Building Interactive Dashboards with jQuery and Chart.js
In the ever-evolving world of web development, data visualization is a crucial element. Interactive dashboards offer a powerful way to present data, enabling users to explore and analyze information intuitively. In this guide, we’ll explore how to build interactive dashboards using two powerful tools: jQuery and Chart.js.
Table of Contents
1. Why jQuery and Chart.js?
Before we dive into the technical details, let’s briefly understand why we’ve chosen jQuery and Chart.js for this task.
1.1. jQuery: A Versatile JavaScript Library
jQuery has been a staple in web development for years due to its simplicity and extensive community support. It simplifies DOM manipulation, event handling, and AJAX requests, making it an excellent choice for building interactive web applications. In our case, jQuery will help us create dynamic and responsive dashboards with ease.
1.2. Chart.js: Elegant Data Visualization
Chart.js is a JavaScript library that specializes in creating visually stunning charts and graphs. It provides a wide range of chart types, from bar charts and line charts to pie charts and radar charts. Chart.js is user-friendly and highly customizable, making it perfect for building interactive dashboards that display data in a meaningful way.
Now that we understand our tools let’s dive into the step-by-step process of building an interactive dashboard.
2. Setting Up Your Development Environment
Before we start coding, ensure that you have a development environment set up. You’ll need the following:
- Code Editor: Use a code editor like Visual Studio Code, Sublime Text, or any other of your choice.
- Web Browser: We’ll be testing our dashboard in a web browser, so make sure you have one installed (e.g., Chrome, Firefox, or Edge).
- Basic Knowledge: Familiarity with HTML, CSS, and JavaScript will be helpful.
Now, let’s get started!
3. Creating the HTML Structure
The foundation of any web page is its HTML structure. In our case, we want to create an organized structure for our dashboard. Here’s a simple HTML template to start with:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Interactive Dashboard</title> <!-- Include jQuery --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- Include Chart.js --> <script src="https://cdn.jsdelivr.net/npm/chart.js"></script> <!-- Include your custom CSS file --> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="dashboard"> <!-- Your dashboard content goes here --> </div> <!-- Include your custom JavaScript file --> <script src="script.js"></script> </body> </html>
In this template, we’ve included the necessary jQuery and Chart.js libraries, as well as placeholders for your custom CSS and JavaScript files.
4. Designing the Dashboard Layout
Now that we have our HTML structure in place let’s work on designing the dashboard layout. CSS will help us achieve the desired look and feel. Here’s an example of a basic dashboard layout:
css /* styles.css */ .dashboard { display: grid; grid-template-columns: 1fr 1fr; /* Create a two-column layout */ grid-gap: 20px; /* Add some spacing between elements */ } .chart-container { border: 1px solid #ccc; padding: 20px; background-color: #fff; border-radius: 5px; }
In this CSS snippet, we’ve defined a simple two-column grid layout for our dashboard. Each chart will reside in a .chart-container div, which provides some styling to make the dashboard visually appealing.
5. Initializing Chart.js
Now that we have our HTML structure and CSS in place, it’s time to start using Chart.js to create interactive charts. Let’s create a simple bar chart as an example.
First, add a canvas element to your HTML where you want the chart to appear:
html <div class="chart-container"> <canvas id="bar-chart"></canvas> </div>
Next, initialize Chart.js in your JavaScript file:
javascript // script.js $(document).ready(function () { // Get the canvas element var ctx = document.getElementById('bar-chart').getContext('2d'); // Create the data for your chart var data = { labels: ['January', 'February', 'March', 'April', 'May'], datasets: [{ label: 'Monthly Sales', data: [65, 59, 80, 81, 56], backgroundColor: 'rgba(75, 192, 192, 0.2)', // Bar color borderColor: 'rgba(75, 192, 192, 1)', // Border color borderWidth: 1, // Border width }] }; // Create the chart var myChart = new Chart(ctx, { type: 'bar', data: data, }); });
In this code, we first get the canvas element using getElementById. Then, we define our chart’s data, including labels, data points, and styling options. Finally, we create a new Chart instance, specifying the chart type (in this case, ‘bar’) and the data to use.
6. Adding Interactivity with jQuery
To make our dashboard truly interactive, we can use jQuery to add dynamic features. For example, let’s say we want to update the chart’s data when the user clicks a button. First, add a button to your HTML:
html <button id="update-chart">Update Chart</button>
Now, use jQuery to handle the button click event and update the chart’s data:
javascript // script.js $(document).ready(function () { var ctx = document.getElementById('bar-chart').getContext('2d'); // Initial data var initialData = { labels: ['January', 'February', 'March', 'April', 'May'], datasets: [{ label: 'Monthly Sales', data: [65, 59, 80, 81, 56], backgroundColor: 'rgba(75, 192, 192, 0.2)', borderColor: 'rgba(75, 192, 192, 1)', borderWidth: 1, }] }; var myChart = new Chart(ctx, { type: 'bar', data: initialData, }); // Update the chart data on button click $('#update-chart').click(function () { // New data values var newData = [45, 75, 60, 92, 48]; // Update the chart's data myChart.data.datasets[0].data = newData; // Update the chart myChart.update(); }); });
In this code, we first define the initial chart data and create the chart as before. Then, we use jQuery to listen for the button click event and update the chart’s data when the button is clicked. This dynamic behavior enhances the interactivity of our dashboard.
7. Adding More Charts
To create a comprehensive interactive dashboard, you can add more charts and elements as needed. Simply follow the same pattern for each chart you want to include. Here’s an example of adding a pie chart:
html <div class="chart-container"> <canvas id="pie-chart"></canvas> </div> javascript Copy code // script.js $(document).ready(function () { // ... // Create a pie chart var pieCtx = document.getElementById('pie-chart').getContext('2d'); var pieData = { labels: ['Red', 'Blue', 'Yellow'], datasets: [{ data: [30, 45, 25], backgroundColor: ['red', 'blue', 'yellow'], }] }; var pieChart = new Chart(pieCtx, { type: 'pie', data: pieData, }); });
This code follows the same structure as the previous chart creation. You can add as many charts and interactive elements as needed to build a comprehensive dashboard that suits your data visualization needs.
Conclusion
In this tutorial, we’ve explored how to create interactive dashboards using jQuery and Chart.js. These powerful tools make it easy to build dynamic and engaging web-based data visualizations. By following the steps outlined here, you can create your own custom dashboards, adding charts, interactivity, and style to present your data effectively.
Remember that the key to building compelling dashboards is not just the technical implementation but also thoughtful design and user experience considerations. As you continue to explore and expand your skills, you’ll be able to create even more sophisticated and user-friendly interactive dashboards for various applications.
So, go ahead, start coding, and unleash the potential of data visualization in your web projects! Happy coding!
Table of Contents
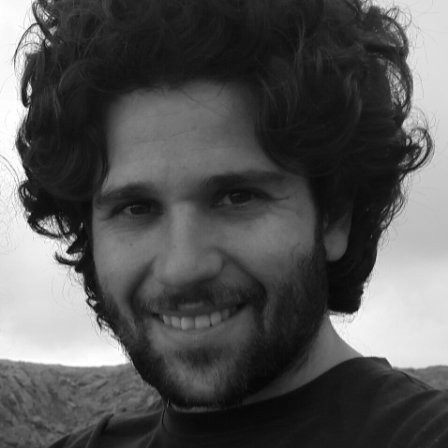
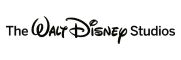