Building Dynamic Content Loaders with jQuery AJAX
In today’s fast-paced digital world, user experience is paramount. Slow-loading websites can frustrate users and lead to high bounce rates. To tackle this challenge, developers often turn to techniques that enhance the loading speed and interactivity of their websites. One such technique is using jQuery AJAX to build dynamic content loaders. In this blog post, we’ll dive into the world of dynamic content loaders, exploring their benefits, implementation, and code samples.
Table of Contents
1. Understanding Dynamic Content Loading
1.1. What is Dynamic Content Loading?
Dynamic content loading is a technique used to fetch and display content on a web page without requiring a full page refresh. It allows developers to load data, such as text, images, or even entire sections of a website, dynamically, enhancing the user experience by providing seamless and interactive content retrieval.
1.2. Benefits of Dynamic Content Loading
Dynamic content loading offers several benefits, including:
- Faster Load Times: By fetching only the necessary content, dynamic loading reduces initial page load times, ensuring a smoother experience for users.
- Reduced Bandwidth Usage: Loading only the required data minimizes bandwidth consumption, making the website more efficient, especially for users on slower connections or mobile devices.
- Improved User Engagement: With dynamic loaders, users can interact with the site while content loads in the background, keeping them engaged rather than frustrated by long loading times.
- Enhanced Aesthetics: Content can be loaded in a visually appealing manner, such as fading in or sliding into view, adding a touch of elegance to the user interface.
2. Introducing jQuery AJAX
2.1. What is jQuery AJAX?
jQuery AJAX (Asynchronous JavaScript and XML) is a powerful technique that allows you to send and retrieve data from a server asynchronously without needing to refresh the entire page. It leverages JavaScript’s capabilities to interact with the server, making it possible to fetch data and update parts of a web page in real-time.
2.2. Why Choose jQuery AJAX for Dynamic Loading?
jQuery AJAX simplifies the process of implementing dynamic content loading by providing an easy-to-use interface for making asynchronous requests. Its cross-browser compatibility and straightforward syntax make it a popular choice among developers.
By using jQuery AJAX for dynamic loading, you can focus on enhancing user experience without getting bogged down by complex server-side interactions.
3. Building Dynamic Content Loaders Step by Step
Let’s walk through the process of building a dynamic content loader using jQuery AJAX.
3.1. Setting Up HTML Structure
Start by creating the HTML structure for the content loader. For this example, let’s assume you want to load and display a list of blog posts:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Dynamic Content Loader</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="scripts.js"></script> </head> <body> <div id="content"> <!-- Content will be dynamically loaded here --> </div> </body> </html>
In this example, we’ve included the jQuery library and a separate JavaScript file named “scripts.js.”
3.2. Writing jQuery AJAX Code
In the “scripts.js” file, write the jQuery AJAX code to fetch and display the content. We’ll use a mock API endpoint for demonstration purposes:
javascript $(document).ready(function() { $.ajax({ url: 'https://api.example.com/posts', // Replace with your API endpoint method: 'GET', dataType: 'json', success: function(data) { // Process and display the data var contentDiv = $('#content'); data.forEach(function(post) { var postElement = '<div class="post">' + '<h2>' + post.title + '</h2>' + '<p>' + post.body + '</p>' + '</div>'; contentDiv.append(postElement); }); } }); });
In this code, we use the $.ajax() method to send a GET request to the API endpoint. Once the data is retrieved successfully, we iterate through the list of blog posts and append them to the “content” div.
3.3. Displaying Loading Animation
To enhance the user experience, it’s a good practice to display a loading animation while the content is being fetched. You can do this by modifying the jQuery AJAX code:
javascript $(document).ready(function() { var contentDiv = $('#content'); contentDiv.html('<div class="loading">Loading...</div>'); // Display loading animation $.ajax({ url: 'https://api.example.com/posts', // Replace with your API endpoint method: 'GET', dataType: 'json', success: function(data) { contentDiv.empty(); // Remove loading animation data.forEach(function(post) { var postElement = '<div class="post">' + '<h2>' + post.title + '</h2>' + '<p>' + post.body + '</p>' + '</div>'; contentDiv.append(postElement); }); } }); });
Here, we’ve added a loading animation div before making the AJAX request and removed it once the data is loaded successfully.
