jQuery Animations: Adding Life to Your Web Pages
In the world of web development, aesthetics play a crucial role in captivating and engaging users. jQuery animations offer an excellent way to add life and interactivity to your web pages. With a simple and intuitive syntax, jQuery allows developers to create dynamic and visually appealing effects that enhance the user experience. In this blog, we will explore the world of jQuery animations, discussing various effects, transitions, and techniques to create compelling web pages.
1. Understanding jQuery Animations:
1.1 What is jQuery?
jQuery is a popular JavaScript library that simplifies HTML document traversal, event handling, and animations. It provides a wide range of functions and methods that make it easier to manipulate web page elements and create interactive user experiences.
1.2 Why Use jQuery Animations?
jQuery animations offer numerous benefits for web developers:
- Enhance user experience: Animations can grab users’ attention, guide their focus, and provide visual feedback.
- Cross-browser compatibility: jQuery takes care of browser inconsistencies, ensuring animations work across various platforms.
- Simplified syntax: jQuery’s syntax is concise and easy to understand, making it accessible to developers of all skill levels.
- Time-saving: jQuery simplifies complex animation tasks, saving developers valuable time and effort.
2. Getting Started with jQuery Animations:
2.1 Setting Up jQuery:
To get started with jQuery animations, you need to include the jQuery library in your HTML file. You can either download the library and host it locally or use a content delivery network (CDN) to link to the library hosted on a remote server. Here’s an example:
html <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> </head>
2.2 Basic Animation Effects:
jQuery provides a range of built-in animation effects, including fading, sliding, and toggling. Let’s explore a few examples:
javascript // Fading an element in and out $("#elementId").fadeIn(); $("#elementId").fadeOut(); // Sliding an element up and down $("#elementId").slideUp(); $("#elementId").slideDown(); // Toggling an element's visibility $("#elementId").toggle();
3. Advanced Animation Techniques:
3.1 Chaining Animations:
One of the strengths of jQuery is the ability to chain animations, creating complex and dynamic effects. By using the animate() method, you can create a sequence of animations that occur one after another:
javascript $("#elementId") .animate({ opacity: 0.5 }) .animate({ left: "250px" }) .animate({ fontSize: "30px" });
3.2 Animating CSS Properties:
jQuery allows you to animate CSS properties of elements. You can animate properties such as width, height, color, and more:
javascript $("#elementId").animate({ width: "200px", height: "200px", backgroundColor: "red" });
3.3 Working with Easing Functions:
Easing functions provide control over the speed of animations, allowing for smooth transitions. jQuery provides various easing functions, such as “swing” and “linear,” or you can use custom easing functions. Here’s an example using the “easeInOutExpo” easing function from the jQuery Easing Plugin:
javascript $("#elementId").animate({ left: "250px", top: "250px" }, 1000, "easeInOutExpo");
4. Creating Transitions and Transformations:
4.1 Fading In and Out:
Fade effects are commonly used to smoothly transition elements in and out of visibility. Here’s an example of fading an element in and out on button click:
javascript $("#fadeButton").click(function() { $("#elementId").fadeToggle(); });
4.2 Sliding Effects:
Slide effects can be used to reveal or hide elements by sliding them in various directions. Here’s an example of sliding an element horizontally:
javascript $("#slideButton").click(function() { $("#elementId").slideToggle("slow"); });
4.3 Rotating and Scaling Elements:
With jQuery animations, you can create engaging transformations like rotating or scaling elements. Here’s an example of rotating an element on button click:
javascript $("#rotateButton").click(function() { $("#elementId").animate({ transform: "rotate(180deg)" }); });
5. Interactivity and Event Handling:
5.1 Triggering Animations with Events:
jQuery allows you to trigger animations based on user interactions or specific events. Here’s an example of animating an element on button hover:
javascript $("#hoverButton").hover( function() { $("#elementId").animate({ fontSize: "30px" }); }, function() { $("#elementId").animate({ fontSize: "16px" }); } );
5.2 Creating Interactive Menus:
You can use jQuery animations to create interactive menus that expand or collapse on click. Here’s an example of a collapsible menu:
javascript $(".menu-item").click(function() { $(this).next(".sub-menu").slideToggle(); });
6. Enhancing User Experience with Animation Libraries:
6.1 Animate.css:
Animate.css is a popular animation library that works seamlessly with jQuery. It provides a wide range of pre-built animations that can be easily applied to elements. To use Animate.css, include the library in your HTML file and add the desired animation class to your element:
html <head> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/animate.css/4.1.1/animate.min.css"> </head> <div class="animate__animated animate__bounce">Bouncing Element</div>
6.2 Velocity.js:
Velocity.js is another powerful animation library that offers advanced animation capabilities. It provides high-performance animations with a simple syntax. To use Velocity.js, include the library in your HTML file and start animating:
html <head> <script src="https://cdnjs.cloudflare.com/ajax/libs/velocity/2.0.6/velocity.min.js"></script> </head> <script> $("#elementId").velocity({ width: "200px" }, 1000); </script>
7. Best Practices for Using jQuery Animations:
7.1 Optimizing Performance:
Minimize the number of animations on a single page to prevent performance issues.
Use hardware-accelerated properties (e.g., opacity, transform) for smoother animations.
Utilize requestAnimationFrame for better performance and smoother frame rates.
7.2 Ensuring Compatibility:
Test your animations on multiple browsers and devices to ensure consistent behavior.
Use feature detection techniques to provide fallbacks for browsers that don’t support certain animations.
Optimize animations for mobile devices by considering performance constraints.
Conclusion:
jQuery animations are a powerful tool for creating engaging and interactive web pages. By leveraging the built-in animation effects, advanced techniques, and external animation libraries, you can bring your web pages to life. Whether you’re adding subtle transitions or creating complex transformations, jQuery provides a user-friendly and efficient way to enhance the visual appeal and user experience of your web projects. So go ahead, experiment with animations, and take your web development skills to new heights!
Table of Contents
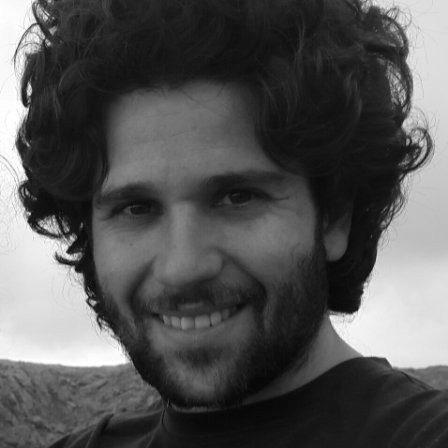
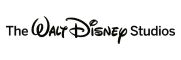