Building Animated Countdowns with jQuery Countdown
Countdowns are powerful tools for building anticipation, whether you’re launching a new product, promoting an event, or counting down to a special occasion. While there are various ways to implement countdowns, jQuery Countdown stands out as a versatile and user-friendly solution. In this comprehensive guide, we’ll explore how to build animated countdowns using jQuery Countdown, step by step.
Table of Contents
1. Why Choose jQuery Countdown?
Before we dive into the implementation, it’s essential to understand why jQuery Countdown is an excellent choice for creating animated countdowns.
1.1. Ease of Use
jQuery Countdown is known for its simplicity. You don’t need to be a JavaScript guru to use it effectively. With just a basic understanding of HTML and JavaScript, you can create stunning countdowns.
1.2. Customization Options
jQuery Countdown offers a wide range of customization options. You can tailor your countdown to match the style and theme of your website or application, ensuring it seamlessly integrates with your design.
1.3. Animations
The real magic of jQuery Countdown lies in its ability to create animated countdowns. These animations not only grab the user’s attention but also add an element of excitement and engagement to your website.
1.4. Compatibility
jQuery Countdown is highly compatible with various browsers, ensuring a consistent experience for all your users, regardless of the platform they’re using.
Now that we’ve covered the advantages let’s get into the nitty-gritty of building animated countdowns with jQuery Countdown.
2. Getting Started
2.1. Setting Up the Environment
Before you can create your animated countdown, you need to set up your development environment. Ensure you have the following prerequisites:
- HTML file
- jQuery library
- jQuery Countdown plugin
You can include the jQuery library and Countdown plugin by adding the following code to your HTML file’s <head> section:
html <!-- jQuery library --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- jQuery Countdown plugin --> <script src="https://cdn.rawgit.com/hilios/jQuery.countdown/2.2.0/dist/jquery.countdown.min.js"></script>
2.2. Creating the Countdown HTML Structure
Now, let’s set up the HTML structure for our countdown. Create a <div> element with a unique ID to hold your countdown. For example:
html <div id="countdown"></div>
This is where your countdown will be displayed.
2.3. Initializing jQuery Countdown
Next, you’ll need to initialize jQuery Countdown. Add the following JavaScript code to your HTML file, usually just before the closing </body> tag:
html <script> $(document).ready(function() { // Set the target date for your countdown var targetDate = new Date("2023-12-31 23:59:59"); // Initialize the countdown $('#countdown').countdown(targetDate, function(event) { // Update the countdown's display $(this).html(event.strftime('%D days %H:%M:%S')); }); }); </script>
In this code, we first set the target date for our countdown. You can replace “2023-12-31 23:59:59” with your desired end date and time. The countdown function initializes the countdown, and the event.strftime method formats the countdown display.
3. Customizing Your Countdown
One of the standout features of jQuery Countdown is its flexibility in customization. Let’s explore some ways to tailor your countdown to your specific needs.
3.1. Changing the Display Format
The event.strftime method allows you to control the display format of your countdown. Here are some common placeholders you can use:
- %D: Days
- %H: Hours
- %M: Minutes
- %S: Seconds
You can arrange these placeholders to create your desired format. For example, if you want to display the countdown in the format “10 days 05:30:15,” you can use the following code:
javascript $(this).html(event.strftime('%D days %H:%M:%S'));
Feel free to experiment with different formats to find the one that best suits your website’s style.
3.2. Adding Labels
To make your countdown more user-friendly, you can add labels to the display. For instance, you can display “Days” next to the number of days remaining. Here’s how you can do it:
javascript $(this).html(event.strftime('%D <span class="label">days</span> %H:%M:%S'));
In this example, we’ve wrapped the “days” label in a <span> element with the class “label.” You can style it using CSS to match your design.
3.3. Custom Styling
Speaking of styling, you can further customize the appearance of your countdown with CSS. For example, you can change the font, color, and size to match your website’s theme. Here’s a sample CSS snippet to get you started:
css #countdown { font-family: Arial, sans-serif; font-size: 24px; color: #333; }
This CSS code sets the font to Arial, adjusts the font size to 24 pixels, and changes the text color to a dark gray (#333). Adjust these styles according to your design preferences.
4. Adding Animations
Now comes the fun part—adding animations to your countdown. Animations not only make your countdown visually appealing but also capture the user’s attention. jQuery Countdown allows you to animate the countdown easily.
4.1. Fade In Animation
To create a simple fade-in animation when the countdown starts, you can use jQuery’s fadeIn method. First, make sure your countdown is hidden by default using CSS:
css #countdown { display: none; }
Next, modify your JavaScript code as follows:
javascript $(document).ready(function() { // Set the target date for your countdown var targetDate = new Date("2023-12-31 23:59:59"); // Initialize the countdown $('#countdown').countdown(targetDate, function(event) { // Update the countdown's display $(this).html(event.strftime('%D days %H:%M:%S')); // Add fade-in animation $(this).fadeIn(); }); });
Now, when the countdown starts, it will smoothly fade in, creating a visually pleasing effect.