3.4. Handling AJAX Errors
It’s essential to handle errors gracefully to ensure a smooth user experience. You can add an error handling function to your jQuery AJAX code:
javascript $(document).ready(function() { var contentDiv = $('#content'); contentDiv.html('<div class="loading">Loading...</div>'); $.ajax({ url: 'https://api.example.com/posts', // Replace with your API endpoint method: 'GET', dataType: 'json', success: function(data) { contentDiv.empty(); data.forEach(function(post) { var postElement = '<div class="post">' + '<h2>' + post.title + '</h2>' + '<p>' + post.body + '</p>' + '</div>'; contentDiv.append(postElement); }); }, error: function() { contentDiv.html('<div class="error">Failed to load content.</div>'); } }); });
The error callback is triggered if the AJAX request encounters an issue. In this case, we display an error message to the user.
4. Advanced Techniques for Enhanced User Experience
While the basic dynamic content loader is effective, there are advanced techniques you can implement for an even better user experience.
4.1. Implementing Infinite Scroll
Infinite scroll allows users to continuously load content as they scroll down the page. This technique eliminates the need for pagination and provides a seamless browsing experience. You can achieve this by monitoring the user’s scroll position and triggering additional AJAX requests to fetch more content.
javascript var isLoading = false; var page = 1; $(window).on('scroll', function() { if ($(window).scrollTop() + $(window).height() >= $(document).height() - 100 && !isLoading) { isLoading = true; page++; loadMoreContent(page); } }); function loadMoreContent(page) { // Update the API endpoint with the appropriate page parameter var endpoint = 'https://api.example.com/posts?page=' + page; $.ajax({ url: endpoint, method: 'GET', dataType: 'json', success: function(data) { isLoading = false; data.forEach(function(post) { // Append the new posts to the contentDiv }); }, error: function() { isLoading = false; } }); }
4.2. Preloading Content
Preloading content anticipates user actions and fetches content before it’s requested. This can be particularly useful for image-heavy websites. You can preload content by initiating AJAX requests in advance and storing the data for later use.
javascript var preloadedData = []; $(document).ready(function() { $.ajax({ url: 'https://api.example.com/preload', // Replace with preloading API endpoint method: 'GET', dataType: 'json', success: function(data) { preloadedData = data; } }); }); // When user triggers content display function displayPreloadedContent() { var contentDiv = $('#content'); preloadedData.forEach(function(post) { // Display the preloaded content }); }
4.3. Caching Retrieved Data
Caching involves storing previously fetched data to reduce the need for repeated AJAX requests. This can significantly enhance loading speeds and minimize server load. jQuery AJAX itself doesn’t provide built-in caching, but you can achieve caching using browser storage mechanisms like Local Storage or Session Storage.
javascript $(document).ready(function() { var cachedData = JSON.parse(localStorage.getItem('cachedPosts')); if (cachedData) { // Use cached data to display content } else { $.ajax({ url: 'https://api.example.com/posts', // Replace with your API endpoint method: 'GET', dataType: 'json', success: function(data) { localStorage.setItem('cachedPosts', JSON.stringify(data)); // Display content using data } }); } });
By implementing these advanced techniques, you can provide users with a more fluid and engaging experience while optimizing data retrieval and resource usage.
Conclusion
Dynamic content loaders powered by jQuery AJAX are valuable tools for improving user experience by delivering content seamlessly and efficiently. They contribute to faster load times, reduced bandwidth consumption, and enhanced engagement. By following the step-by-step guide in this article, you can easily integrate dynamic content loaders into your web projects and leverage advanced techniques to further elevate the user experience. Whether you’re building a blog, an e-commerce site, or any other type of web application, dynamic content loaders can help you create a more user-friendly and responsive interface. So why wait? Start implementing dynamic content loaders with jQuery AJAX and provide your users with a browsing experience they’ll appreciate.
Table of Contents
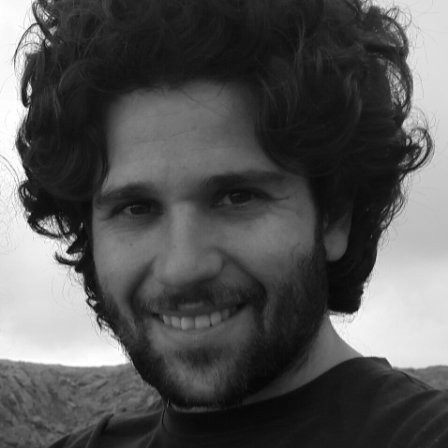
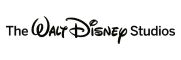