJSON()
The $.getJSON() method is specifically designed for making GET requests and expecting JSON responses. It’s a more specialized shorthand compared to $.get().
Example:
javascript $.getJSON('https://api.example.com/json-data', function(response) { // Handle the JSON response here });
This method is handy when you know that you will be dealing with JSON data, as it automatically parses the JSON response for you.
3. Handling AJAX Responses
Now that you know how to make AJAX requests using jQuery, let’s dive into handling the responses.
3.1. Success Callback
The success callback function is called when the AJAX request is successful. You can use this callback to process the data returned by the server.
Example:
javascript $.get('https://api.example.com/data', function(response) { // Handle the successful response here });
3.2. Error Callback
The error callback function is called when an error occurs during the AJAX request. It can be used to handle errors gracefully and provide feedback to the user.
Example:
javascript $.ajax({ url: 'https://api.example.com/data', method: 'GET', dataType: 'json', success: function(response) { // Handle a successful response here }, error: function(xhr, status, error) { // Handle errors here } });
3.3. Complete Callback
The complete callback function is called regardless of whether the AJAX request is successful or encounters an error. It’s often used to perform cleanup tasks or to hide loading indicators.
Example:
javascript $.ajax({ url: 'https://api.example.com/data', method: 'GET', dataType: 'json', success: function(response) { // Handle a successful response here }, error: function(xhr, status, error) { // Handle errors here }, complete: function() { // This code runs after success or error } });
4. Adding AJAX Headers
Sometimes, you might need to include custom headers in your AJAX requests, such as authentication tokens or API keys. jQuery allows you to set headers easily using the headers option.
Example:
javascript $.ajax({ url: 'https://api.example.com/data', method: 'GET', dataType: 'json', headers: { 'Authorization': 'Bearer YOUR_TOKEN', 'Custom-Header': 'Custom-Value' }, success: function(response) { // Handle a successful response here }, error: function(xhr, status, error) { // Handle errors here } });
In this example, we’re setting two custom headers: Authorization and Custom-Header. You can adjust this according to your specific needs.
5. Handling AJAX with Promises
Promises are a modern way of handling asynchronous operations in JavaScript, and you can use them with jQuery AJAX methods. jQuery provides a way to return a promise from an AJAX call using the .then() method.
Example:
javascript $.get('https://api.example.com/data') .then(function(response) { // Handle the response here }) .catch(function(error) { // Handle errors here });
Using promises can make your code more organized and easier to read, especially when dealing with multiple asynchronous operations.
6. AJAX and Event Delegation
Event delegation is a powerful concept in JavaScript that allows you to attach a single event listener to a parent element and have it respond to events that occur on its child elements. This concept can be combined with AJAX to create dynamic web applications.
Example:
html <ul id="todo-list"> <li>Task 1</li> <li>Task 2</li> <li>Task 3</li> </ul> javascript $('#todo-list').on('click', 'li', function() { // This code runs when a <li> element is clicked var task = $(this).text(); // Make an AJAX request to mark the task as completed $.post('https://api.example.com/mark-complete', { task: task }, function(response) { // Handle the response here }); });
In this example, we’re using event delegation to listen for clicks on <li> elements within the #todo-list container. When an <li> element is clicked, an AJAX request is made to mark the corresponding task as complete.
7. AJAX and Cross-Origin Requests
Cross-origin requests, also known as CORS (Cross-Origin Resource Sharing), can be a challenge when working with AJAX. Browsers restrict AJAX requests to the same origin (domain, protocol, and port) by default for security reasons.
However, there are scenarios where you need to make AJAX requests to a different domain. To enable this, the server must include appropriate CORS headers in its response. If you don’t have control over the server, you can use JSONP (JSON with Padding) or server-side proxying as workarounds.
8. JSONP (JSON with Padding)
JSONP is a technique for overcoming the same-origin policy by dynamically adding script tags to the document. It allows you to fetch data from a different domain.
Example:
javascript $.ajax({ url: 'https://api.example.com/data?callback=?', dataType: 'jsonp', success: function(response) { // Handle the response here }, error: function(xhr, status, error) { // Handle errors here } });
In this example, we’re using the dataType: ‘jsonp’ option and appending ?callback=? to the URL. jQuery will automatically generate a unique callback function and handle the JSONP response.
