Building Responsive Tabs with jQuery UI
In the world of web development, creating interactive and responsive user interfaces is crucial for delivering exceptional user experiences. One common element you’ll frequently encounter is tabbed navigation, which allows users to switch between different sections of content without navigating to separate pages. jQuery UI, a powerful JavaScript library, provides a convenient way to build responsive tabs effortlessly. In this guide, we will explore how to create dynamic and adaptable tabbed navigation using jQuery UI, complete with code samples and step-by-step explanations.
Table of Contents
1. Understanding the Power of jQuery UI
jQuery UI is an extension of the popular jQuery library, designed to simplify the process of creating interactive and visually appealing user interfaces. It provides a variety of widgets, including tabs, sliders, dialog boxes, and more, that can be easily customized and integrated into your web projects. One of the standout features of jQuery UI is its responsiveness, allowing your UI components to adapt seamlessly to different screen sizes, making it ideal for creating tabbed navigation that works well across devices.
2. Setting Up the Environment
Before diving into the code, ensure that you have jQuery UI included in your project. You can either download the library or use a Content Delivery Network (CDN) to include it directly in your HTML file.
html <!DOCTYPE html> <html> <head> <title>Responsive Tabs with jQuery UI</title> <link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script> </head> <body> <!-- Your tabbed navigation content will go here --> </body> </html>
3. Creating the Tabbed Navigation Structure
Now that you have jQuery UI set up, let’s start building our responsive tabs. We’ll begin by creating the basic HTML structure for the tabbed navigation.
html <div id="tabs"> <ul> <li><a href="#tabs-1">Tab 1</a></li> <li><a href="#tabs-2">Tab 2</a></li> <li><a href="#tabs-3">Tab 3</a></li> </ul> <div id="tabs-1"> <!-- Content for Tab 1 --> </div> <div id="tabs-2"> <!-- Content for Tab 2 --> </div> <div id="tabs-3"> <!-- Content for Tab 3 --> </div> </div>
In this example, we’ve created a tabbed navigation with three tabs. The ul element contains the tab headers, and each div element represents the content of a tab.
4. Initializing the Tabs
To transform the HTML structure into functional tabs, you need to initialize the tabs using jQuery UI. Add the following JavaScript code after including the jQuery UI library:
javascript $(function() { $("#tabs").tabs(); });
This code snippet targets the #tabs element and converts it into a tabbed navigation interface. The tabs() function handles the initialization and behavior of the tabs, making it easier for you to manage the user interaction.
5. Customizing the Tabbed Navigation
While the default behavior of jQuery UI tabs is useful, you’ll often want to customize the appearance and behavior to match your project’s design. Here are some common customizations you can apply:
5.1. Styling the Tabs
You can customize the visual style of the tabs using CSS. jQuery UI provides various classes that you can target to modify the appearance. For example, to change the background color of the active tab, you can use the .ui-tabs-active class:
css .ui-tabs-active { background-color: #3498db; color: white; }
5.2. Adding Icons to Tabs
Icons can enhance the user experience and provide visual cues for each tab’s content. To add icons to your tabs, include an i element within the a element of each tab header:
html <li><a href="#tabs-1"><i class="fa fa-home"></i> Home</a></li> <li><a href="#tabs-2"><i class="fa fa-info-circle"></i> About</a></li> <li><a href="#tabs-3"><i class="fa fa-envelope"></i> Contact</a></li>
In this example, we’ve used Font Awesome icons to illustrate the concept. Replace the classes and icons with your preferred icon library.
6. Implementing Responsive Behavior
Responsive design is crucial for providing a consistent experience across various devices. jQuery UI tabs inherently adapt to different screen sizes, but you can take additional steps to ensure a seamless experience:
6.1. Enabling Scrollable Tabs
When dealing with a large number of tabs, it’s a good idea to make them scrollable on smaller screens. To achieve this, you can use the scrollable option when initializing the tabs:
javascript $(function() { $("#tabs").tabs({ scrollable: true }); });
This option adds navigation arrows to the tab headers, allowing users to scroll through tabs that don’t fit within the available space.
6.2. Hiding Tabs on Small Screens
For an even more streamlined experience on small screens, you can choose to hide certain tabs and provide a dropdown menu for navigation. Here’s how you can implement this:
javascript $(function() { $("#tabs").tabs({ responsive: true, activate: function(event, ui) { if ($(window).width() <= 768) { // Hide other tabs and show a dropdown menu } } }); });
In this example, when a tab is activated and the screen width is 768 pixels or less, you can write code to hide the other tabs and display a dropdown menu for navigation.
Conclusion
jQuery UI provides an efficient and user-friendly way to create responsive tabs that enhance the user experience of your web applications. By following the steps outlined in this guide, you can build interactive and visually appealing tabbed navigation that adapts seamlessly to various screen sizes. Whether you’re designing a portfolio website, an e-commerce platform, or a blog, mastering the art of responsive tabs using jQuery UI will undoubtedly elevate your development skills and contribute to delivering outstanding user experiences.
Remember, while jQuery UI is a powerful tool, staying updated with the latest web development trends and technologies will help you create even more compelling and user-centered interfaces. With this newfound knowledge, you’re well-equipped to embark on your journey to crafting exceptional user interfaces that engage and delight users across the digital landscape.
Table of Contents
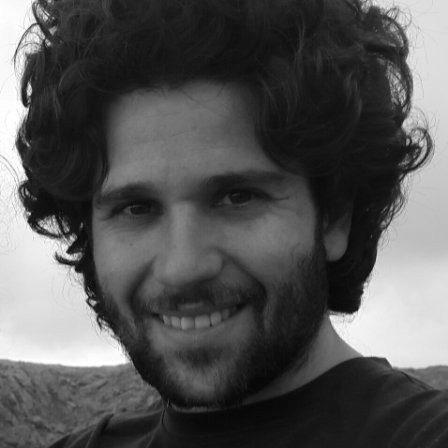
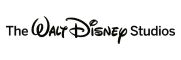