4.2. Countdown Flip Animation
For a more advanced animation, you can implement a countdown flip effect, where the numbers flip like an old-school scoreboard. This effect adds a touch of nostalgia and uniqueness to your countdown.
First, you’ll need to include the FlipClock.js library, which works well with jQuery Countdown. Add the following code to your <head> section, below the jQuery Countdown script:
html <!-- FlipClock.js library --> <link rel="stylesheet" href="https://cdn.rawgit.com/objectivehtml/FlipClock/master/compiled/flipclock.css"> <script src="https://cdn.rawgit.com/objectivehtml/FlipClock/master/compiled/flipclock.min.js"></script>
Now, you can use FlipClock.js to create the flip animation. Replace your existing JavaScript code with the following:
javascript $(document).ready(function() { // Set the target date for your countdown var targetDate = new Date("2023-12-31 23:59:59"); // Initialize the FlipClock countdown $('#countdown').FlipClock({ clockFace: 'DailyCounter', countdown: true, showSeconds: true, autoStart: true, callbacks: { stop: function() { // Handle countdown completion here } } }); });
This code initializes the FlipClock countdown and enables the daily counter with seconds. You can further customize the appearance and behavior of the FlipClock countdown by adjusting the options.
5. Countdown Completion
When your countdown reaches zero, it’s essential to have a plan for what happens next. You might want to display a message, redirect users to a different page, or trigger a specific action. jQuery Countdown provides a callback function that you can use for this purpose.
In the previous example with the FlipClock countdown, we included a stop callback. This callback is called when the countdown reaches zero. You can customize it to suit your needs. For example, to display a message when the countdown ends, you can modify the code like this:
javascript $('#countdown').FlipClock({ // ...other options... callbacks: { stop: function() { // Display a message when the countdown ends $(this.el).html("Countdown has ended!"); } } });
Feel free to adapt this code to perform actions specific to your website or application when the countdown reaches its end.
6. Advanced Tips
To take your animated countdowns to the next level, consider these advanced tips:
6.1. Multiple Countdowns on One Page
You can have multiple countdowns on a single page by creating separate <div> elements with unique IDs and initializing jQuery Countdown for each one individually.
html <div id="countdown1"></div> <div id="countdown2"></div> javascript Copy code $(document).ready(function() { // Set target dates for each countdown var targetDate1 = new Date("2023-12-31 23:59:59"); var targetDate2 = new Date("2024-01-31 23:59:59"); // Initialize the first countdown $('#countdown1').countdown(targetDate1, function(event) { $(this).html(event.strftime('%D days %H:%M:%S')); }); // Initialize the second countdown $('#countdown2').countdown(targetDate2, function(event) { $(this).html(event.strftime('%D days %H:%M:%S')); }); });
6.2. Responsive Design
Ensure that your countdown is responsive to different screen sizes. Use CSS media queries to adjust the countdown’s styling for mobile devices and tablets. This ensures that your countdown remains visually appealing and functional on all platforms.
6.3. Localization
jQuery Countdown supports localization, allowing you to display countdowns in different languages. You can specify language options when initializing the countdown. For example, to display the countdown in French, use the following code:
javascript $('#countdown').countdown(targetDate, { language: 'fr' });
Conclusion
Creating animated countdowns with jQuery Countdown is a straightforward yet effective way to engage your website’s visitors, build excitement, and promote events or product launches. With its ease of use, customization options, and compatibility, jQuery Countdown provides a reliable solution for developers.
Remember that the key to a successful countdown lies not only in its functionality but also in its ability to captivate your audience. By adding animations and customizing the countdown to match your website’s style, you can create a memorable and enticing user experience.
So, go ahead and start building your animated countdowns with jQuery Countdown. Experiment with different styles, animations, and features to create countdowns that leave a lasting impression on your users. Whether you’re counting down to a product release, a big event, or the start of a new year, jQuery Countdown has you covered.
Table of Contents
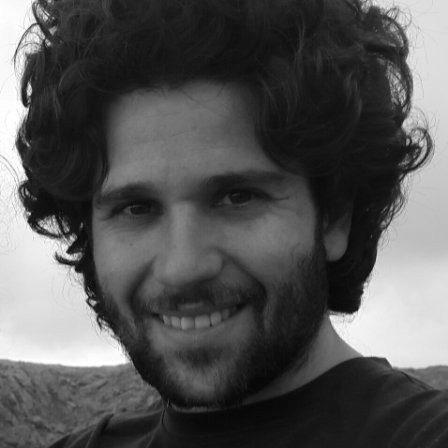
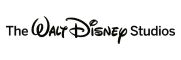