9. Server-Side Proxying
Another way to overcome CORS restrictions is to use server-side proxying. You can create a server-side script on your domain that acts as a middleman between your web page and the remote server. Your web page sends requests to your server, and your server, in turn, fetches data from the remote server and sends it back to your web page.
This method is useful when you have control over the server and can configure it to allow your domain for AJAX requests.
10. Handling AJAX Loading Indicators
When making AJAX requests, it’s a good practice to provide visual feedback to the user, indicating that something is happening in the background. Loading indicators can improve the user experience and prevent confusion.
Here’s a simple example of how to show and hide a loading indicator during an AJAX request:
HTML:
html <div id="loading-indicator" style="display: none;">Loading...</div>
JavaScript:
javascript $('#loading-indicator').ajaxStart(function() { $(this).show(); }).ajaxStop(function() { $(this).hide(); });
In this example, we have a div element with the ID loading-indicator. We use the .ajaxStart() and .ajaxStop() methods to show and hide it automatically when AJAX requests start and stop.
11. Handling AJAX Forms
Forms are an integral part of web applications, and you can use jQuery AJAX methods to submit form data without refreshing the entire page. This technique is often referred to as AJAX form submission.
Example:
html <form id="my-form"> <input type="text" name="username" placeholder="Username"> <input type="password" name="password" placeholder="Password"> <button type="submit">Login</button> </form> <div id="response"></div> javascript $('#my-form').submit(function(e) { e.preventDefault(); // Prevent the form from submitting traditionally var formData = $(this).serialize(); // Serialize form data $.post('https://api.example.com/login', formData, function(response) { // Handle the login response $('#response').html(response); }); });
In this example, we prevent the form from submitting traditionally (which would cause a page reload) using e.preventDefault(). We then serialize the form data using $(this).serialize() and send it to the server via an AJAX POST request. The server responds with the result of the login attempt, which is displayed in the #response div.
Conclusion
jQuery AJAX methods provide a convenient and powerful way to work with asynchronous requests in web development. They simplify the process of making requests, handling responses, and dealing with common challenges like cross-origin requests and form submissions. By mastering these methods, you can enhance the interactivity and responsiveness of your web applications.
Remember that while jQuery is a valuable tool, it’s essential to stay up-to-date with modern web development practices. As the web evolves, new technologies and techniques may emerge that offer even more efficient ways to handle asynchronous operations. However, jQuery’s AJAX methods remain a reliable choice for simplifying AJAX calls in your web projects.
In summary, jQuery AJAX methods are a developer’s best friend when it comes to simplifying the complex world of asynchronous web requests. Whether you’re fetching data, handling forms, or dealing with cross-origin requests, jQuery’s intuitive API and powerful features make it a valuable addition to your web development toolkit. So, start simplifying your AJAX calls today with jQuery and watch your web applications come to life with dynamic content and interactivity. Happy coding!
Whether you’re a seasoned developer or just starting your journey in web development, jQuery AJAX methods can significantly simplify the process of making asynchronous requests. In this comprehensive guide, we’ve covered the most common jQuery AJAX methods, handling responses, dealing with cross-origin requests, and even enhancing user experience with loading indicators and AJAX forms. Armed with this knowledge, you’re well-equipped to create dynamic and interactive web applications with ease. Happy coding!
Table of Contents
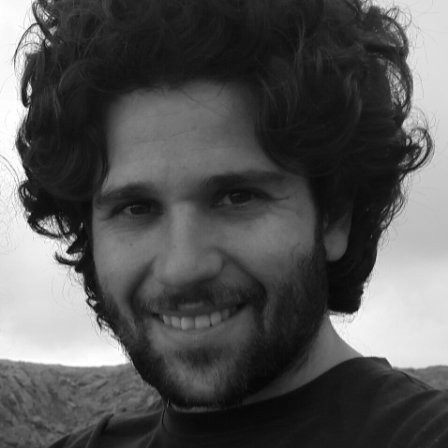
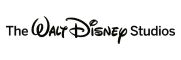